GraphQL
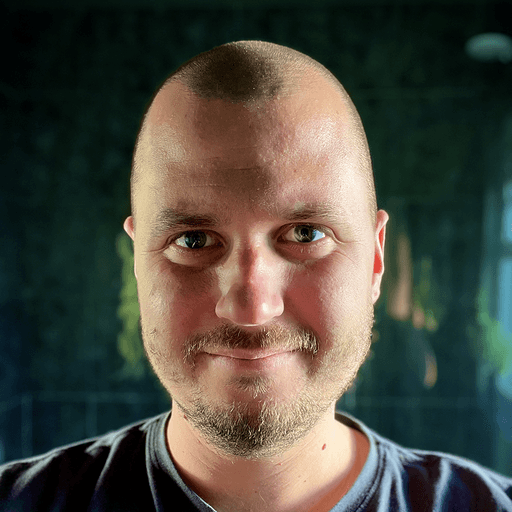
Luke Stephens
Nov 13, 2023
What is GraphQL?
GraphQL is a powerful query language for APIs and a runtime for fulfilling those queries with your existing data. Unlike REST
, it allows clients to request exactly the data they need, making it an efficient and robust alternative.
Learn the difference between GraphQL and REST.
Principles of GraphQL
Strong Typing: GraphQL enforces a schema that defines the types of data that can be queried and mutated. This schema serves as a contract between the client and server, ensuring consistency.
Example:
In this example, the type
keyword defines a new Book
type with title
and author
fields, where String!
indicates that the fields are non-nullable strings.
Hierarchical Structure: GraphQL’s hierarchical structure organizes data in a way that aligns with the layout of the UI, making it intuitive for frontend developers.
Example Query:
In this query, we're asking for a book
with a specific id
, and within that book, we want the title
and the author
's name
.
Real-time Updates (Subscriptions): GraphQL subscriptions allow real-time data updates, enabling dynamic and interactive user interfaces.
Example Subscription:
Here, the subscription
keyword is used to define a real-time subscription to listen for bookAdded
events.
Benefits of GraphQL?
GraphQL stands out due to its efficiency in data retrieval, real-time updates, and strong typing which ensures data consistency and aids in error prevention.
Efficiency: GraphQL queries allow clients to request exactly the data they need, preventing over-fetching or under-fetching of data.
Example Query:
This query is efficient because it only requests the title
of the book, nothing more, nothing less.
Real-time Updates: With subscriptions, GraphQL enables real-time updates that help in building dynamic and interactive UIs.
Example Subscription:
This subscription will notify the client in real-time whenever a new book
is added.
Strong Typing: The strong typing ensures that the API has a clear contract, preventing potential bugs and aiding in error prevention.
How does GraphQL Work?
Client Sends a Query
Clients send queries to the GraphQL server describing the data they need.
In this query
, we're asking for a book
with a specific id
, and we want to retrieve the title
and author
of the book.
Server Resolves the Query
The server processes the query, resolves it against the schema, and retrieves the data from the data sources.
Server Sends a Response to the Client
The server sends a response with the requested data to the client.
This response
contains the data as requested by the client in the same shape as the query
.
Components of a GraphQL Query
Fields: The properties you want to retrieve.
In this query
, title
and author
are fields
on the book
type.
Arguments: Parameters you can pass to fields to get specific data.
Here, id
is an argument
passed to the book
field to retrieve a specific book.
Aliases: Aliases allow you to rename fields in the result.
In this query
, firstBook
and secondBook
are aliases
for the book
field.
Fragments: Reusable pieces of queries.
In this query
, bookDetails
is a fragment
that is reused to get details of the book.
Components of a GraphQL Mutation
GraphQL mutations allow clients to change data on the server.
Mutation Definition:
In this Mutation
definition, we're defining a mutation
called addBook
that takes title
and author
as arguments
and returns a Book
type.
Mutation Query:
In this mutation query
, we're calling the addBook
mutation to create a new book and requesting the title
and author
fields in the response.
Example of GraphQL
Let's consider a social media application where users can post, like, and comment.
query getPosts
: Retrieving All PostsThis query provides a list of all posts.
This query
retrieves all posts, along with their title
, author
, and likes
.
mutation createPost
: Adding a New Post
This mutation allows users to create new posts.
This mutation
creates a new post with the given title
and content
, and returns the title
and author
of the new post.
query getPost
: Accessing a Specific Post
This query allows users to view a specific post.
This query
retrieves a specific post by id
, along with its title
, author
, and comments
.
mutation updatePost
: Updating a PostThis mutation allows users to update existing posts.
This mutation
updates the title
of the post with id
"1" to "Updated Title".
Conclusion
GraphQL is a robust and efficient alternative to traditional REST
APIs, offering precise data retrieval, real-time updates, and strong typing. By understanding its basics, developers can create more efficient, scalable, and maintainable web services, enhancing the overall development workflow and user experience.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.