Server-Side Request Forgery: Proactive SSRF Prevention Tactics for Developers
In this blog, you will learn how to prevent Server-Side Request Forgery (SSRF) as a developer.
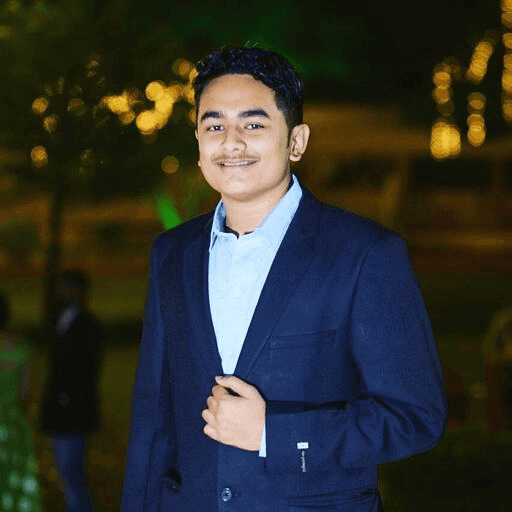
Jaydev Ahire
Feb 25, 2023
Introduction SSRF:
Server-Side Request Forgery (SSRF) is a type of web application vulnerability that allows an attacker to send crafted requests from a vulnerable server to an arbitrary destination. This can allow the attacker to access sensitive information, such as internal network resources, by making requests on behalf of the vulnerable server. SSRF attacks can also be used to launch further attacks, such as port scanning and denial of service attacks.
What is SSRF?
In a Server-Side Request Forgery (SSRF) attack, an attacker manipulates the functionality of a server to gain access or modify resources. An attacker manipulates a server into accessing resources that it was not intended to access. This is done by altering a URL that the server uses to retrieve data, such as an image or file upload link, and redirecting the server to a malicious URL. When the manipulated request is sent to the server, the server-side code attempts to read data from the altered URL, potentially allowing the attacker to access information that is not intended to be publicly available.
To safeguard against SSRF attacks, it is crucial for developers to implement best practices and security measures in their applications and API endpoints.
Attacks scenarios and preventions:
1. Exploiting Internal Resources (Localhost) via SSRF: Accessing and reading files from the server through the internal network by targeting the administrator panel (/admin), which is only accessible within the internal network, is an example of exploiting internal resources via SSRF.
Example: https://www. example. com/index?ImageUrl=http://127.0.0.1/admin
Example of how SSRF vulnerability could be introduced and mitigated in a more complex web application using a PHP framework such as Laravel:

This controller takes a user-supplied $url parameter, reads the contents of the URL using file_get_contents(), and returns it as a download to the user. An attacker could exploit this vulnerability by crafting a URL that points to a file on the server's file system, such as "file:/// etc/passwd", and passing it as a parameter in the request. This would allow the attacker to read sensitive files on the server.
To mitigate this vulnerability, Developers can use Laravel's built-in validation and sanitization functions to ensure that only safe URLs are accepted:

Here we use the validate() method of the Request object to validate that the url parameter is present and is a valid URL. This will prevent an attacker from passing in a malicious file URL. This will not prevent an attacker from putting in URLs like 127.0.0.1/admin
or http://169.254.169.254/latest/meta-data/
, to prevent this you could use a whitelist of URLs only to allow specific URLs to be passed to the controller. This will prevent the attacker from passing any malicious URLs.
2. Exploiting Cloud Metadata (AWS): Accessing metadata on Amazon Web Services (AWS), SSRF can be used to obtain sensitive information about the AWS environment, such as the internal IP addresses of the servers, the IAM role associated with the instance, and the metadata of the IAM role. Check out these AWS metadata tests by Akto.
sensitive-aws-details-exposed-via-replacing-url-param-with-encoded-url-due-to-ssrf
sensitive-aws-details-exposed-via-replacing-csv-param-due-to-ssrf
sensitive-aws-details-exposed-via-replacing-image-param-due-to-ssrf
sensitive-aws-details-exposed-via-replacing-file-param-due-to-ssrf
sensitive-aws-details-exposed-via-replacing-xml-param-due-to-ssrf
sensitive-aws-details-exposed-via-replace-pdf-param-due-to-ssrf
An example of an SSRF vulnerability in the context of AWS metadata access is an API endpoint that allows users to upload images but does not properly validate the file type. An attacker could upload a specially crafted file that includes a request to the AWS metadata endpoint (http://169.254.169.254/latest/meta-data/
) as the file content. The server-side script that processes the image upload would then send this request to the AWS metadata endpoint, and the attacker could obtain sensitive information about the environment.
Here is an example of a Python script that demonstrates this vulnerability:

In this example, the attacker creates a file called "ssrf.jpg" with the content "GET http://169.254.169.254/latest/meta-data/
" and then uploads this file to the web application using the upload_image() function. The server-side script that processes the image upload would then request the AWS metadata endpoint, and the attacker could obtain sensitive information about the environment.
It's important to note that this is a simplified example, and in real-world scenarios, the validation of the file and the request will be more complex.
Here is a python script that demonstrates how to prevent this type of SSRF vulnerability:

