REST API
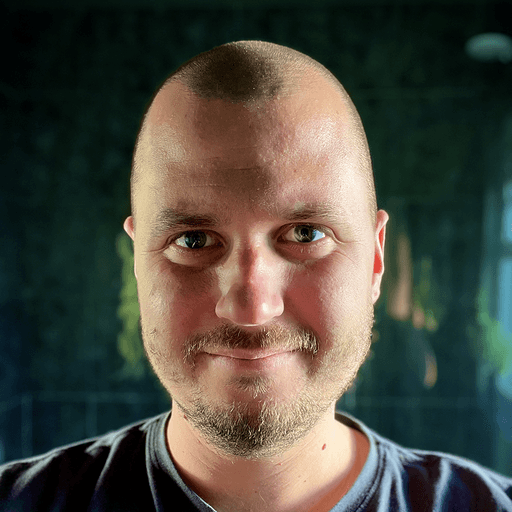
Luke Stephens
Nov 13, 2023
What is a REST API?
Representational State Transfer (REST) is a software architecture that provides guidelines for API design. APIs adhering to REST are termed as REST APIs or RESTful APIs.
At its heart, REST is a set of principles that provide guidelines for designing networked applications. These principles are devised to make applications more scalable and easier to maintain. When APIs adhere to these principles, they are often termed as RESTful APIs.

Origins of REST
It was introduced and defined in 2000 by Roy Fielding in his doctoral dissertation. Fielding was one of the principal authors of the HTTP specification versions 1.0 and 1.1.
In his dissertation, Fielding proposed REST as an architectural approach to designing networked applications. He contrasted it with other prevalent approaches of the time, notably SOAP (Simple Object Access Protocol), highlighting the benefits of a stateless, client-server model that operates over the HTTP standard.
Principles of REST API
Uniform Interface: One of the most lauded aspects of REST, this principle promotes a standard way of interacting with a given API. By providing a consistent interface, REST ensures that regardless of the underlying system, the way you interact remains uniform. For instance, whether you're interacting with an API, managing products in an e-commerce platform or one that handles user data in a social media application, the methods to create, retrieve, update, or delete data remain largely consistent.
For example, when accessing a list of books in a library website, the URL might look something like this:
GET /api/books
. Similarly, when accessing a list of tasks in a to-do list API, the request might beGET /api/tasks
. We’ll dive deeper into how one might perform other tasks such as deleting and editing later.Client-Server Architecture: REST employs a client-server model. The client is responsible for the user interface and user experience, and the server is responsible for storing and retrieving data. This separation of concerns simplifies component implementations, improves scalability, and allows the components to evolve independently.
Statelessness: REST emphasizes that each request from a client to a server should be treated in isolation. This means every request should contain all the information needed for its processing without relying on any stored context on the server. This means that every request to the server should contain:
The full endpoint and HTTP verb, including the resource identifier, e.g.
PUT /api/books/14
Any authentication tokens that are required, e.g.
Authorization: Basic YWxhZGRpbjpvcGVuc2VzYW1
Layered System: In a layered architecture, a client interacts only with the adjacent layer without knowing the intricate details of other layers in the hierarchy. This modularity means you can upgrade, scale, or modify one layer without affecting others, which is crucial for scaling systems over time.
Cacheability: Caching ensures that frequent and recent requests are served faster by storing data from previous requests. In a RESTful system, the server can explicitly labels responses as cacheable or non-cacheable, ensuring timely data delivery while preserving the system's integrity.
Why REST? Benefits and Drawbacks
Benefits:
Simplicity: Uses standard HTTP methods and status codes, making it easy to understand and implement.
Scalability: Its stateless nature means it scales out, allowing for more significant distribution and load-balancing.
Performance: With proper caching, REST can provide fast responses leading to a better user experience.
Language and Platform Agnostic: Can be consumed by any client that understands HTTP and can parse the data format, typically JSON or XML.
Drawbacks:
Statelessness Overhead: Every request must contain all information, leading to larger payloads if not managed properly.
Lack of Real-Time: Traditional REST is request-response, not well-suited for real-time applications.
Multiple Requests: Some operations might require multiple round trips, potentially affecting performance.
How do RESTful APIs Work?
Below you will find a typical lifecycle of a RESTful API operation:
Client Sends a Request
This begins when a user action triggers the client application, such as clicking on a button to view a product list in an e-commerce app. The request might look something like this:
Server Authenticates the Client
Before processing, the server validates the client's identity. It checks tokens or API keys to ensure the client has the right permissions. For instance, banking apps use authentication to ensure only authorized users can check account balances.
In the example above, the server would check the token from the Authorization header, to ensure that it is a valid token and determine which user it belongs to. This authorization header uses Basic
authentication.
Server Processes the Request
Depending on the type of request, the server might retrieve, update, delete, or add data. It communicates with databases, processes data, and prepares a relevant response.
In the example above, the server would update the book with ID 14 to have the title The Wind in the Willows
, and also associate that book with the author of ID 15
.
