What is Authentication?
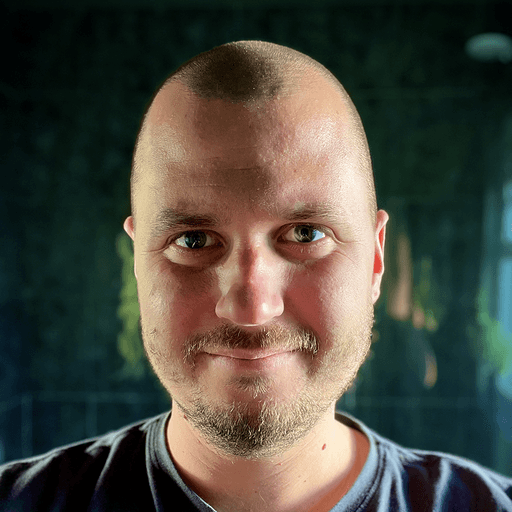
Luke Stephens
Mar 7, 2024
What is API Authentication?
API Authentication is the process used to verify the identity of a client or user trying to access an API. It involves confirming that the client is who they claim to be, typically through credentials like usernames and passwords, tokens (like JWTs), or API keys.
Why is API Authentication Necessary?
API Authentication is essential for various reasons:
Resource Protection: It ensures that only authorized users can access, modify, or delete data.
Rate Limiting: Authentication helps in tracking and controlling the rate at which users can make requests to your API, ensuring fair usage and preventing abuse.
Audit Trails: By authenticating users, you can maintain a log of who accessed what resources and when, which is crucial for auditing and regulatory compliance.
User Experience: Provides personalized user experiences by identifying the user and serving tailored content.
Legal and Regulatory Compliance: Ensures compliance with various data protection laws and regulations by controlling access to sensitive data.
Basic Authentication
Basic Authentication is a simple authentication scheme built into the HTTP protocol. The client sends a HTTP request with an Authorization
header containing the word Basic
followed by a space and a base64-encoded username:password
string.
Basic Authentication Example:
A client wants to access a resource at https://api.example.com/resource
. It must include an Authorization
header with its request:
In the above example, QWxhZGRpbjpvcGVuIHNlc2FtZQ==
is the base64 encoding of username:password
.
Implementation:
Server-Side:
Decode the base64 string, extract the username and password.
Verify the credentials against your user database or authentication provider.
If the credentials are valid, process the request.
If not, return a
401 Unauthorized
response.
Client-Side:
Collect username and password from the user.
Create a base64 encoded string of
username:password
.Include this string in the
Authorization
header in subsequent HTTP requests.
API Key
API keys are unique strings associated with an individual or application. They act as both identifiers and access tokens. However, API keys only identify the client, not the user, and are simple to implement.
API Key Example:
A client wants to access a resource at https://api.example.com/resource
. It must include an API-Key
header with its request:
Implementation:
Server-Side:
Extract the API key from the
API-Key
header.Verify the API key against your database or authentication provider.
If the API key is valid, process the request.
If not, return a
401 Unauthorized
response.
Client-Side:
Include the API key in the
API-Key
header in HTTP requests.
Bearer Token
Bearer Tokens are given to the user after a successful authentication. They are called bearer tokens because any bearer of the token can use it to access the API, without further proof of identity.
Bearer Token Example:
A client wants to access a resource at https://api.example.com/resource
. It must include an Authorization
header with its request:
Implementation:
Server-Side:
Extract the token from the
Authorization
header.Verify the token's signature and check its expiration date.
If the token is valid, process the request.
If not, return a
401 Unauthorized
response.
Client-Side:
Include the bearer token in the
Authorization
header in HTTP requests.
OAuth 2.0
OAuth 2.0 is a protocol that allows a user to grant a third-party website or application access to their resources, without sharing their credentials. It's widely used for token-based authentication.
OAuth 2.0 Example:
A client wants to access a user's data on https://api.example.com/resource
. It directs the user to an OAuth 2.0 authorization server, the user authenticates, and grants the necessary permissions. The client receives an access token which it uses to access the user's data:
Implementation:
Server-Side:
Extract the token from the
Authorization
header.Verify the token with the OAuth 2.0 authorization server.
If the token is valid, process the request.
If not, return a
401 Unauthorized
response.
Client-Side:
Direct the user to the OAuth 2.0 authorization server for authentication.
Obtain user consent for the required permissions.
Exchange the authorization code for an access token.
Include the access token in the
Authorization
header in HTTP requests.
Refresh Tokens (a part of OAuth 2.0)
Refresh tokens are used to obtain new access tokens without requiring the user to re-authenticate. They are particularly useful in scenarios where access tokens have short expiration times.
Refresh Tokens Example:
After the access token expires, the client can use a refresh token to obtain a new access token:
Implementation:
Server-Side:
Verify the refresh token.
If the refresh token is valid, issue a new access token and optionally, a new refresh token.
If not, return a
401 Unauthorized
response.
Client-Side:
Store the refresh token securely.
Use the refresh token to request new access tokens once the current access token expires.
JWT (JSON Web Tokens)
JWT is a compact, URL-safe means of representing claims to be transferred between two parties. The claims in a JWT are encoded as a JSON object that is used as the payload of a JSON Web Signature (JWS) structure or as the plaintext of a JSON Web Encryption (JWE) structure, enabling the claims to be digitally signed or integrity protected with a Message Authentication Code (MAC) and/or encrypted.
JWT Example:
A client wants to access a resource at https://api.example.com/resource
. It includes a signed JWT in the Authorization
header of its request:
Implementation:
Server-Side:
Extract the JWT from the
Authorization
header.Verify the signature of the JWT and check the claims contained within the JWT.
If the JWT is valid, process the request.
If not, return a
401 Unauthorized
response.
Client-Side:
Obtain a signed JWT from the authorization server.
Include the JWT in the
Authorization
header in HTTP requests.
Different types of Authentication

Conclusion
This module has provided a foundation in API authentication methods, enabling you to make informed decisions in securing your API.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.