What is Content Security Policy (CSP)?
Content Security Policy (CSP) is a security mechanism designed to mitigate cross-site scripting (XSS) and other code injection attacks.
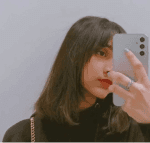
Medusa
8 mins
What is Content-Security-Policy?
Content Security Policy (CSP) is a security mechanism designed to mitigate cross-site scripting (XSS) and other code injection attacks by allowing web developers to specify which sources of content are considered legitimate for a particular web page or application by allowing web developers to specify which sources types of content that can be loaded and executed on a web page.
Why should developers care about CSP?
Here are several reasons why developers should prioritize CSP implementation:
1. Mitigating Attacks:
CSP functions as a digital guard, particularly against cross-site scripting (XSS) attacks. XSS is a type of cyber threat where attackers inject malicious scripts into web pages.
CSP prevents these injected scripts from running by allowing only authorized sources of content to execute. This protective layer greatly reduces the risk of such attacks.
2. Client-Side Code Injection Defense:
Client-side injection attacks involve introducing harmful code into an application's for example XSS, leading to potential breaches and vulnerabilities.
CSP acts like a gatekeeper, permitting only trusted content sources and blocking unauthorized code injections that could compromise the application's stability and security.
3. Guarding APIs:
APIs often interact with client-side code, which can become an avenue for attackers to target the API itself.
CSP, by securing the client side, indirectly safeguards APIs from being exploited by malicious actors through vulnerabilities on the user's side.
4. Reduced Attack Surface:
An application's attack surface is essentially the number of ways an attacker can breach it. CSP trims this surface by defining which sources of content are permissible.
This controlled approach means fewer opportunities for attackers to find weak points and infiltrate the application.

This flowchart illustrates how Content Security Policy (CSP) enables loading JavaScript files from only allowed domains.
How to Implement CSP
Apply the principle of least privilege in CSP by starting with a strict policy that blocks most content sources, gradually whitelisting only trusted sources and behaviors necessary for your application's functionality. Regularly monitor violation reports and adjust the policy iteratively to maintain security without breaking the application.
Implementing Content Security Policy (CSP) involves configuring the appropriate directives in your web application's HTTP headers or within the HTML meta tag. Here's a step-by-step guide on how to implement CSP:
1. Understand Your Application:
Determine the types of content sources your application should trust and the ones it should block. This involves analyzing which domains host your scripts, styles, images, fonts, and other resources.
Choose Directives:
Decide which CSP directives to include based on your application's needs. Common directives include
default-src
,script-src
,style-src
,img-src
,connect-src
,frame-src
, and more.
Balancing Security and Functionality in CSP:
Restricting content to your domain only can inadvertently break numerous websites that rely on external resources, such as scripts from CDNs like jQuery. Consider allowing specific trusted sources, like CDNs, to ensure the proper functioning of third-party content while maintaining a strong security posture. Regular testing and fine-tuning of the policy are crucial to avoid unintended disruptions.
Update HTTP Headers:
If you're using a server-side language like PHP, Python, or Node.js, you can set the CSP policy by adding the
Content-Security-Policy
header to the server's HTTP response. For example, in PHP:
header("Content-Security-Policy: default-src 'self'");
HTML Meta Tag (Optional):
Alternatively, you can specify the CSP policy directly in your HTML file's
<meta>
tag within the<head>
section:
<meta http-equiv="Content-Security-Policy" content="default-src 'self'">
Gradual Policy Refinement:
As you monitor your application's behavior with the initial policy, gradually expand it to allow additional trusted content sources.
Test your application thoroughly after each modification to ensure nothing breaks.
Testing and Debugging:
Browsers often have built-in developer tools that can help identify CSP violations. Check the browser console for error messages related to CSP violations.
Utilize tools like the "CSP Evaluator" to validate your CSP policy for potential errors.
Implementing Nonces and Hashes (Optional):
For more granular control, you can use nonces or hashes in your CSP directives. Nonces are random tokens that match between script tags and CSP policies, while hashes verify inline scripts.
Report-Only Mode (Optional):
During initial implementation, you can set the CSP policy to "report-only" mode. This mode doesn't enforce the policy but reports violations, allowing you to fine-tune the policy before applying it.