Server Sends a Response to the Client
The response typically includes data (in formats like JSON or XML) that the client application can interpret and display to the user.
In this instance, the server would most likely respond with a 200 OK
response code and a JSON representation of the book in the response body, for Rest API Example:
Components of a RESTful API Request
Each API request is likely to contain these components:
Unique Resource Identifier (URI): Typically a URL specifying the resource path. For example:
/api/books/14
Method: Common HTTP methods include
GET
(retrieve data),POST
(send data),PUT
(update data), andDELETE
(remove data).HTTP Headers: Metadata about the request. This can include the
User-Agent
, various authorization headers, caching information, and much more!Body: The information that is used to pass additional data to narrow down or customize the request. For example:
{ "title": "Dune", "author": "Frank Herbert" }
Query Parameters: Parameters are the bits after the
?
in a URL. For example inGET /api/books?author=15
, the parameter nameauthor
has been set to15
. This might be used to filter the books that are written by the author with an ID of15
.
RESTful API Authentication Methods
To ensure security, RESTful APIs may use authentication methods such as:
HTTP Authentication:
Basic: Transfers a base64-encoded string that combines the username and password. This is generally considered to be less secure since it can be easily decoded by anyone who can view the request.
Bearer (token-based): More secure than Basic, it provides the client with a token after initial login, which is used for subsequent requests. Bearer tokens are most often combined with JWTs, which are signed tokens that have additional security mechanisms built in.
API Keys: These are unique identifiers given to developers. While they offer easy control, they aren't the most secure as they can be shared or exposed.
OAuth: A robust method, OAuth, especially OAuth 2.0, provides tokens after a more complex initial authentication, ensuring a higher security level. Platforms like Google and Facebook employ OAuth for their APIs. OAuth is used extensively, but is quite complex to implement.
Components of a RESTful API Response
When a server sends back a response, it isn’t just the data; the response also provides meta-information that aids in understanding the context and status:
Status Code: A numeric code indicating the result of the request. Example:
200
means OK/success,404
means the resource wasn't found, and500
indicates a server error.The status line is always the first line in a HTTP response, for example, this curl command returns a 200 response from example.com, the full line reads
HTTP/1.1 200 OK
:
Headers: Headers provide meta-information about the response. Example:
Content-Length
might indicate the size of the response body.Body: It's the main content of the response, often in JSON format. Example: In response to a
GET /books/12
request, the body might be:{ "id": 12, "title": "Dune", "author": "Frank Herbert" }
Status Message: A brief message corresponding to the status code.
Example: For a status code of
200
, the status message is "OK".

Example of a REST API
Understanding the practical application of any concept often requires diving into a tangible, real-world example.
Enter the world of REST APIs via the virtual gateway of a commonly used application: The "To-Do List."
Almost everyone has encountered a "To-Do List" application at some point. These tools are simple yet crucial in helping manage daily tasks and ensuring productivity. From a software perspective, the back end of such an app involves storing, retrieving, updating, and deleting tasks - operations aptly handled by a RESTful API.
API Endpoints in Action
For our To-Do List API, the REST API defines several endpoints, each corresponding to a specific action:
GET /tasks
: Viewing All TasksThis endpoint provides a comprehensive list of all tasks stored in the application's database.
POST /tasks
: Adding a New TaskThrough this endpoint, users can add new tasks, ensuring they don’t forget that important activity they intended to do.
GET /tasks/{taskId}
: Accessing a Specific TaskSometimes, you might want to view the details of a specific task, especially if additional details like descriptions or due dates are attached. This endpoint serves that purpose.
PUT /tasks/{taskId}
: Updating a TaskPlans change, and so do tasks. Whether it's renaming a task or updating its status, this endpoint ensures adaptability.
HTTP Requests and Responses
The heart of any REST API lies in its ability to manage HTTP requests and deliver appropriate responses. Clients interact with the REST API by making HTTP requests. For Rest API Example, a GET request to /tasks
would retrieve all tasks. The server would respond with a JSON object containing all tasks.
Viewing All Tasks: GET Requests
To retrieve all tasks, the client makes an HTTP GET request:
The server then processes this request, accessing its database, and formulating a response:
Adding a New Task: POST Requests
Similarly, a POST request to /tasks
would create a new task. The request body would contain the details of the new task.
When adding a new task, the client sends a POST request with the new task's details:
Recognizing the creation of a new resource, the server acknowledges and indicates where this new task resides:
Modifying an Existing Task: PUT Requests
Updating a single task can be done by using the PUT HTTP verb. For modifications, like changing the title or updating a task's status, a PUT request is used:
The server confirms the changes and reflects them in its response:
Conclusion
REST APIs are a popular way to create web services. They use HTTP requests to GET, PUT, POST and DELETE data. RESTful web services use HTTP requests to perform operations on resources. By understanding the basics of REST APIs, you can create web services that are easy to use and maintainable.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.