Authorization
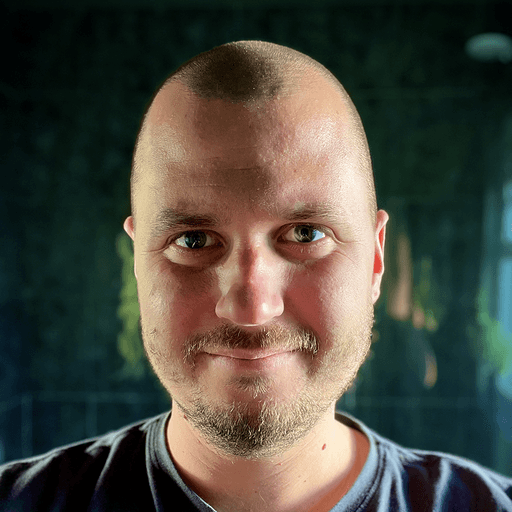
Luke Stephens
Mar 19, 2024
What is API Authorization?
API Authorization is the process of determining whether a client has permission to perform a specific action or access particular data in an API. It's about verifying that once a user or application is authenticated (proven their identity), they are allowed to do what they are trying to do.
Why is API Authorization Necessary?
Resource Protection: Ensures that only permitted entities, be it users or systems, can access or modify critical resources, protecting them from potential threats.
Fine-grained Access Control: It isn't just about granting access; it's about defining levels and specifics. This feature enables precise control over who can do what, and sometimes even when or from where within the system.
Audit Trail: A robust API Authorization system allows for comprehensive logging and monitoring of actions. This is invaluable not just for diagnostics but also for compliance and security audits.
System Integrity: A well-authorized system is less prone to errors and misuses. It ensures the system operates as intended by preventing any unauthorized or unintended operations.
Legal and Regulatory Compliance: API Authorization aids in adhering to various data protection laws and regulations by controlling who can access what, ensuring businesses remain compliant.
Role Based Access Control (RBAC)
RBAC simplifies access management by tying access permissions to roles, not individuals. This means when a user is assigned a certain role, they automatically inherit the permissions associated with it, streamlining administration.
Example of RBAC:
In a sophisticated document management system, roles might be as broad as Admin
, Editor
, and Viewer
or as niche as Document Approver
or Metadata Editor
. While Admins have a bird's eye view and control, Editors might have specific rights to create and modify documents, and Viewers would be limited to just reading.
Implementation:
Attribute-Based Access Control (ABAC)
ABAC takes a more dynamic approach, moving beyond roles. It bases access control decisions on multiple attributes associated with the user, the resource they're trying to access, the environment, and the specific action they're attempting.
Example of ABAC:
Beyond just roles, imagine a system where users are allowed to edit documents only if they are the author, the document isn’t finalized, and they're trying to access it during office hours.
Implementation:
OAuth Scopes
OAuth scopes provide a way to limit the access granted to an access token.
Example:
During login, or when granting access, an application can request specific permissions through scopes, like read:documents
or write:documents
. The API then verifies these scopes on subsequent requests, ensuring proper authorization.
Implementation:
Claims Based Access Control (CBAC)
CBAC focuses on claims or assertions about a user to make authorization decisions. It doesn't rely on predefined roles or attributes but leverages information or claims about a user.
Example of CBAC:
Information or claims like isManager
or department: HR
could be used to determine a user’s access to certain resources, allowing for a more individualized access control.
Implementation:
Different types of Authorization:

Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.