OpenAPI Specification
In this section you will learn about OpenAPI Specification and methods to generate OpenAPI Specification with examples
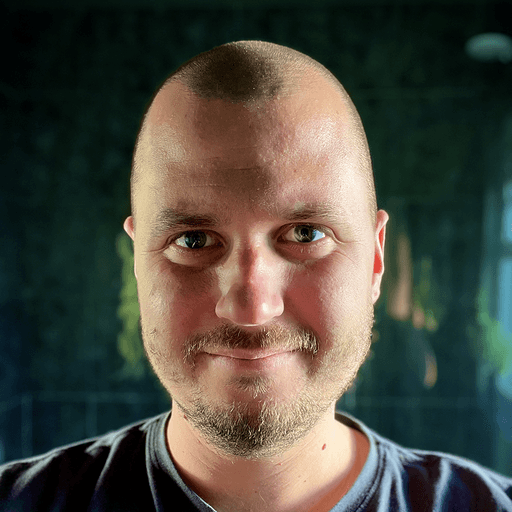
What is an OpenAPI Specification?
The OpenAPI Specification (OAS), formerly known as Swagger Specification, defines a standard, language-agnostic interface for RESTful APIs. The OpenAPI Specification File is a text file that describes your API following this standard. The OpenAPI Spec file is a document that describes all aspects of your API including:
Endpoints: The paths and operations available in your API. For example:
/books
,/books/{id}
.Operations: The HTTP methods supported by each endpoint, and their parameters. For example:
GET
,POST
.Responses: The possible responses from each operation, including error responses. For example:
200 OK
,404 Not Found
.Schemas: The data models used by your API. For example: defining a
Book
object with properties liketitle
,author
, andpublishedYear
.Security: The authentication methods supported by your API. For example: OAuth 2.0, API keys.
This file can be written in either YAML or JSON format, although YAML is often preferred for its readability. Here's a brief snippet of what an OpenAPI Spec file might look like:
Methods to Generate OpenAPI Specification File
Generating an OpenAPI specification file can be done through various methods:
1. Manual Creation:
This method is often chosen when you have a clear understanding of your API and the OpenAPI specification. Here’s a step-by-step breakdown:
Step 1: Setup
Choose a text editor of your preference. Common choices include Visual Studio Code, Sublime Text, or Atom.
Create a new file with an extension of
.yaml
or.json
, depending on which format you prefer to work with.
Step 2: Defining Basic Information
At the beginning of your file, define the basic information of your API including its title and version.
Step 3: Defining Endpoints
Define the endpoints of your API along with the supported operations (e.g., GET, POST, PUT, DELETE) and the expected responses.
Step 4: Defining Models
Define the data models used by your API, specifying the properties and types of data your API can handle.
Step 5: Save and Review
Save your file frequently to prevent any loss of work.
Review your file to ensure it adheres to the OpenAPI specification and accurately represents your API.
2. Automated Generation from Code (Annotations):
Automated generation from code involves annotating your API code with special comments or attributes, and then using a tool to scan your code and generate an OpenAPI spec file. This method can save a lot of time, especially for large or complex APIs. Here’s how you might go about it:
Step 1: Setup
Choose a library suited to your development environment. For .NET, Swashbuckle is a popular choice, while springdoc-openapi is often used with Spring Boot in Java projects.
Install the library into your project following the provided instructions.
Step 2: Annotate Your Code
Go through your API code, annotating endpoints, operations, parameters, responses, and models with the appropriate annotations or attributes provided by the library.
Step 3: Generate the Spec File
Use the library to scan your annotated code and generate an OpenAPI spec file. This usually involves running a command or building your project.
Step 4: Review and Adjust
Review the generated OpenAPI spec file to ensure it accurately represents your API.
Make any necessary adjustments to the file or your annotations, and regenerate the spec file as needed.
3. Conversion from Other Formats:
If you have existing API documentation in another format, converting it to OpenAPI can be a quick way to get started. Here’s a basic outline of the process:
Step 1: Choose a Conversion Tool
Select a tool that supports conversion from your existing documentation format to OpenAPI.
Step 2: Convert Your Documentation
Use the conversion tool to generate an OpenAPI spec file from your existing documentation.
Step 3: Review and Adjust
Review the generated OpenAPI spec file, making any necessary adjustments to ensure it accurately represents your API and adheres to the OpenAPI specification.
4. Using Online Editors:
Online editors like Swagger Editor provide a user-friendly interface for defining your API and generating an OpenAPI spec file. Here’s a simple guide:
Step 1: Access the Editor
Navigate to the online editor of your choice, like Swagger Editor.
Step 2: Define Your API
Use the editor to define your API according to the OpenAPI specification. The editor often provides real-time validation and feedback to help ensure your spec file is correct.
Step 3: Export Your Spec File
Once your API is fully defined and validated, export the OpenAPI spec file from the editor.
Step 4: Review
Review the exported OpenAPI spec file to ensure it meets your needs and make any necessary adjustments using either the online editor or a text editor of your choice.
Step-by-Step Guide to Generating OpenAPI Specification File
Using Swagger Editor:
Access Swagger Editor:
Navigate to Swagger Editor in your web browser.
Create New or Import Existing:
Click on
File
in the menu, then selectNew
to start a new OpenAPI spec file from scratch, orImport File
to upload an existing one.
Define Your API:
In the editor, define your API according to the OpenAPI Specification.
For example, define a
GET /books
operation to retrieve a list of books:
Validate Your Spec File:
Swagger Editor provides real-time validation as you type.
Look for any errors or warnings in the editor and correct them.
Export Your Spec File:
Once your spec file is complete and valid, click on
File
in the menu, then selectSave As
to export it as a YAML or JSON file.
Conclusion
The methods discussed in this module provide various options to suit different project needs. With a well-defined OpenAPI spec file, you can generate interactive documentation, client SDK generation, and more, making it a cornerstone of a successful API program. The easiest way to generate an OpenAPI spec file is through Akto. You can get started with Akto quickly using Helm charts.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.