JSON-RPC
In this section you will learn about JSON RPC APIs, what they are, their benefits and drawbacks with some real world examples.
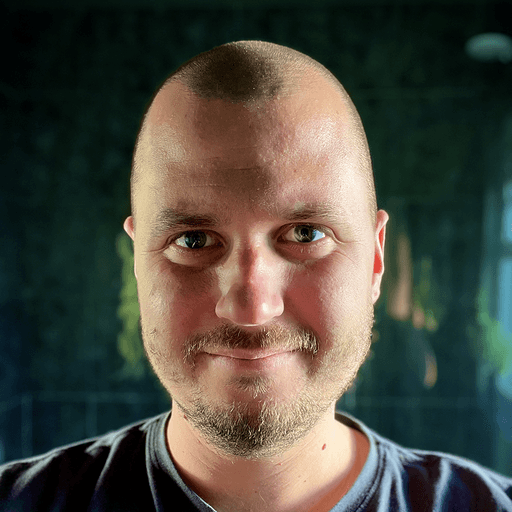
What is JSON RPC?
JSON-RPC is a remote procedure call (RPC) protocol encoded in JSON. It's a light and easy-to-use protocol that allows for structured data communication, making it a great choice for inter-process communication (IPC) or networked interactions. Unlike REST
which exposes resources as URLs, JSON-RPC exposes methods that you can call with arguments, get return values, or catch exceptions.
Principles of JSON RPC
Transport Agnostic: JSON-RPC doesn't tie you to one transport mechanism. It can be used over HTTP, WebSockets, TCP, or any other reliable transport layer. This quality makes it versatile for a wide range of applications.
Example: You can send a JSON-RPC message via HTTP like so:
In this example, the POST
request to the /jsonrpc
endpoint on example.com
contains a JSON-RPC message requesting a subtraction operation.
Stateless: Like
REST
, each call in JSON-RPC is stateless, which means that each request from a client to a server must contain all the information the server needs to fulfill the request.Simple and Lightweight: JSON-RPC's simplicity makes it a great choice for lightweight or lower power applications. The protocol's messages are easy to construct and parse, requiring minimal code.
Benefits of JSON RPC?
In a world with a plethora of protocols, JSON-RPC stands out due to its transport agnostic nature, simplicity, and lightweight structure, which make it a solid choice for various networking scenarios from server-to-server communication to client-server interaction.
Simplicity: JSON-RPC's protocol design is simple and intuitive, making it easy to implement and use. It follows a convention over configuration principle, meaning it has sensible defaults that help reduce the amount of decision-making and setup required to get it running.
Example: A basic JSON-RPC request to subtract two numbers looks like:
The method
field specifies the operation, and the params
field specifies the input values. The id
field is a unique identifier that helps match the request to the response, ensuring the client can correlate the received data to the initial request.
Transport Flexibility: Being transport agnostic, JSON-RPC can be used over HTTP, WebSockets, TCP, or any other transport mechanism, providing a lot of flexibility. This means you can send JSON-RPC messages over different transport layers without changing the message format, making it adaptable to different network configurations.
Example: The same
subtract
request can be sent over WebSocket, TCP, or any other transport mechanism without changing the JSON-RPC message.Structured Data Communication: JSON-RPC's use of JSON encoding allows for structured data communication, which is easily understandable both by machines and humans.
How does JSON RPC Work?
JSON-RPC operates on a simple send-receive model, making it straightforward to understand and implement:
Client Sends a Request
Clients send a request to the server encoded in JSON with a specified method and parameters.
In this request
, the method
field indicates the operation to perform, subtract
in this case, and the params
field provides the arguments for the method, here 42
and 23
.
Server Processes the Request
The server processes the request, performs the specified method with the provided parameters, and prepares a response. It decodes the JSON message, extracts the method name and parameters, performs the operation, and then prepares a JSON-encoded response.
Server Sends a Response to the Client
The server sends a response back to the client with the result or error of the request.
In this response
, the result
field contains the result of the subtraction operation, and the id
field matches the id
of the request, allowing the client to correlate the response to the original request.
Components of a JSON-RPC Request
Each JSON-RPC request is composed of several key components that provide the necessary information for the server to process the request:
jsonrpc
: This field specifies the version of JSON-RPC being used, which should be "2.0".
This tells the server that the client is using version 2.0 of the JSON-RPC protocol, ensuring compatibility.
method
: Themethod
field specifies the name of the method to be invoked on the server.
This is akin to calling a function in programming, where subtract
is the name of the function to be called.
params
: Theparams
field specifies the parameters of the method and can be an array or object.
This is akin to passing arguments to a function in programming, where 42
and 23
are the arguments to the subtract
function.
id
: Theid
field is a unique identifier for the request, which is used to match responses to requests.
This is a unique identifier for this request, allowing the client to match the response to the request.
JSON-RPC Error Handling
JSON-RPC has a structured error handling mechanism, providing clear error messages and codes. This is crucial for troubleshooting and understanding what went wrong when a request fails.
Error Object:
In this error object
, the code
field provides a standardized error code, the message
field provides a human-readable error message, and the data
field can provide additional data about the error.
Standard Error Codes:
JSON-RPC defines a set of standard error codes, like
-32602
for "Invalid params", making it easier to identify and handle errors in a standardized way.
Components of a JSON-RPC Response
When the server sends back a response, it provides the result of the method invocation or an error object if something went wrong:
jsonrpc
: This field specifies the version of JSON-RPC being used, which should be "2.0".
This ensures that the client and server are using the same version of the JSON-RPC protocol.
result
orerror
: Theresult
field contains the result of the method invocation, or theerror
field contains an error object if something went wrong.
or
The result
field contains the result of the subtraction operation, or the error
field contains an error object detailing what went wrong.
id
: Theid
field is a unique identifier for the response, matching theid
of the request.
This allows the client to correlate the response to the original request.
Real-world example of JSON-RPC
To illustrate JSON-RPC in action, let’s delve into a real-world scenario. Let’s say you are building a "To-Do List" application that allows users to manage their tasks. JSON-RPC can be used to handle the server-client interactions such as adding, deleting, or retrieving tasks.
API Endpoints in Action
For our hypothetical To-Do List application, the JSON-RPC defines several methods, each corresponding to a specific action:
getTasks
: Viewing All TasksThis method provides a comprehensive list of all tasks stored in the application's database.
Request:
Response:
addTask
: Adding a New Task
Through this method, users can add new tasks, ensuring they don’t forget that important activity they intended to do.
Request:
Response:
JSON-RPC vs GraphQL vs gRPC
When choosing a communication protocol for APIs, it's crucial to compare JSON-RPC, GraphQL, and gRPC, as each has unique features suited to different scenarios:

JSON-RPC stands out for its simplicity and flexibility over various transport layers, making it a good choice for straightforward, less bandwidth-intensive operations. GraphQL shines in situations where you need to fetch complex and varied data shapes in a single request, offering a powerful, flexible query language. gRPC is ideal for high-performance applications that benefit from HTTP/2 features, like server-side streaming, and require strict service contracts, commonly used in microservices architectures.
Ultimately, the choice among JSON-RPC, GraphQL, and gRPC will depend on the specific needs of your project, such as the complexity of the data operations, the performance requirements, and the preferred API style for the development team.
Conclusion
JSON-RPC is a powerful protocol that allows for simple, structured, and efficient communication between clients and servers. Its transport agnostic and stateless nature, along with its easy-to-understand JSON encoding, makes it a solid choice for various applications, enabling developers to build reliable and maintainable web services.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.