Secure File Uploads with GitHub Copilot
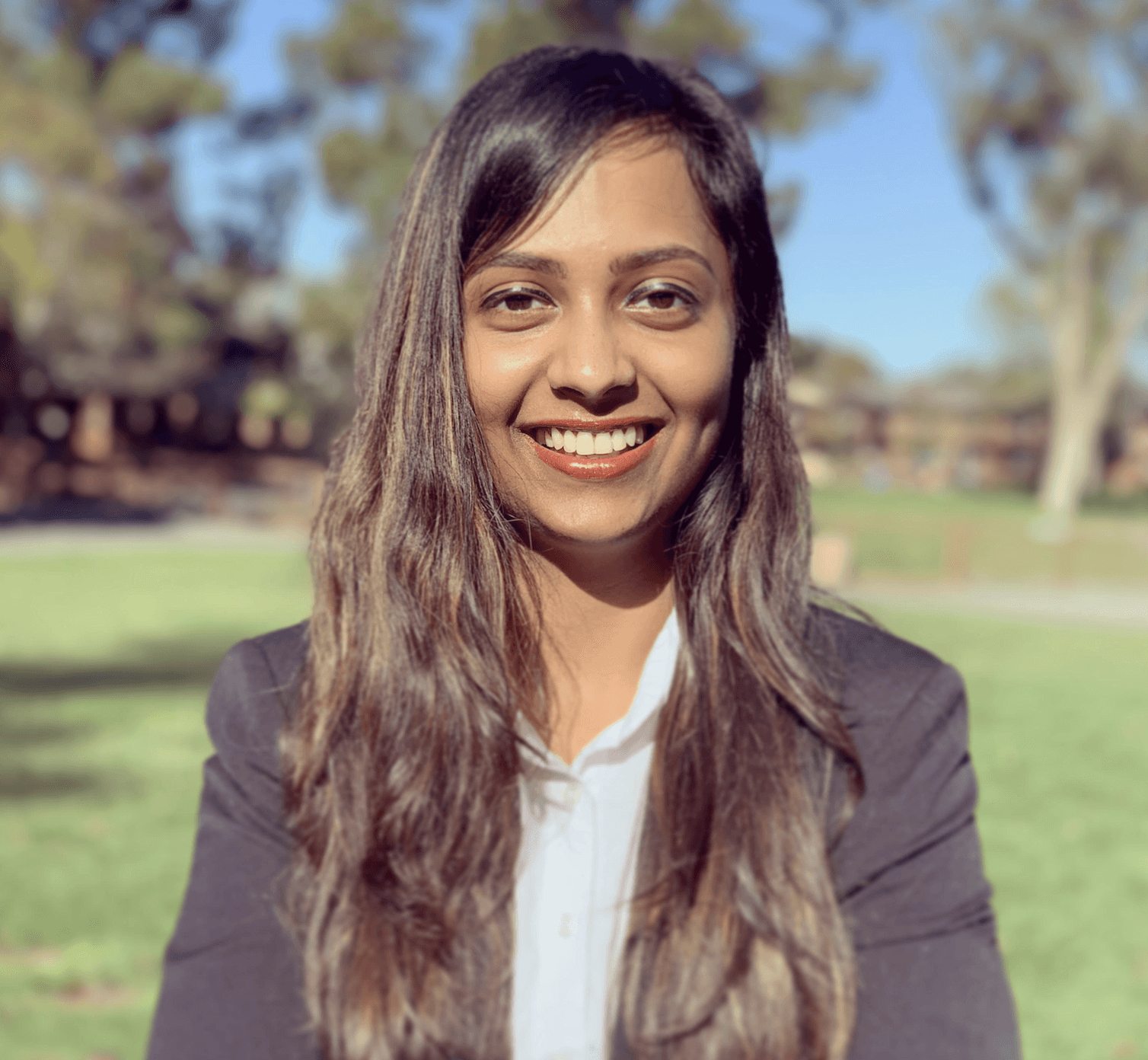
Ankita Gupta
Mar 9, 2024
File upload features are common in web applications but can introduce significant security risks if not properly handled. Attackers might exploit these features to upload malicious files, leading to unauthorized access, data breaches, or other security incidents. Reviewing and securing file upload mechanisms is essential to protect your application and its users.
Real-Life Scenario:
Consider a PHP script designed to handle file uploads:
In this example, the script uploads a file from the user to a specified directory without performing extensive checks on the file.
Engaging GitHub Copilot:
Prompt to GitHub Copilot: "Review this file upload feature for security vulnerabilities."
Anticipated Copilot Analysis:
GitHub Copilot would scrutinize the file upload mechanism and might respond with:
Incorporating these changes will significantly enhance the security of the file upload feature by validating the file type and size, sanitizing file names, and ensuring safe storage practices.
Common pitfalls when checking secure file uploads using GitHub Copilot include:
Overreliance on AI: While GitHub Copilot can provide helpful suggestions, it's important to remember that it's an AI tool and not a replacement for a seasoned developer's judgement. Always double-check the code it generates and use it as a starting point rather than a final solution.
Limited Context Understanding: GitHub Copilot may not fully grasp the context of your project and may overlook certain security considerations that are unique to your application. It's important to review the provided code in the context of your specific application and security requirements.
Lack of Updates on Security Practices: Security practices evolve over time, and GitHub Copilot may not always suggest the most up-to-date security practices. Always consult the latest security guidelines and best practices to ensure your file upload feature is secure.
Explore more from Akto
Blog
Be updated about everything related to API Security, new API vulnerabilities, industry news and product updates.
Events
Browse and register for upcoming sessions or catch up on what you missed with exclusive recordings
CVE Database
Find out everything about latest API CVE in popular products
Test Library
Discover and find tests from Akto's 100+ API Security test library. Choose your template or add a new template to start your API Security testing.
Documentation
Check out Akto's product documentation for all information related to features and how to use them.