What is API?
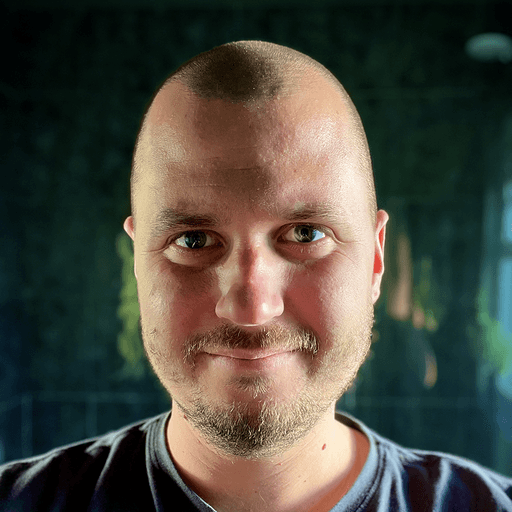
What is an API?
APIs are mechanisms that enable two software components to communicate with each other using a set of definitions and protocols. They facilitate communication between different software applications, allowing them to request and exchange data seamlessly, just like placing an order in a restaurant. For instance, when you click on the "See profile" button on Facebook, an API called api/fetchprofiledetails
talks to the database, fetches profile data and shows you the profile on your mobile phone.
What does API stand for?
API stands for "Application Programming Interface". At its core, an API mean serves as a bridge that facilitates seamless communication and data sharing among different software applications. Think of it as a universal translator that ensures smooth interaction between various software components, regardless of their programming languages or underlying technologies.
How do APIs work?
At its core, an API (Application Programming Interface) acts as a messenger or intermediary between two software applications, allowing them to communicate and share information with each other. It simplifies interactions and allows developers to access specific features or data from one application to another.
Step 1: Request and Response
Imagine you're in a restaurant, and you want to order a dish. In this analogy:
You: You're like the client or user, making a request.
Waiter: The API acts as the waiter, taking your request and serving you a response.
Kitchen: The server (or the application you're interacting with) is like the kitchen, where the actual work happens.
Step 2: Making a Request
You decide what dish you want (e.g., a burger).
You raise your hand and call the waiter.
You tell the waiter your order (e.g., "I'd like a burger with fries, please").
In API terms:
You decide what data or action you want from a remote application (e.g., weather data).
You create a request to the API by specifying the data you need (e.g., "I'd like weather data for New York, please").
You send this request to the API, typically using HTTP (Hypertext Transfer Protocol) as the communication method.
Step 3: Processing the Request
The waiter takes your order to the kitchen.
The kitchen prepares the burger and fries.
The waiter brings your order back to you.
In API terms:
The API receives your request and understands what you're asking for.
The remote application (the server) processes your request. For example, it fetches the current weather data for New York.
The API then prepares the response, which contains the requested data.
Step 4: Receiving a Response
The waiter brings your burger and fries to your table.
You enjoy your meal.
In API terms:
The API sends the response back to you, typically in a format like JSON (JavaScript Object Notation) or XML (eXtensible Markup Language).
Your application (or code) receives the response and can use the data as needed.
Example: Weather API
Let's apply this concept to a real-world example using a Weather API:
Step 1: Request and Response
You want to display the current weather on your website.
You need weather data for New York City.
You make a request to a Weather API, specifying the location (New York City).
Step 2: Making a Request
Your code sends an HTTP request to the Weather API, saying, "I'd like the current weather data for New York City." (
/weather?location=NewYorkCity
)The API receives your request.
Step 3: Processing the Request
The Weather API processes your request by fetching the latest weather data for New York City from its database or another source.
It prepares a response containing this weather data.
Step 4: Receiving a Response
The Weather API sends the response back to your code in JSON format.
Your website's code receives the JSON response, extracts the weather data, and displays it on your webpage.
This is a simplified example, but it illustrates the fundamental process of how APIs work.
Example of APIs
Social Media Integrations (Facebook API): The Facebook API acts as an interface enabling third-party applications to authenticate users, access user profiles, and facilitate content sharing across platforms. It leverages OAuth 2.0 for secure authorization, allowing applications to interact with the Facebook Graph API to retrieve, post, or delete data on behalf of the user.
E-commerce Transactions (PayPal API): In e-commerce, the PayPal API integrates payment gateway services. It utilizes RESTful APIs for transaction processing, supporting operations like direct payments, refunds, and pre-authorized sales. The API ensures PCI compliance for secure credit card processing and employs encrypted calls for data protection.
Mobile App Data Access (Google Maps API): This API allows mobile applications to fetch geospatial data and integrate mapping services. It provides a suite of HTTP interfaces enabling apps to request map tiles, location data, and routing information. The API supports various data formats including JSON and XML, and allows for the customization of maps with location-based data layers.
Internet of Things (IoT) Connectivity (Philips Hue API): This API facilitates communication between IoT devices, using RESTful endpoints for device control and status updates. It supports JSON payloads for data interchange and employs both local and cloud-based interactions, enabling remote control of smart devices within a Philips Hue ecosystem.
Financial Systems and Fintech (Stripe API): The Stripe API is a cornerstone in fintech for managing online payments, providing robust endpoints for card transactions, billing, and fraud prevention. It adheres to the principles of Representational State Transfer (REST) and utilizes Webhooks for event-driven updates. The API ensures encryption of sensitive data and compliance with financial regulations.
Travel Industry Solutions (Amadeus Travel APIs): These APIs offer comprehensive solutions for the travel industry, interfacing with GDS (Global Distribution Systems). They support REST/JSON interfaces for accessing flight schedules, hotel bookings, and car rental services. The APIs facilitate real-time data synchronization, offering a range of functionalities from price comparison to itinerary planning.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.