Test on Different Browsers:
Different browsers may interpret CSP directives slightly differently. Test your application on various browsers to ensure consistent behavior.
Document Your Policy:
Clearly document your CSP policy, detailing the directives and sources you've allowed. This documentation helps other developers understand and maintain the policy.
Regular Review and Maintenance:
Security threats and application requirements evolve over time. Regularly review and adjust your CSP policy to accommodate these changes.
Remember that the goal of implementing CSP is to strike a balance between security and functionality. It might take some trial and error to find the right policy for your application. Gradually refining your policy ensures that you achieve the desired security while avoiding unintended disruptions to your application's behavior.
Test your APIs for vulnerabilities by deploying Akto from here and try yourself.
Practical Demonstration
Have a look at this PHP code
This code sets the Content Security Policy header to allow content only from the same domain and prevents the loading of any other files such as .js.CSP

CSP blocking script execution
From the image above, it is evident that the JavaScript code is not functioning due to the Content Security Policy (CSP). However, removing the CSP from the file results in an XSS pop-up.
In a real-life scenario, an attacker can inject a script that steals a user's session cookies and sends them to the attacker's domain. This can even lead to account takeover.

No CSP leads to malicious JS script execution
CSP Directives and Use Cases
Some Content Security Policy (CSP) directives along with their corresponding use cases:
'default-src': Sets Default Behavior
Use Case: This directive establishes the default behavior for other directives that aren't explicitly defined. It's ideal for enforcing a strong security baseline for all content types.
Example:
default-src 'self' <https://trusted-domain.com>;
'script-src': Controlling Script Execution
Use Case: This directive manages the sources from which scripts can be executed on a webpage. It's vital for preventing cross-site scripting (XSS) attacks.
Example:
script-src 'self' <https://trusted-scripts.com>;
'style-src': Managing Stylesheets
Use Case: This directive specifies where stylesheets can be loaded from. It prevents unauthorized styles from affecting your content's appearance.
Example:
style-src 'self' <https://trusted-styles.com>;
'img-src': Specifying Image Sources
Use Case: This directive defines the permitted sources for loading images. It guards against loading images from untrusted origins that might contain malicious content.
Example:
img-src 'self' <https://trusted-images.com>;
'connect-src': Controlling Network Requests
Use Case: This directive restricts the domains your application can make network requests to. It's crucial for thwarting unauthorized data leaks.
Example:
connect-src 'self' <https://api.example.com>;
'frame-src': Managing Frame Sources
Use Case: This directive governs the allowed sources for frames or iframes embedded in your page. It's essential for defending against clickjacking attacks.
Example:
frame-src 'self' <https://trusted-frames.com>;
By employing these directives based on your application's requirements, you can effectively manage the origins from which content is loaded, minimizing security risks and ensuring a safer user experience.
Example Code to implement CSP
Code examples to implement Content Security Policy (CSP):
Example 1: Using the Content-Security-Policy
HTTP Header in PHP
In this example, we're setting a more detailed policy that restricts content sources for different directives, including script-src
and style-src
.
Example 2: Using a Meta Tag in HTML
In this example, the CSP policy is defined directly within the HTML file's <meta>
tag. This approach can be useful when you don't have server-side control over headers, or for scenarios where you want to set specific policies for individual pages.
Both examples demonstrate how to define a CSP policy that restricts content sources for various directives. Adjust the allowed domains and directives to match your application's needs while maintaining a balance between security and functionality.
When to Use CSP?
The advice is simple: "Always use CSP." It's like having a shield to guard your site from potential problems. Here are some situations when you should use CSP:
Dynamic and User-Generated Content:
If your application involves user-generated content, comments, messages, or any form of dynamic content, CSP can help mitigate the risk of cross-site scripting (XSS) attacks by controlling what scripts can be executed on the page.
Web Applications with Forms:
Applications that utilize forms, especially login or registration forms, are susceptible to code injection attacks. Implementing CSP can help safeguard against such attacks by limiting script execution.
E-Commerce and Payment Processing:
E-commerce websites and platforms handling sensitive user data, including payment information, should prioritize CSP to prevent data theft and unauthorized code execution.
