Validate Input Sanitization using Github Copilot
Cross-Site Scripting (XSS) is a prevalent web security vulnerability that allows attackers to inject malicious scripts into web pages viewed by other users. Input sanitization is a critical defense technique that involves cleaning user input validation to prevent malicious data from being executed as code.
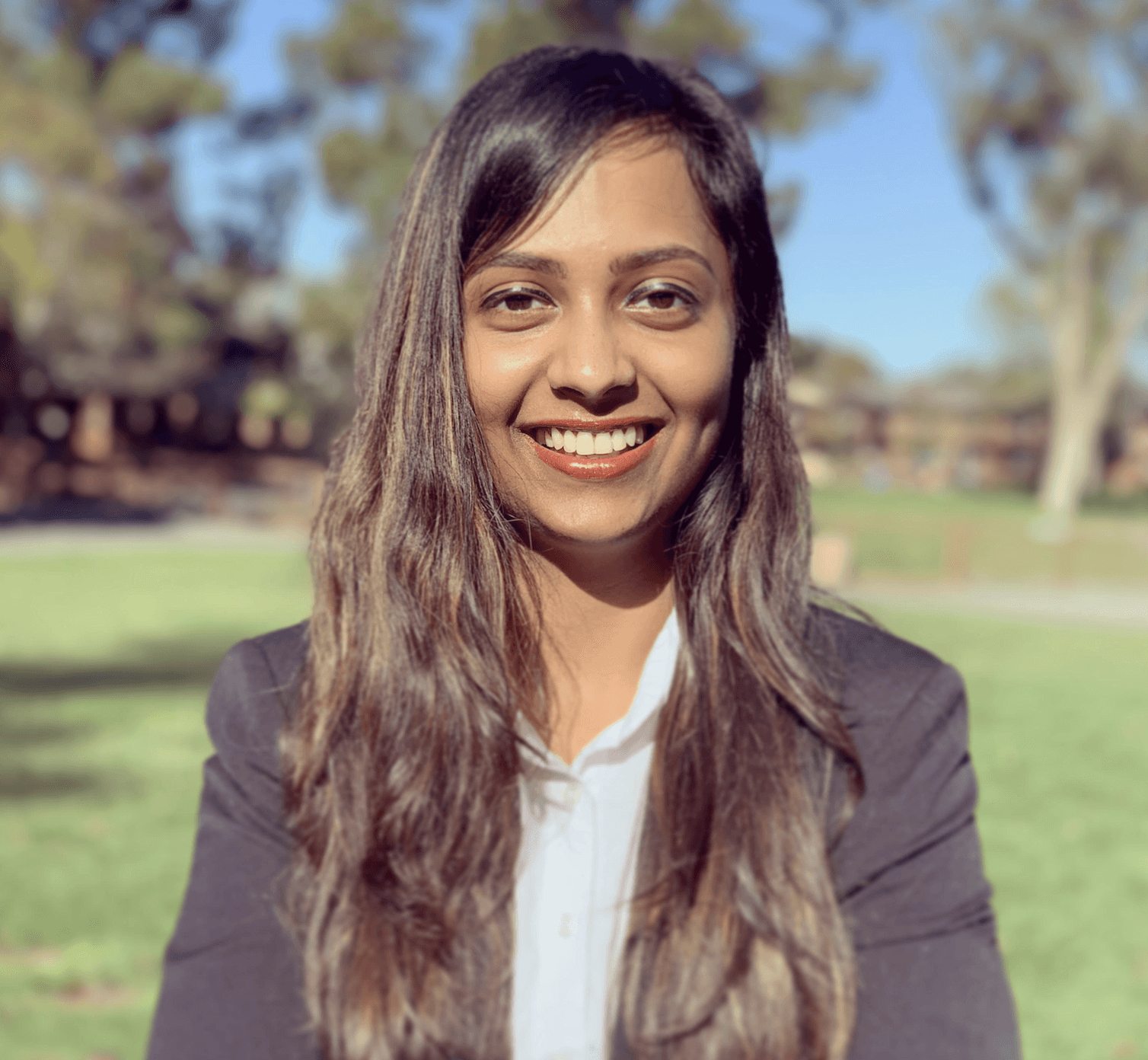
Ensuring that input sanitization functions effectively prevent XSS vulnerabilities is crucial for web application security.
Input Validation: Scenario
Consider a JavaScript function designed to sanitize user input for a web application:
This function atempts to remove <script>
tags from user inputs, aiming to prevent script injection and block XSS attacks.
Engaging GitHub Copilot:
Prompt to GitHub Copilot: "Check this input sanitization function for XSS vulnerabilities."
Anticipated Copilot Analysis:
GitHub Copilot would evaluate the sanitization approach and might respond with:
Alternatively, use a library like DOMPurify to sanitize HTML content securely:
These changes improve the robustness of the sanitization process, reducing the risk of XSS vulnerabilities.
While GitHub Copilot can provide valuable insights when validating input validation sanitization, there are several pitfalls to watch out for:
Over-reliance on AI: GitHub Copilot is an AI-powered tool and, while highly advanced, it may not catch all potential vulnerabilities.
Limited contextual understanding: GitHub Copilot might not fully understand the context of the code it is analyzing. As a result, it might not catch vulnerabilities that are context-specific.
False positives/negatives: Like any automated tool, GitHub Copilot is prone to false positives (identifying a vulnerability that doesn't exist) and false negatives (not identifying a vulnerability that exists).
Constantly evolving threats: Web security threats continually evolve, and new vulnerabilities can emerge. GitHub Copilot's advice is only as good as its training data, and it might not be aware of the latest threats.
Always complement the use of GitHub Copilot with manual code reviews by experienced developers, and consider using other security tools for a comprehensive evaluation.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.