Detect SQL Injection Using Github Copilot
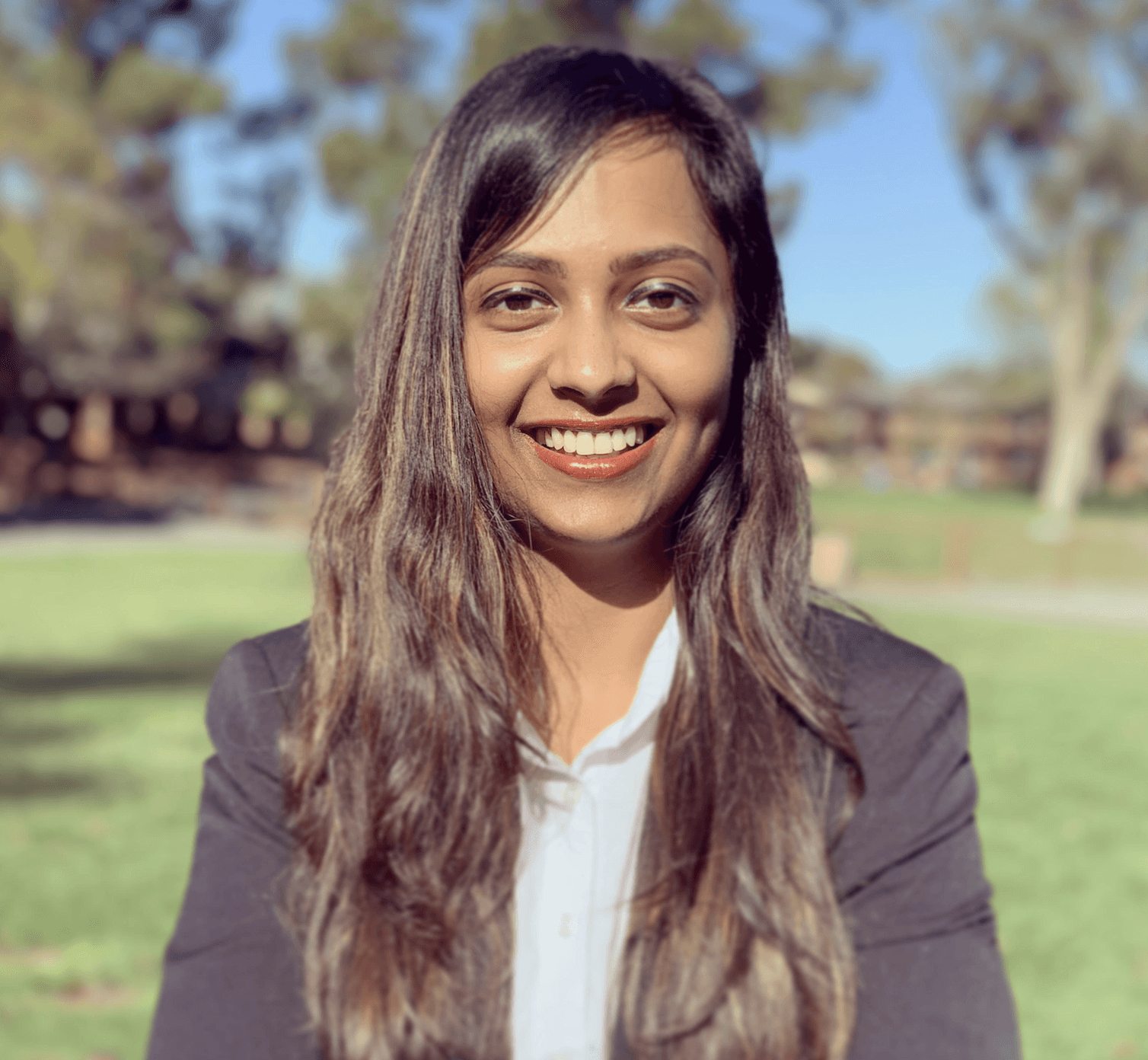
Ankita Gupta
Mar 19, 2024
SQL injection is a prevalent security issue where an attacker manipulates SQL queries by injecting malicious SQL code. This can lead to unauthorized data access, deletion, or manipulation. Reviewing SQL queries for vulnerabilities is crucial in preventing these attacks.
Here’s how one might approach this using an AI assistant like GitHub Copilot, illustrated through a real-life coding scenario.
Scenario:
Consider the following SQL query in a Node.js application using a MySQL database:
This example directly incorporates user input (username
and password
) into the SQL statement, presenting a clear risk for SQL injection.
Engaging GitHub Copilot:
Prompt to GitHub Copilot: "Review this SQL query for injection vulnerabilities"
Anticipated Copilot Analysis:
GitHub Copilot would identify the vulnerability and recommend best practices:
This approach separates the data (user input) from the code (SQL query), effectively preventing SQL injection.
Common Pitfalls of Detecting SQL Injection Vulnerabilities with GitHub Copilot:
Over-reliance on AI: While GitHub Copilot can be a valuable tool for identifying potential vulnerabilities, it should not be the sole method of security testing. Manual code reviews and other security testing tools also play a critical role in identifying and mitigating potential risks.
Limited to Code-Level Vulnerabilities: GitHub Copilot can help identify code-level vulnerabilities such as SQL injection, but it's not designed to detect configuration-level vulnerabilities or issues arising from the server or network level.
Lack of Updates: AI models like GitHub Copilot depends on the data it was trained on. If the model isn't regularly updated, it may not be aware of the latest vulnerabilities or best practices.
No Replacement for Secure Coding Practices: Tools like GitHub Copilot are meant to supplement, not replace, secure coding practices. Developers should still be well-versed in secure coding principles and practices.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.