Evaluating Third-Party Service Integrations Security with GitHub Copilot
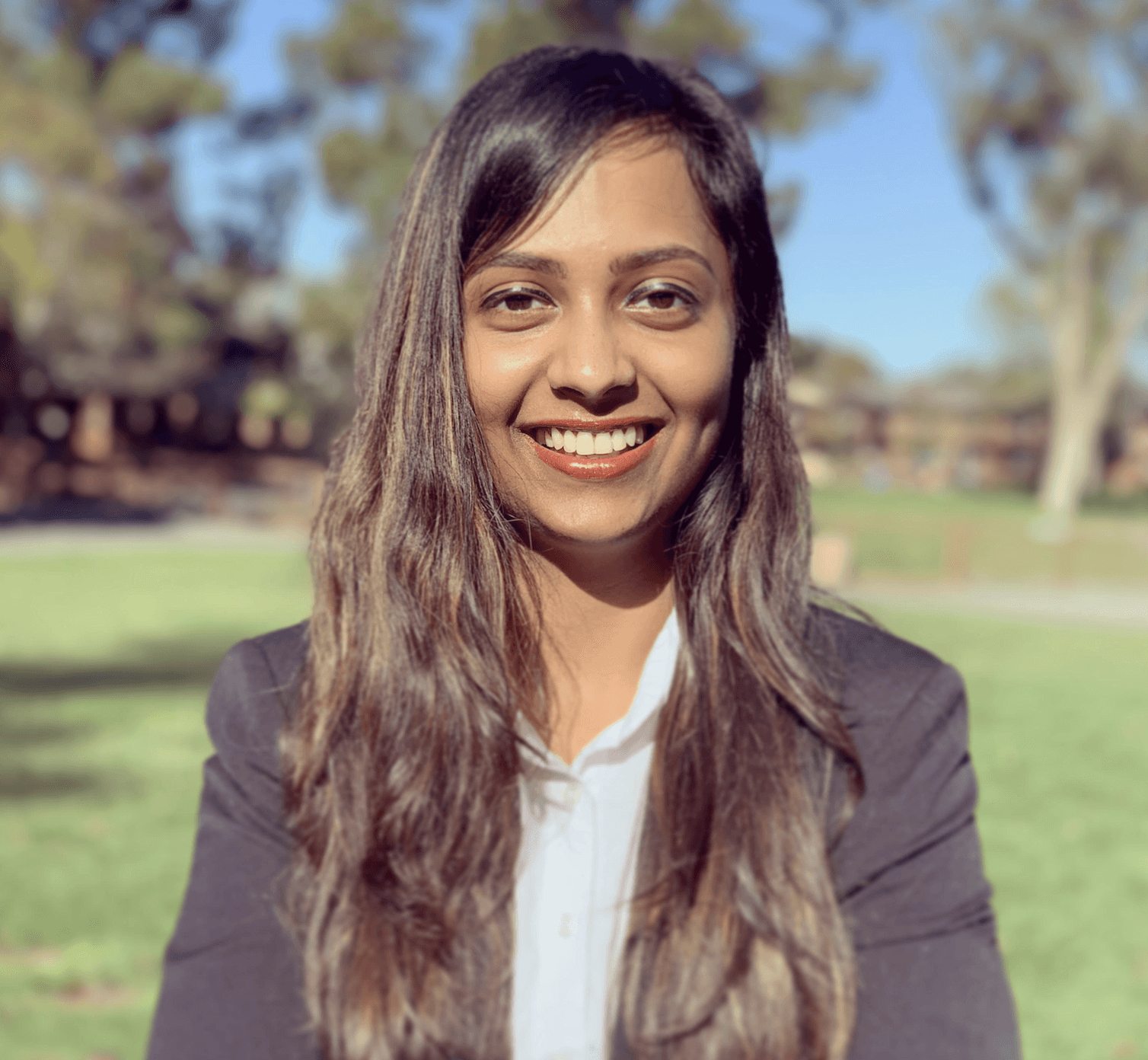
Integrating third-party services into your applications can add valuable features and functionalities. However, it also introduces potential security risks, as you are extending your security perimeter to include external services and data exchanges. It's important to evaluate and secure these integrations to protect your application from vulnerabilities that could arise from third-party services.
Real-Life Scenario:
Consider an application that integrates with a third-party payment processing service and a cloud storage provider:
In this example, API keys and sensitive user data are involved in interactions with third-party services.
Engaging GitHub Copilot:
Prompt to GitHub Copilot: "Evaluate the security of these third-party service integrations."
Anticipated Copilot Analysis:
GitHub Copilot would assess the provided integrations and could respond with:
By implementing these improvements, you can significantly enhance the security of your third-party service integrations, protecting both your application and your users' data.
Common Pitfalls when Checking Secure Third-Party Integrations Using GitHub Copilot:
Overlooking False Positives/Negatives: No tool is perfect. There might be instances of false positives (identifying a problem where there isn't one) or false negatives (missing a potential issue). Always double-check the findings.
Ignoring Context-Specific Risks: GitHub Copilot might not fully understand the context of your project. Therefore, it might overlook risks that are specific to your application's context or environment.
Neglecting Updates in Third-Party Services: The security measures suggested by GitHub Copilot might not account for recent updates or vulnerabilities in third-party services. Always stay updated about the third-party services you use.
Over-reliance on Tool Recommendations: While the recommendations provided by GitHub Copilot can be helpful, they should not be the only basis for your security strategy. Use multiple tools and resources to ensure a well-rounded security approach.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.