Check for Secure Communication Protocols using Github Copilot
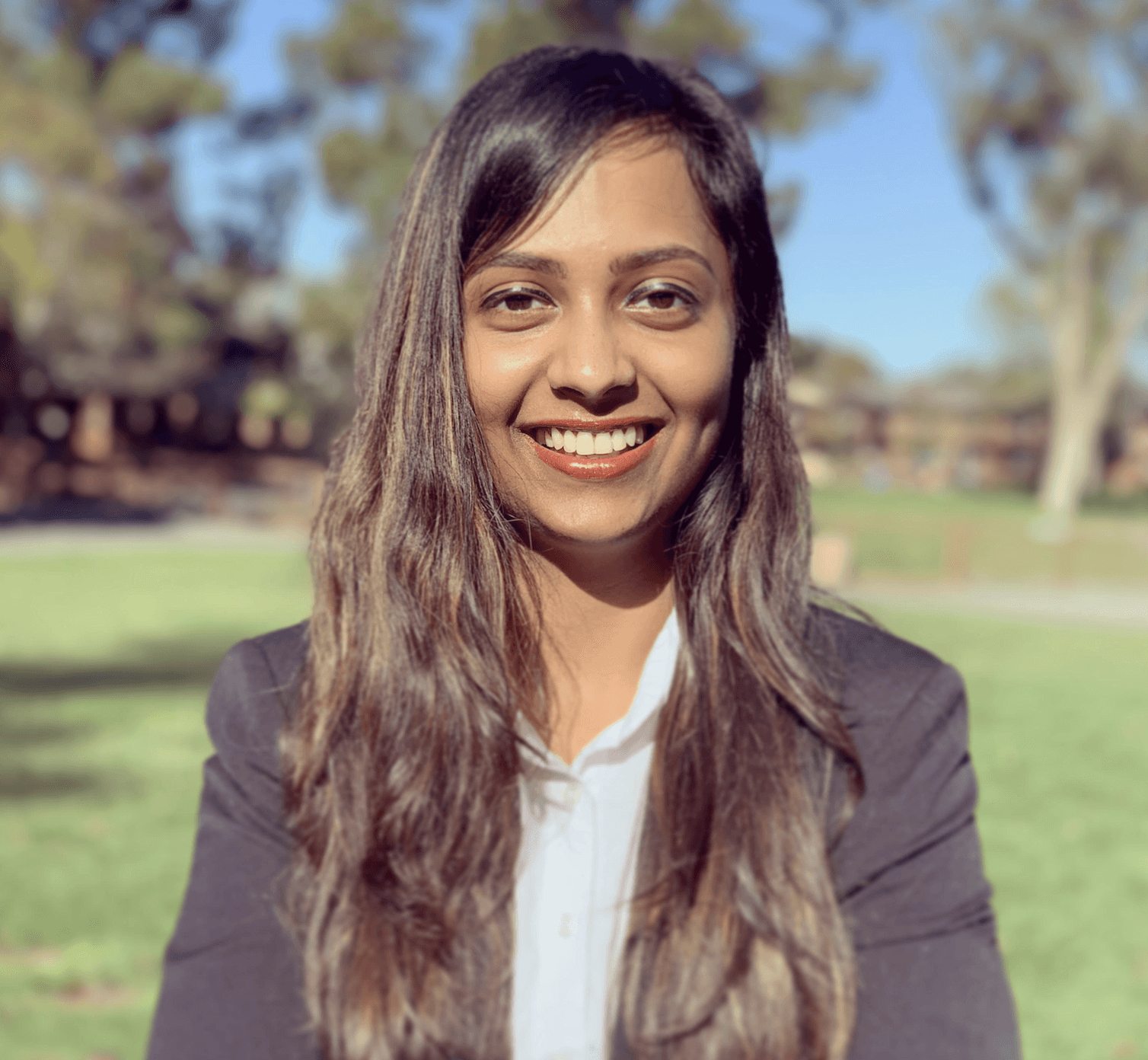
Ankita Gupta
Mar 14, 2024
Secure communication protocols, particularly HTTPS (Hypertext Transfer Protocol Secure), are essential in protecting data in transit. They prevent attackers from intercepting or tampering with the data sent between a client and a server. Ensuring that a service uses HTTPS for all its endpoints is a critical step in maintaining the confidentiality and integrity of user data.
Scenario:
Imagine you're reviewing a Node.js web service configuration to ensure it enforces HTTPS across all endpoints:
In this example, the service is set up using Express.js and is listening for requests on port 3000, which by default, would be over HTTP.
Engaging GitHub Copilot:
Prompt to GitHub Copilot: "Ensure this service uses HTTPS for all its endpoints."
Anticipated Copilot Analysis:
GitHub Copilot would evaluate the existing service setup and likely respond with:
By applying these changes, you can ensure that your service uses HTTPS for all endpoints, protecting data in transit from eavesdropping and tampering.
Common pitfalls when checking for secure communication protocols using GitHub Copilot include:
Relying Solely on Generated Code: While GitHub Copilot can generate code snippets, it's important to remember that it doesn't guarantee the security of your application. Always review and test the generated code before implementation.
Misconfiguration: Using HTTPS involves multiple moving parts, including certificates, private keys, and server configuration. A small misconfiguration can lead to vulnerabilities. It's crucial to thoroughly review the configuration provided by GitHub Copilot to ensure it aligns with best practices for secure communication protocols.
Overlooking HTTP to HTTPS Redirection: While GitHub Copilot might suggest redirecting HTTP traffic to HTTPS, it's easy to overlook this critical step, potentially leaving some endpoints unsecured.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.