Single-Page Applications (SPAs):
SPAs often involve loading content dynamically. CSP can help maintain security as new content is fetched and rendered, preventing malicious scripts from running.
Websites with Advertising:
Third-party advertisements can sometimes introduce security risks. CSP helps mitigate potential threats posed by ad networks by restricting what they can do on the page.
Government and Regulatory Compliance:
Some industries, such as government and healthcare, have stringent security regulations. Implementing CSP can be part of your compliance strategy to ensure data protection.
Protecting User Privacy:
Applications that handle user data should prioritize CSP to prevent data leaks and unauthorized access to sensitive information.
Web Applications with User Sessions:
If your application uses user sessions or maintains sensitive data within a session, CSP can help prevent session hijacking and unauthorized access.
Applications with Sensitive Logic:
Specific applications with sensitive business logic, such as voting systems, should implement CSP to prevent tampering and ensure the integrity of processes.
When not to use Content Security Policy?
While Content Security Policy (CSP) is a powerful security mechanism, there are situations where using CSP might not be necessary or might introduce complications. Here are some scenarios when you might consider not using CSP:
Static Websites:
If your website is purely static and doesn't involve user interactions or dynamic content, implementing CSP might be unnecessary. CSP is most effective when there's potential for dynamic code execution.
Limited Functionality Websites:
Simple websites with minimal functionality, such as basic informational pages, might not benefit significantly from CSP. The overhead of setting up and maintaining CSP might outweigh the security benefits in such cases.
Very Controlled Environments:
In highly controlled and isolated environments where the application's content sources are well-defined and under strict supervision, CSP might provide minimal additional security benefits.
Applications with Existing Strong Security Measures:
If your application already employs robust security practices, such as strong input validation and output encoding, the urgency to implement CSP might be lower. CSP should be considered as part of a comprehensive security strategy.
Compatibility Issues:
Some legacy browsers might not fully support CSP or might interpret CSP directives differently. If your target audience includes users on older browsers, you might encounter compatibility issues.
Third-Party Scripts and Widgets:
If your application heavily relies on third-party scripts, plugins, or widgets that require inline scripts or are not compatible with strict CSP policies, implementing CSP might disrupt functionality.
Performance Concerns:
Implementing and enforcing CSP policies can add overhead to the application's loading and rendering process. In performance-critical applications, the impact of CSP on load times should be considered.
Rapid Prototyping and Testing:
During rapid prototyping or testing phases, CSP might add complexity and hinder the agility needed for quick iterations. It could be better to focus on functionality before tightening security measures.
Limited Resources:
For smaller projects with limited development resources, spending significant time implementing and fine-tuning CSP might not be feasible compared to other development priorities.
Temporary Solutions:
If you're planning a short-term project or a temporary website, the effort required to implement CSP might not be justified.
In essence, CSP should be evaluated on a case-by-case basis, considering the specific needs of the application, the potential security risks, and the trade-offs between security and other factors like performance and functionality. While CSP is a valuable security tool, there are scenarios where it might not be the most suitable choice.
Conclusion
In conclusion, Content Security Policy (CSP) is a valuable security mechanism for web developers to implement in order to protect against various types of attacks and ensure the safety of user data. By carefully analyzing the types of content sources that an application should trust and blocking those that are not authorized, CSP can greatly enhance the security of web applications. Additionally, CSP can help developers comply with security standards and best practices, showcase their dedication to robust security, and create a safe browsing experience for their users. By following the step-by-step guide outlined in this document and considering when and when not to use CSP, developers can effectively implement CSP and strike a balance between security and functionality.
Keep reading
API Security
3 minutes
Twilio Data Breach: 33 Million Authy User Phone Numbers Exposed by Hacker
Twilio's data breach exposed 33 million Authy user phone numbers because of an unauthenticated endpoint.
API Security
10 minutes
Dynamic White Box Testing Guide - Key Features, Levels and Examples
Dynamic White Box Testing is a strategy in which the tester is aware of the internal structure of the application under test.
API Security
8 minutes
DAST Black Box Testing: Types of Black Box Testing and How it works
Black Box Testing is a methodology where the internal workings of the system under test are unknown to the tester.