GraphQL Pagination
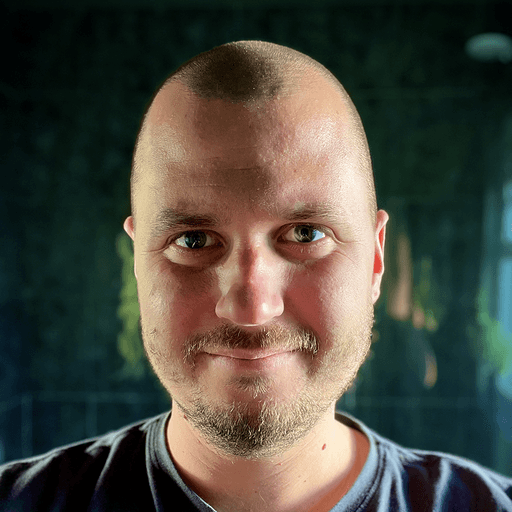
What is GraphQL Pagination?
GraphQL Pagination is a technique to fetch data in smaller, more manageable chunks, rather than all at once. This is crucial for performance especially when dealing with large datasets. Pagination allows clients to request data in specific increments, making data retrieval more efficient.
Types of GraphQL Pagination
There are several pagination strategies used in GraphQL. Here are the two common types:
Offset-based Pagination: This strategy uses a specific number to skip a certain amount of items before returning the requested data.
Cursor-based Pagination: This strategy uses a cursor to keep track of where in the data the next items should be fetched from.
Offset based Pagination
Offset-based pagination is straightforward and involves skipping a specified number of items before fetching the next set.
Description: Using an offset and a limit, clients can control the start point and the number of items to fetch.
Example: In this query, we're fetching books starting from the 6th item and retrieving the next five items.
Cursor based Pagination
Cursor-based pagination is complex but often more efficient. It utilizes a cursor to keep track of where in the dataset the next fetch should start.
Description: A cursor represents a unique point in the dataset, allowing for efficient data retrieval, especially in frequently updated datasets.
Graphql Pagination Example: In this query, we're fetching the first five books, and the
endCursor
will be used to fetch the next set of books.
Combining GraphQL Pagination with Other Features
Pagination can be combined with other GraphQL features like filtering and sorting to retrieve data in a more precise and controlled manner.
Description: Combining pagination with filtering and sorting allows for powerful, flexible data retrieval.
Graphql Pagination Example: In this query, we're fetching books written by a specific author, sorted by title, and paginated using cursor-based pagination.
Example of GraphQL Pagination Request
Real-world pagination requests in GraphQL are structured to fetch specific chunks of data efficiently.
Operation Name: Naming your pagination query aids in debugging and server-side logging.
Graphql Pagination Example:
Pagination Query String: Specifies the pagination strategy along with any other requirements like sorting or filtering.
Graphql Pagination Example:
In the above query:
FetchBooks
is the operation name for clarity and debugging.($first: Int!, $after: String, $orderBy: OrderByInput, $where: BooksWhereInput)
are the variables being passed to the pagination query. They're used to control the pagination, sorting, and filtering.books
is the root field, withedges
andpageInfo
as sub-fields to handle the pagination and return the paginated book details.
Variables: Dynamic values passed to the pagination query.
Graphql Pagination Example:
Example of GraphQL Pagination Response
The server processes the pagination request and returns a response containing the paginated data along with any pagination info.
Data: The paginated data returned from the query, structured according to the request.
Graphql Pagination Example:
Errors: Any errors encountered during the pagination query.
Example:
Best Practices for Implementing Pagination
Performance Considerations: Be aware of the performance implications of different pagination strategies, especially in large datasets. Offset-based pagination can be less efficient than cursor-based pagination as the dataset grows.
Providing Clear Errors: Ensure that error messages are clear and informative to help clients understand any issues with their pagination requests.
Combining with Other Features: Utilize the power of GraphQL by combining pagination with other features like filtering and sorting for more precise data retrieval.
Conclusion
This module has equipped you with the essential knowledge on GraphQL pagination, enabling you to fetch data in manageable chunks and improve the performance of your GraphQL server.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.