Enforce Principle of Least Privilege with GitHub Copilot
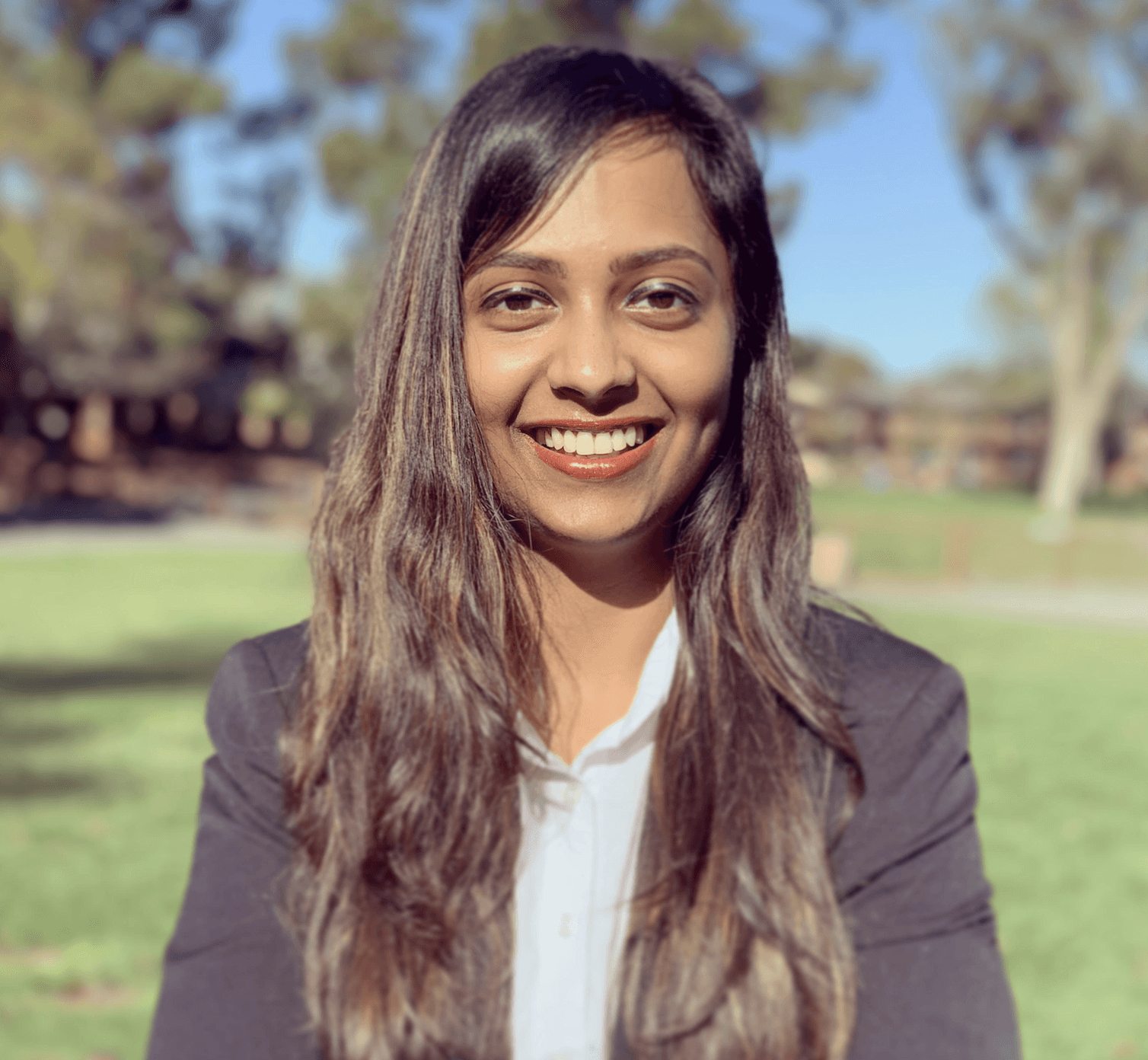
The Principle of Least Privilege (PoLP) is a fundamental concept in security, stating that users should be granted the minimum levels of access — or permissions — needed to perform their functions. This minimizes the potential damage from accidents, errors, or unauthorized use of system privileges. Ensuring that user access control in your code adheres to this principle is important for maintaining a secure software.
Scenario:
Consider this segment of a hypothetical web application managing user roles and access levels:
In this example, users are granted access based on their role, and sensitive actions are restricted to users with 'full' access.
Engaging GitHub Copilot:
Prompt to GitHub Copilot: "Check if the user access control in this code adheres to the principle of least privilege."
Anticipated Copilot Analysis:
GitHub Copilot would examine the user access control implementation and could respond with:
This version ensures that permissions are assigned more granularly and that users receive only the access they need to perform their functions, aligning better with the principle of least privilege.
Here are some common pitfalls when using GitHub Copilot to check if the principle of least privilege is ensured:
Reliance on Generic Prompts: GitHub Copilot can generate code based on a given prompt. However, prompts that are too generic may not yield the most effective solutions for specific situations. It's important to provide detailed and context-specific prompts.
Failure to Review Generated Code: While GitHub Copilot can generate code, it's crucial to review this code thoroughly. It may not always adhere to the principle of least privilege or other best practices. Users should not trust the generated code blindly.
Ignoring Role-Specific Permissions: GitHub Copilot might not consider the specific permissions required for each role in the system. Users need to specify these details to ensure that generated code satisfies the principle of least privilege.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.