Evaluate Session Management with GitHub Copilot
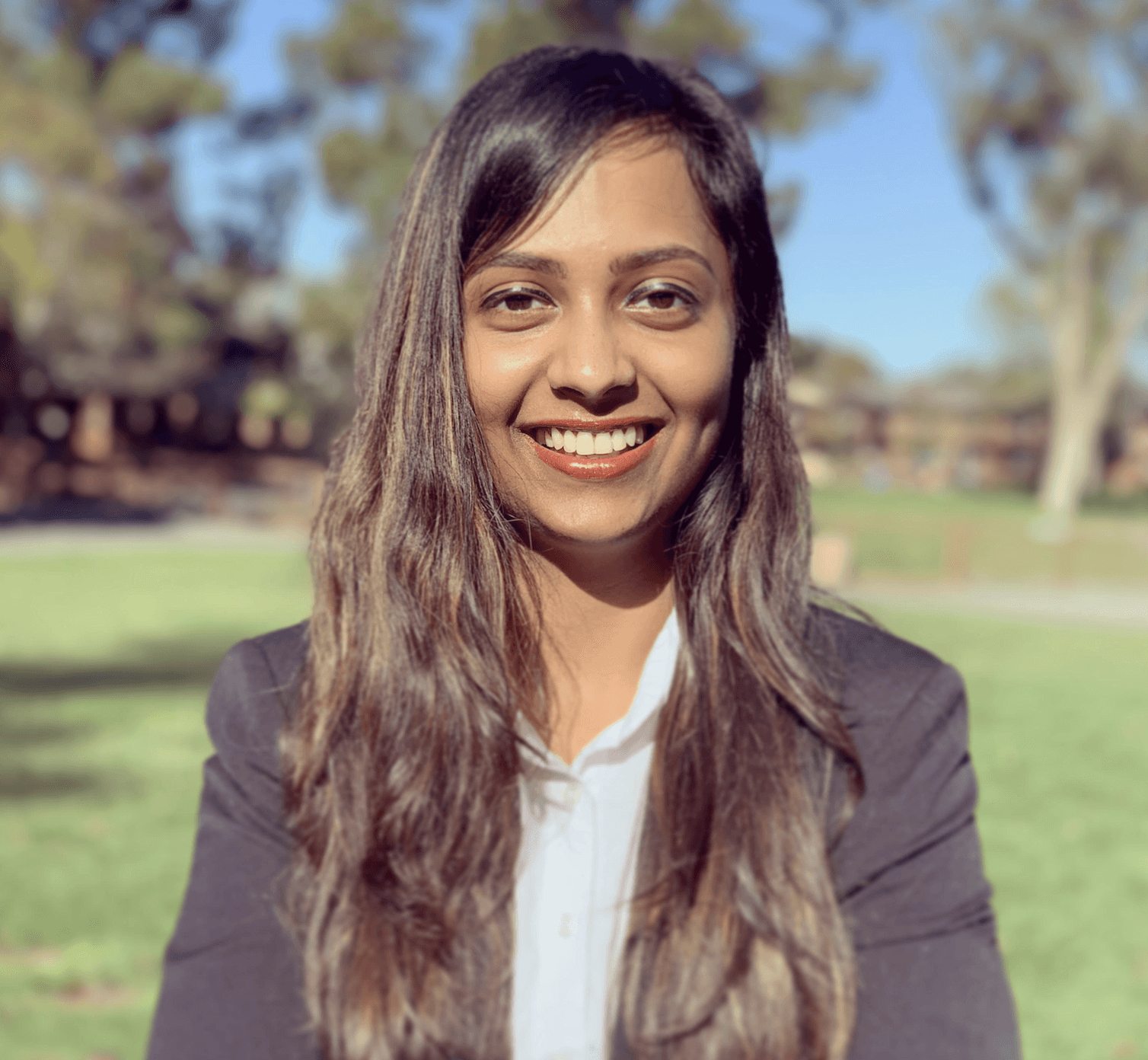
Effective session management is crucial for maintaining the security of user sessions in a web application. Poor session management can lead to a variety of security issues, including session hijacking, session fixation, and unauthorized access. Ensuring that session management is properly implemented and secure against potential vulnerabilities is essential for protecting user data and maintaining trust.
Real-Life Scenario:
Consider the following PHP code snippet that implements session management:
In this example, a session is started, and a check is performed to determine if the user is logged in based on the presence of a user_id
in the session.
Engaging GitHub Copilot:
Prompt to GitHub Copilot: "Review session management implementation for vulnerabilities."
Anticipated Copilot Analysis:
GitHub Copilot would analyze the session management strategy and could respond with:
By implementing these improvements, you can enhance the security of session management in your PHP application and protect against common session-related vulnerabilities.
There are several pitfalls that users might encounter when checking session management using GitHub Copilot:
Limited Context Awareness: While GitHub Copilot is an AI tool trained on a large dataset of code, it doesn't have full context of your entire codebase. Hence, its suggestions are based on the limited context it has, which may not always be accurate.
False Positives/Negatives: GitHub Copilot may give false positives (highlighting issues that are not actual vulnerabilities) or false negatives (missing actual vulnerabilities). It's essential to confirm its findings independently.
Overreliance: There might be a tendency to overly rely on GitHub Copilot for security checks, but it is not a substitute for manual code review, security testing, and understanding security principles.
Updates and Evolving Threats: Security threats evolve over time, and while GitHub Copilot is trained on a vast code dataset, it might not be up-to-date with the latest threats or vulnerabilities.
Remember, while GitHub Copilot can be a useful tool, it should be used in conjunction with other security practices, not as a standalone solution.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.