Check for Proper Error Handling with GitHub Copilot
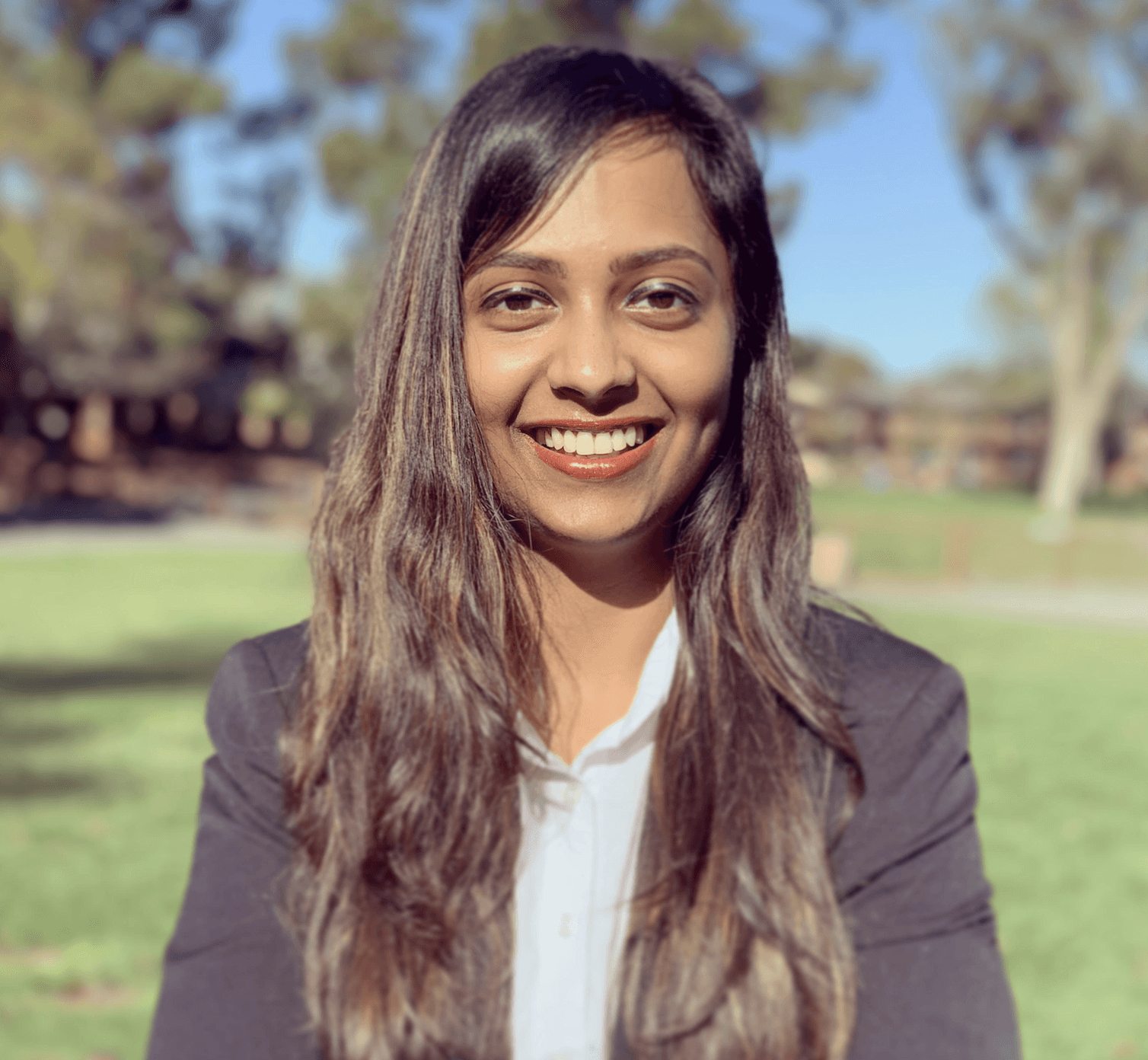
Proper error handling is crucial in any application for both usability and security. Inadequate error handling can lead to unintended information disclosure, providing attackers with insights into the backend system, its frameworks, and its vulnerabilities. Implementing secure error handling practices can mitigate such risks.
Real-Life Scenario:
Consider the following error handling code in an Express.js application:
In this example, the application logs the stack trace and sends a generic error message to the client.
Engaging GitHub Copilot:
Prompt to GitHub Copilot: "Inspect this error handling code for security best practices."
Anticipated Copilot Analysis:
GitHub Copilot would review the error handling strategy and might respond with:
By adopting these improvements, your application's error handling will be more secure and effective, providing necessary information to the users while protecting sensitive details and aiding system administrators in troubleshooting.
Common Pitfalls When Checking Error Handling with GitHub Copilot:
Overreliance on Copilot's Feedback: GitHub Copilot is a powerful tool, but it's not perfect. It's essential to understand that it may not catch all security vulnerabilities or provide an exhaustive list of best practices. Always ensure to double-check and validate the feedback from Copilot against known security best practices.
Limited Contextual Understanding: Copilot does not fully understand the context of your application. It can provide general advice, but it may not be aware of specific business rules, security requirements, or the application's unique aspects.
Inadequate Testing: Even with advice from GitHub Copilot, it's important to test error handling code thoroughly. Automated tools can overlook some issues that only manual testing can uncover.
Lack of Updates: Security best practices and vulnerability landscapes are constantly evolving. GitHub Copilot's training may not include the most recent information or reflect current best practices.
Remember, while GitHub Copilot can be a valuable tool for improving security, it should be part of a broader, comprehensive approach to application security.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.