GraphQL Authentication and Authorization
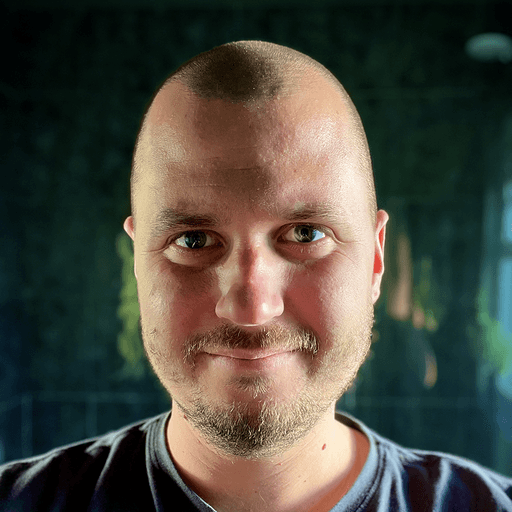
Luke Stephens
Feb 1, 2024
GraphQL Authentication and Authorization
While both authentication and authorization are pivotal in securing GraphQL APIs, they serve distinct functions in the security process:
GraphQL Authentication
Bearer Token Authentication: A widely-used method where clients send a token within the HTTP Authorization header to the server, which then validates the user based on the token.
OAuth 2.0: An authorization framework allowing applications to secure designated access. It operates by delivering an access token from the OAuth provider to the client.
Session-Based Authentication: Involves server-managed sessions with unique session IDs that are stored on the client's side, typically within cookies.
GraphQL Authorization
Role-Based Access Control (RBAC): Defines user roles with specific permissions, controlling their access to the API's resources.
Attribute-Based Access Control (ABAC): Grants or denies access by evaluating user attributes against resource attributes and environmental conditions.
Field-Level Permissions: Fine-tunes access control by assigning permissions directly to individual fields within the GraphQL schema.
Implementing Secure Access
API Keys: Simple security measure used as a unique identifier to control access, often in combination with other methods.
JSON Web Tokens (JWT): Compact tokens that securely encode user claims, sent in the HTTP Authorization header.
HTTPS: Encrypts data transmitted between client and server to protect against interception and ensure integrity.
Digital Signatures: Provides a cryptographic verification of the token or message to ensure it hasn't been altered in transit.
By carefully implementing and combining various strategies for both authentication and authorization, developers can create a secure GraphQL environment.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.