Secure Patch for SQL Injection and XSS in JavaScript with GitHub Copilot
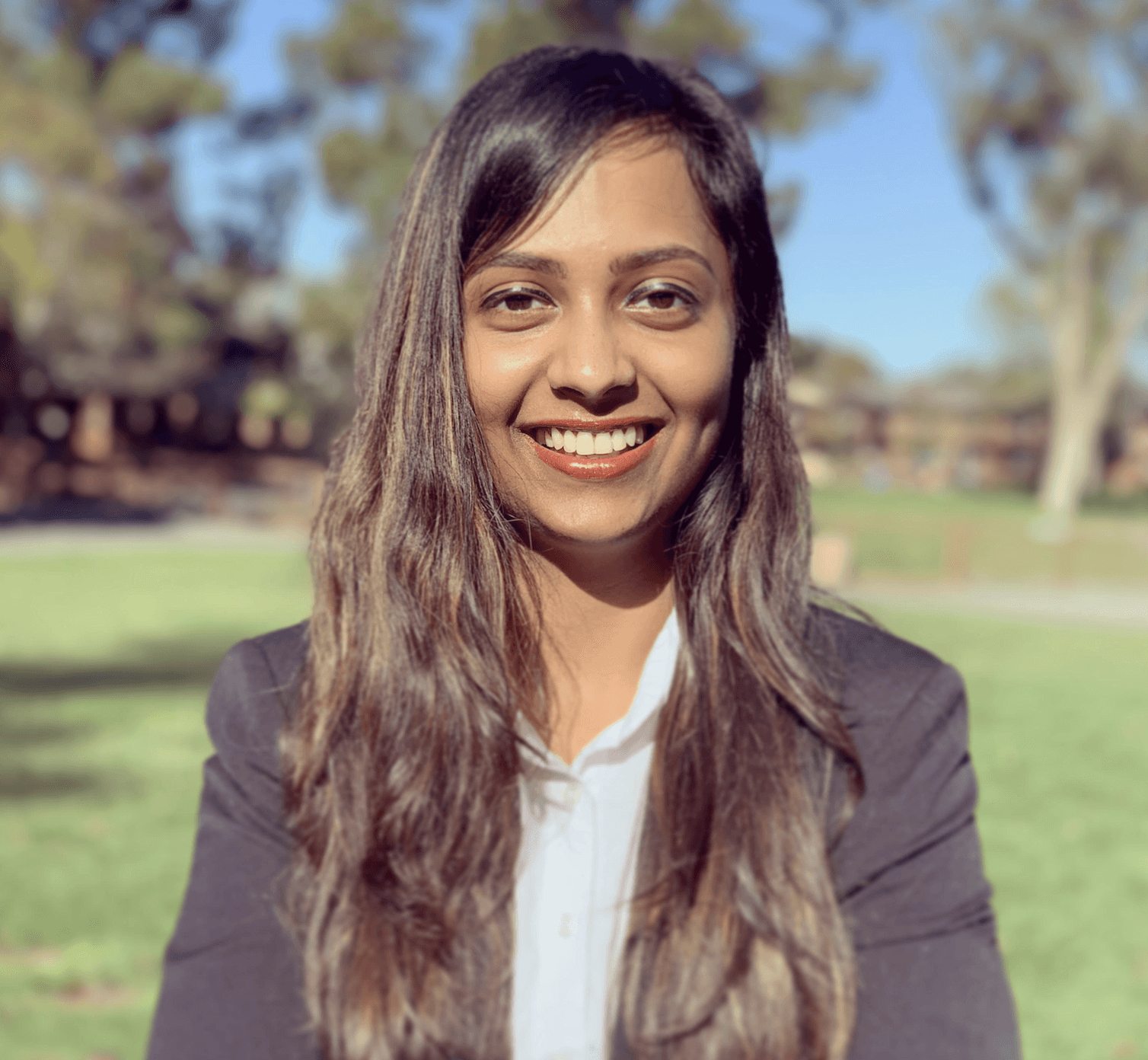
Addressing SQL injection and Cross-Site Scripting (XSS) vulnerabilities is importan for maintaining the security integrity of web applications. Below, we will review a JavaScript code snippet that contains these vulnerabilities and provide secure patches for them.
Real-Life Scenario:
Consider the following JavaScript code snippet that interacts with a database and renders user input:
In this example, the code directly incorporates user input into a SQL query and an HTML element, presenting risks for SQL injection and XSS, respectively.
Engaging GitHub Copilot:
Prompt to GitHub Copilot: "Provide a secure patch for the identified SQL injection and cross-site scripting (XSS) vulnerabilities in the following JavaScript code snippet."
Anticipated Copilot Analysis and Patch:
GitHub Copilot would analyze the provided code and suggest the following patches:
By using parameterized queries, you prevent attackers from manipulating the SQL query by injecting malicious SQL code through user input.
Cross-Site Scripting (XSS) Vulnerability Patch:
Escape user input before rendering it to the DOM or use textContent instead of innerHTML to prevent XSS attacks:
Alternatively, if you need to set HTML content safely, consider using a library like DOMPurify to sanitize the content:
By sanitizing the input or using textContent
, you prevent malicious scripts embedded in user input from being executed in the browser.
While GitHub Copilot can be a valuable tool in identifying and suggesting patches for code vulnerabilities, it’s important to be aware of common pitfalls:
Lack of Context: GitHub Copilot doesn't always understand the full context of your codebase. It might suggest a patch that doesn't work well with your specific setup.
Dependence on User Input: The quality of the output from GitHub Copilot largely depends on the quality of the input given by the user. Vague or incorrect instructions can lead to incorrect or unhelpful suggestions.
Updates & Maintenance: Codebases and programming languages are constantly evolving. The patch suggested by GitHub Copilot today might not be the best solution tomorrow, so continuous review and maintenance are necessary.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.