Detect Hardcoded Secrets with GitHub Copilot
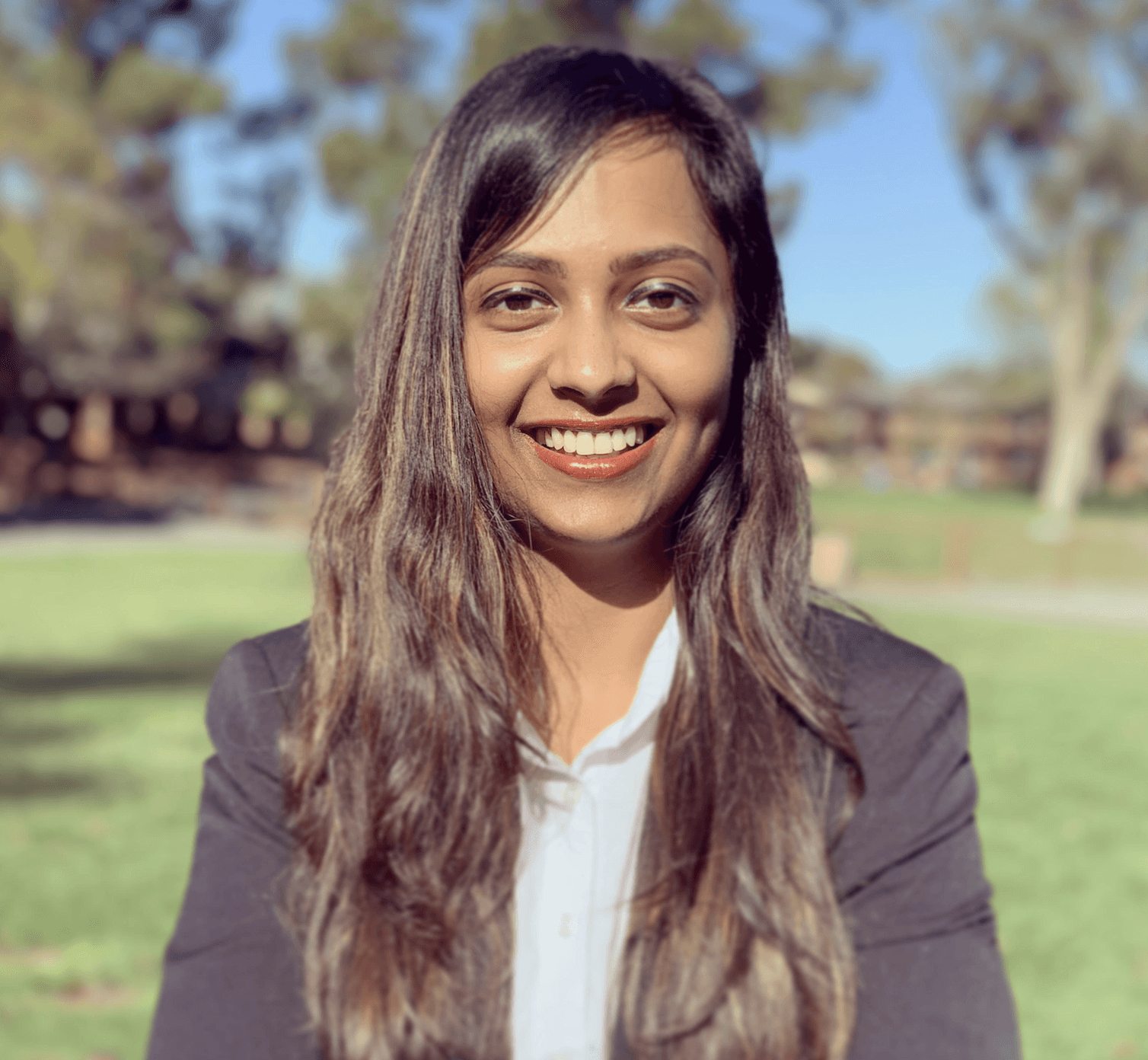
Hardcoded secrets, such as API keys, passwords, and cryptographic keys, pose a significant security risk if they are embedded directly within source code. These secrets can be easily exposed through version control systems, shared code, or other means, leading to unauthorized access and potential data breaches.
Scanning and removing hardcoded secrets from your codebase is important for maintaining security.
Real-Life Scenario:
Imagine reviewing a segment of a Python application that interacts with a cloud storage service:
In this example, AWS access and secret keys are hardcoded directly into the function.
Engaging GitHub Copilot:
Prompt to GitHub Copilot: "Scan this codebase for hardcoded secrets."
Anticipated Copilot Analysis:
GitHub Copilot would identify the security risks and might respond with:
Secrets Management Tools: Utilize AWS Secrets Manager, HashiCorp Vault, or other secrets management tools to securely store and access secrets. These tools provide additional security features such as access control, secret rotation, and audit logs.
Code Scanning Tools: Integrate code scanning tools into your development workflow to automatically detect hardcoded secrets. Tools like GitGuardian, TruffleHog, or AWS CodeGuru can help identify and prevent the commitment of secrets into version control.
Common Pitfalls for Detecting Hardcoded Secrets Using GitHub Copilot:
Overreliance on Automation: While GitHub Copilot can aid in identifying hardcoded secrets, it should not be the sole tool used for this purpose. Manual code reviews and other automated tools should also be employed to ensure all secrets are detected.
False Positives and Negatives: GitHub Copilot, like any tool, may produce false positives and negatives. It may flag certain parts of the code as containing hardcoded secrets when they do not, or it may miss certain hardcoded secrets. It is essential to double-check flagged code and not solely rely on the tool's results.
Ignoring Context: GitHub Copilot might not fully understand the context of the code. It might not be able to recognize if a particular hardcoded string is an actual secret or just a placeholder or test data.
Not a Substitute for Secure Coding Practices: Using GitHub Copilot to detect hardcoded secrets does not replace the need for secure coding practices. Developers should be educated on the risks of hardcoded secrets and how to avoid them in the first place.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.