Review Authentication Mechanism using Github Copilot
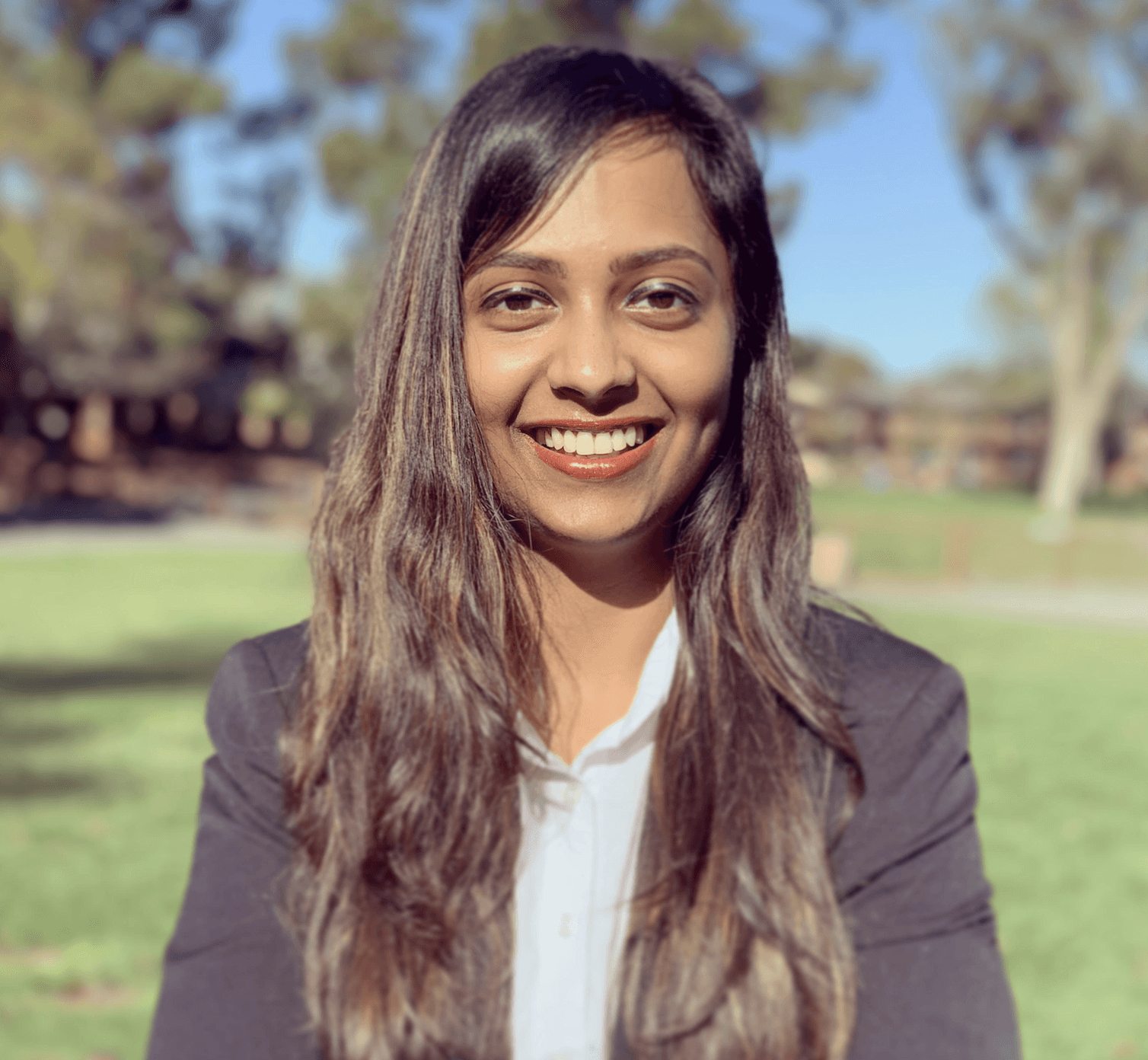
JSON Web Tokens (JWT) are a popular method for implementing token-based authentication in web applications. They enable secure transmission of information between parties as a JSON object, cryptographically signed.
Reviewing the security of JWT authentication implementations is important to ensure that they are not vulnerable to common exploits and are correctly protecting user data.
Real-Life Scenario
Consider a simplified example of a JWT authentication mechanism implemented in a Node.js application:
This function attempts to authenticate a user by verifying a JWT passed in the Authorization
header of the request.
Engaging GitHub Copilot
Prompt to GitHub Copilot: "Evaluate the security of this JWT authentication implementation."
Anticipated Copilot Analysis
GitHub Copilot would assess the security aspects of the implementation and might respond with:
Incorporating these recommendations will strengthen the security of the JWT authentication mechanism in your application.
GitHub Copilot is a valuable tool to assist in reviewing an authentication mechanism, but there are common pitfalls to be aware of:
Limited Context Understanding: GitHub Copilot may not fully understand the context of the code it's analyzing. It might miss nuances that a human reviewer would catch.
Dependence on AI Suggestions: Relying solely on GitHub Copilot may lead to overlooking other potential issues. It should be used as a tool to aid review, not replace a human reviewer.
Potential for Misinterpretation: Suggestions from GitHub Copilot need to be interpreted correctly. Misinterpretation of these suggestions can lead to flawed implementations.
Lack of Real-Time Updates: The AI model of GitHub Copilot might not be up-to-date with the latest security practices. Regular training and updates are essential to maintain its effectiveness.
Despite these pitfalls, when used correctly, GitHub Copilot can be a powerful tool in helping to review and improve the security of an authentication mechanism.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.