SOAP API
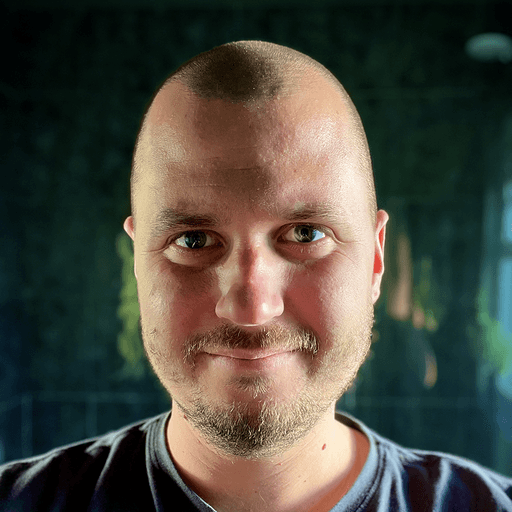
Luke Stephens
Nov 3, 2023
What is SOAP?
SOAP (Simple Object Access Protocol) is a messaging protocol for exchanging structured information in the implementation of web services. It acts as a messenger between applications. In simpler terms, think of SOAP as a courier that delivers messages between distant applications.
SOAP is XML-based, meaning it uses XML (eXtensible Markup Language) to encode its messages, and it can be carried over a variety of lower-level protocols, including the common HTTP (used in web browsers) and SMTP (used for email). Its primary purpose is to ensure programmatic functionality for web services, emphasizing extensibility, neutrality, and independence.
Learn the difference between SOAP and REST.
Principles of SOAP API
Extensibility: SOAP can be extended to accommodate various requirements, including security, transaction management, and more. This adaptability means it can grow and change according to needs.
For instance, consider this example:
In this SOAP message, the Header
element can be customized to contain extended information like security tokens, while the Body
holds the message's primary content.
Neutrality: SOAP is like a universal translator. It can operate over any protocol or transport layer, meaning it isn't tied to just one way of sending and receiving data. This includes HTTP, SMTP, and even more.
Independence: SOAP is versatile. It can be utilized with any programming model or language, ensuring interoperability between diverse systems. For instance, a SOAP message created in Java can be understood by a system using Python.
Why SOAP API?
At first glance, SOAP may seem like a complex structure of XML tags. However, its design emphasizes robustness, ensuring reliability and security for mission-critical applications.
Benefits of SOAP API
Standardized: SOAP provides a clear protocol and standard for exchanging messages, ensuring a consistent approach across different platforms. This means less room for error and smoother communication.
For instance, a SOAP message to retrieve user information might uses a consistent format, like this example:
Regardless of where it's coming from, the structure remains the same, ensuring it's always understood.
Reliable: Ever worried about a message not getting through? SOAP's built-in features, like message acknowledgment and retry logic, make sure messages are delivered reliably.
Secure: SOAP doesn't disappoint. With built-in security standards like WS-Security, SOAP provides a comprehensive security model. This ensures that the messages are only accessible to intended recipients and remain unaltered during transit.
How does SOAP API Work?
Much like when you send a letter and await a response, SOAP operates as a request-response mechanism:
Client Sends a Request
Just as you'd pen a letter, clients formulate a SOAP message and send it to request specific operations. It's like asking a question.
Server Processes the Request
Upon receiving the message (or question), the server processes the SOAP message, interprets the XML, and takes action. It's like the server is reading your letter and preparing a reply.
Server Sends a Response to the Client
Finally, the server sends back a response, much like receiving a reply to your letter. This message contains the answers or results.
Components of a SOAP Message
Imagine a SOAP message as a package. Each part of the package has a specific role, ensuring clarity and consistency in delivery:
Envelope: This is the package's outermost layer or wrapper. It holds everything inside and ensures the message is recognized as a SOAP message.
Header (Optional): Think of this as the special instructions on a package. This section contains any optional attributes of the message that might be needed for its processing, like authentication details.
Body: The
Body
is the main content of the package. It carries the actual message or the core information.
Fault (Optional): Sometimes, things go wrong. Within the
Body
, aFault
element can highlight errors that occurred during processing, acting as an error message.
SOAP Fault Handling
Mistakes happen. SOAP's built-in error handling mechanism, "SOAP Faults," shines a light on these errors, offering clarity on what went wrong:
Fault Codes: Think of these as error categories. SOAP defines a few basic fault codes to quickly identify the type of error, making troubleshooting smoother.
Fault String: This is a brief explanation of the fault. It provides a human-readable description, much like an error pop-up on your computer.
Detail: Just as a doctor provides specifics after a general diagnosis, the optional
Detail
element provides application-specific error information.
Example of SOAP API
Let's bring SOAP into a familiar scenario: the "To-Do List" application. SOAP can be used to manage tasks between a client and server, ensuring clear and secure communication for each task operation:
SOAP Methods in Action
listTasks
: Viewing All Tasks
This method retrieves all tasks stored in the database. Think of this as checking your task list in a to-do app, where the application sends a request to fetch all your tasks.
Request:
Response:
Conclusion
SOAP serves as a foundational protocol for web service communication, emphasizing reliability, security, and extensibility. When you think of SOAP API, envision a reliable courier, ensuring your messages are securely and consistently delivered, regardless of where they're coming from or going to.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.