In this example, the upload_image() function has been modified to check the file content against a whitelist of allowed domains before sending the request. The is_valid_url() function is used to parse the URL and check the domain against the whitelist. If the domain is not in the whitelist, the function returns False, and the upload is rejected. This prevents an attacker from uploading a file containing a request to an unauthorized domain, such as the AWS metadata endpoint.
It's important to note that this is a simplified example, and in real-world scenarios, the validation of the file and the request will be more complex.
It's also important to mention that AWS has mitigated this issue by blocking requests from external IP addresses to the metadata service on IP 169.254.169.254. So, even if an attacker managed to exploit an SSRF vulnerability, the response would be a 403 error. It is important to have security best practices and security controls in place to prevent this kind of attack.
Test it out with Akto using Akto's SSRF test library!
SSRF Prevention Techniques for Developers:
1. Whitelisting: Only allow requests to specific, known domains, API endpoints or IP addresses. Whitelisting is a security measure that allows only specific, known domains or IP addresses to be accessed by a server-side program. This helps to prevent an attacker from making requests to unauthorized domains through a Server-Side Request Forgery (SSRF) vulnerability.
2. Restrict request protocols: Control request protocols on APIs by blocking requests made using uncommon protocols such as ftp:/, gopher:/, and file:/. Requests to servers are usually sent over HTTPS or HTTP, but it's important to block requests using FTP and GOPHER because they are not commonly used. By blocking the FILE protocol, attackers cannot access sensitive files.
To prevent SSRF attacks by restricting request protocols, add a check in the code that verifies the protocol used in the request URL. If the protocol is not one of the allowed protocols (e.g. "https:" or "http:"), the request is blocked, and an error message is returned.
Here is an example code snippet in PHP that demonstrates this approach:

In this example, the script checks the protocol of the URL passed in the request using the parse_url function and compares it to the protocols in the whitelist. If the protocol is not in the whitelist, the request is blocked, and an error message is returned. If the protocol is in the whitelist, the request is processed as usual.
3. Implement a robust allowlist: Validate IP addresses and DNS names using an allowlist to ensure your application only accesses authorized resources. This helps to prevent attackers from making unauthorized requests to unintended resources. The SSRF prevention technique of implementing a strong allowlist involves using a whitelist of IP addresses and DNS names that the application is allowed to access. This helps prevent SSRF attacks by ensuring that the application can only make requests to trusted resources.
For example, consider a web application that needs to retrieve information from an API at api.example.com. An attacker could try to exploit an SSRF vulnerability in the application to retrieve sensitive information from a different API at api.evil.com.
Here is an example of a Python script that implements an allowlist:

In this example, the retrieve_url()
function has been modified to check the URL's host against an allowlist of allowed hosts before sending the request. The is_valid_host() function extracts the host from the URL and checks it against the allowlist.
If the host is not in the allowlist, the function returns False, and the request is rejected. This helps prevent attackers from exploiting an SSRF vulnerability to request unintended resources.
4. Implement the cloud built-in protection mechanism: AWS has implemented a new version of the Instance Metadata Service (IMDSv2) to address the potential risk of SSRF attacks. This new version requires an additional token to be obtained through a separate API call and allows administrators to set a maximum number of network hops for IMDS access.
These measures enhance the security of IMDS and can be enforced as an organizational policy, ensuring that all instances within the organization are protected.
It's important to keep in mind that the attack scenarios and code snippets presented above are simplified illustrations for developers to understand the preventions of SSRF vulnerability. The validation/prevention process may be more complex in real-world scenarios.
Here is a list of all SSRF tests in Akto. We are adding more tests every week to make SSRF testing comprehensive:
sensitive-aws-details-exposed-via-replacing-url-param-with-encoded-url-due-to-ssrf
sensitive-aws-details-exposed-via-replacing-csv-param-due-to-ssrf
sensitive-aws-details-exposed-via-replacing-image-param-due-to-ssrf
sensitive-aws-details-exposed-via-replacing-file-param-due-to-ssrf
sensitive-aws-details-exposed-via-replacing-xml-param-due-to-ssrf
sensitive-aws-details-exposed-via-replace-pdf-param-due-to-ssrf
sensitive-localhost-details-exposed-via-replacing-uri-param-with-localhost-admin-due-to-ssrf
sensitive-localhost-details-exposed-via-replacing-uri-param-to-encoded-localhost-admin-due-to-ssrf
If you want us to add a case you don't see above, write to us at support@akto.io
Important Links
Keep reading
API Security
8 minutes
NIST Cybersecurity Framework
The NIST Cybersecurity framework provides organizations with a set of standards, guidelines, and practices to develop strong cybersecurity practices for managing cybersecurity risks effectively.
API Security
7 minutes
API Security Audit
An API Security Audit evaluates APIs, identifies potential risks, and strengthens the organization's defenses against security breaches and cyber-attacks.
API Security
8 minutes
Security Information and Event Management (SIEM)
SIEM aggregates and analyzes security data across an organization to detect, monitor, and respond to potential threats in real time.
Experience enterprise-grade API Security solution