Assess CORS Configuration with GitHub Copilot
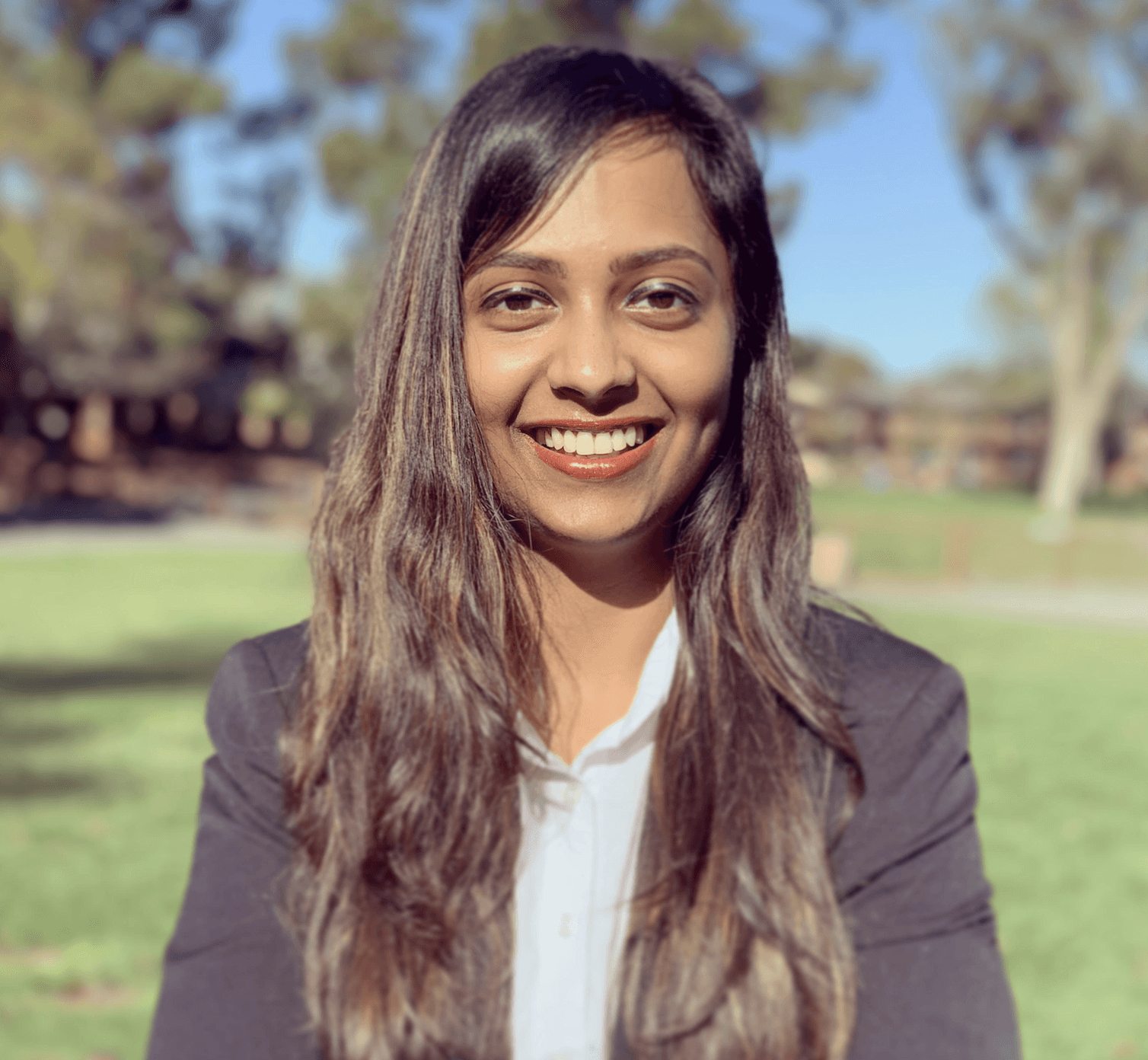
Ankita Gupta
Apr 25, 2024
Cross-Origin Resource Sharing (CORS) is a mechanism that allows or restricts requested resources on a web server depending on where the HTTP request was initiated. While CORS is essential for resource sharing across different origins, improper configurations can lead to significant security vulnerabilities, such as data breaches and unauthorized access.
Real-Life Scenario:
Imagine reviewing the CORS configuration in a Node.js application:
In this example, the application is configured to accept requests from any origin, which can be risky depending on the nature of the application and the data it handles.
Engaging GitHub Copilot:
Prompt to GitHub Copilot: "Analyze this CORS configuration for security risks."
Anticipated Copilot Analysis:
GitHub Copilot would scrutinize the CORS settings and might respond with:
Limit HTTP Methods: Restrict the methods to those that the application actually uses. For example, if your application does not need to handle
DELETE
requests, do not allow it.Credentials and Headers: If your application needs to handle credentials (cookies, session tokens, etc.), ensure that the CORS configuration correctly supports this by setting
credentials: true
, and validate the configuration of exposed headers.Review Regularly: CORS policies should be reviewed regularly to ensure they align with the current operational requirements and security practices of the application.
Common pitfalls when checking CORS configuration for security checks using GitHub Copilot include:
Overreliance on Automated Tools: While GitHub Copilot can provide valuable insights, it is important to remember that it is an AI tool and may not cover every potential security issue. Manual review by a skilled security expert is still essential.
Misunderstanding of Suggestions: GitHub Copilot can suggest changes to your code, but it is crucial to understand the implications of these changes before implementing them. Blindly following suggestions may lead to unexpected behavior or new vulnerabilities.
Overlooking Context-Specific Risks: GitHub Copilot can analyze code based on common security best practices, but it might not account for risks specific to your application or environment. Ensure to consider these when reviewing its recommendations.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.