GraphQL Query
In this section, you'll dive into the fundamentals of crafting queries in GraphQL, complete with examples to guide you. You'll learn how to structure queries to retrieve exactly what you need from a GraphQL API, understanding the syntax and components that make up a query. Through hands-on examples, you'll see how to efficiently fetch data, including specifying fields on objects, nesting objects for related data, and applying arguments to refine results, setting a solid foundation for working with GraphQL in your projects.
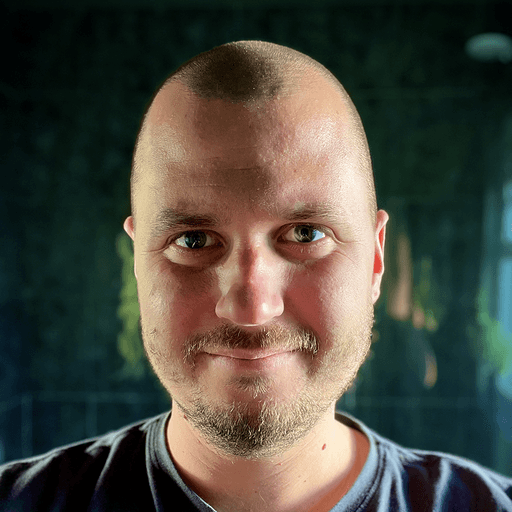
GraphQL stands as a query language for your API, and a server-side runtime for executing queries by specifying types and functions for each type in your data model. Unlike REST, where the server pre-defines how the client can access the data, GraphQL hands the power to the client, allowing it to request exactly the data it needs.
What is a GraphQL Query?
GraphQL Query is the means through which we request specific pieces of data from the server. They allow clients to specify precisely what data they need, potentially reducing the amount of data transferred over the network and improving performance.
1. Basic GraphQL Query
When you send a GraphQL query, you're describing your data shape in a syntax that mirrors the eventual response.
Description: The fields in a query correspond to the types in the server's data model, while the sub-fields correspond to the properties of those types.
Graphql Query Example: In this simple query, we ask for a list of books, and for each book, we want the title and the author.
2. GraphQL Query with Arguments
GraphQL isn't just about asking for specific fields on objects; you can also pass arguments to those fields, much like passing arguments to a function in many programming languages.
Description: Arguments in a query provide a way to make our queries dynamic, allowing for more specific data retrieval.
Graphql Query Example: In this scenario, we’re asking for details of a specific book by providing an
id
argument.
3. Aliases in GraphQL Query
Sometimes, you might need to make multiple calls to the same field but with different arguments. Aliases come in handy to handle such scenarios by allowing you to rename the result of a field to anything you want.
Description: Aliases can resolve naming conflicts in your query results, providing a way to request the same field with different arguments without confusion.
Graphql Query Example: Here,
firstBook
andsecondBook
are aliases that help differentiate between the two book objects in the response.
4. Directives in GraphQL Query
Directives in GraphQL add a dynamic element to our queries. They are preceded by a @
symbol and can modify the behavior of queries by including or skipping fields based on a condition.
Description: Directives, like
@include
and@skip
, provide a mechanism to dynamically alter the execution and response of our queries.Graphql Query Example:
5. Operation Name and Variables in GraphQL Query
Naming your operations and using variables in your queries can significantly improve the clarity and reusability of your code.
Description: Naming operations and using variables make your queries more readable and capable of handling dynamic values.
Graphql Query Example:
GraphQL Query Example with Request and Response
GraphQL Query Request
The request in a GraphQL system contains a query string and, optionally, variables. Here’s how it’s structured:
Operation Name: Naming your query is useful for debugging and server-side logging. When a query is named, it's easier to identify among other requests.
Graphql Query Example:
Query String: The core of your request, specifying the data you need, along with any required or optional arguments, aliases, and directives.
Graphql Query Example:
GetBookDetails
is the operation name which helps in logging and debugging.($bookId: ID!, $withAuthor: Boolean!)
are the variables being passed to the query.$bookId
is required to identify which book we're querying, and$withAuthor
is a directive condition to decide if we should include theauthor
field in the result.book
is the root field, andtitle
,author
,genre
, andpublication
are sub-fields. Thepublication
field has its sub-fieldspublisher
andyear
.
Variables: Optional dynamic values you might want to pass to your query.
Example:
Here, we're passing the actual values for the variables defined in the query string. This allows the query to be reusable with different values.
GraphQL Query Response
After the server processes your request, it sends back a response containing the requested data and potentially some metadata or errors.
Data: The actual content you requested, structured in the same shape as your query.
Example:
Here, the response has the same structure as the query. Each field in the query has a corresponding field in the response, and the publication
field has been broken down into publisher
and year
as requested.
Errors: Any errors that occurred while processing your query.
Example:
Here, an error occurred because the author
field was requested but does not exist on the Book
type in the GraphQL schema. The error message provides useful debugging information including the message, and the line and column in the query where the error was detected.
Conclusion
Through this module, we've explored the basic structure of GraphQL queries, how to make queries dynamic with arguments, aliases to manage multiple similar queries, directives for conditional field inclusion, and the importance of operation names and variables for better clarity and reusability.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.