Conduct a Security Assessment of REST API Endpoints with GitHub Copilot
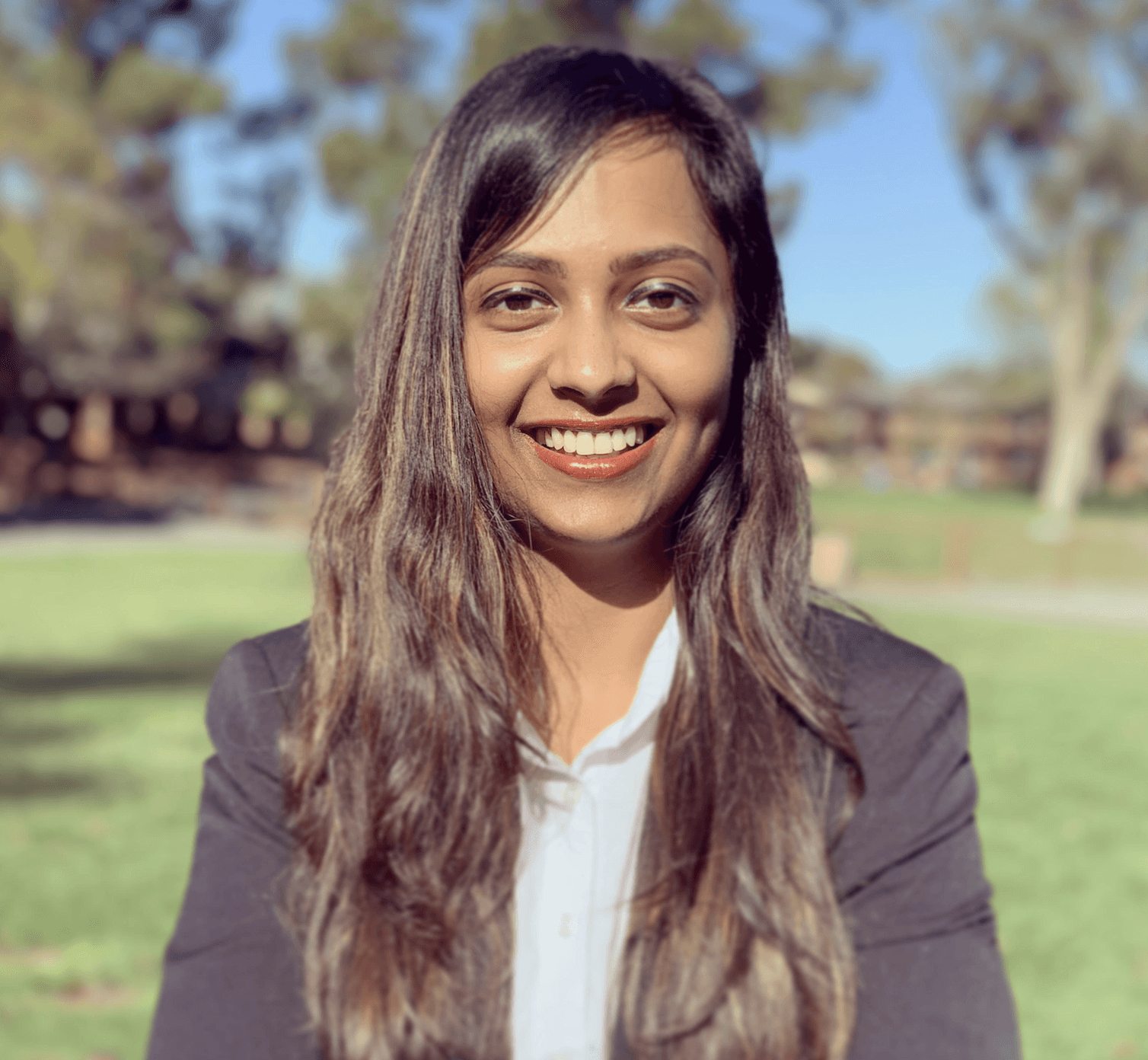
Ankita Gupta
Apr 8, 2024
APIs (Application Programming Interfaces) are critical components of modern web services, enabling interoperability between different software applications. However, they can also introduce security vulnerabilities if not properly secured. Conducting a security assessment of REST API endpoints is essential to identify and mitigate potential security risks.
Real-Life Scenario:
Consider a set of REST API endpoints in a Node.js application:
In this example, the application provides basic CRUD operations for user data through its REST API.
Engaging GitHub Copilot:
Prompt to GitHub Copilot: "Conduct a security assessment of these REST API endpoints."
Anticipated Copilot Analysis:
GitHub Copilot would analyze the REST API endpoints and might respond with:
By addressing these concerns, you can significantly improve the security of your REST API endpoints and protect against common web vulnerabilities.
While GitHub Copilot can be a handy tool in assessing API security, it's important to be aware of some common pitfalls:
Reliance on Automated Suggestions: GitHub Copilot provides coding suggestions based on its training data. However, it is not capable of understanding the full context of your application's security requirements. Therefore, it should not be solely relied on for security assessments.
Lack of Update on New Vulnerabilities: GitHub Copilot is not updated in real-time as new vulnerabilities and exploits are discovered. Therefore, its suggestions may not account for the most recent security threats.
Absence of Human Insight: While GitHub Copilot can provide automated suggestions, it lacks the ability to provide human insight that comes from experience and understanding of the unique intricacies of your specific application.
Therefore, while GitHub Copilot can be a useful tool in identifying potential security issues, it should be used as a part of a larger, more comprehensive security assessment strategy.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.