Date Regex Go Validator
Go
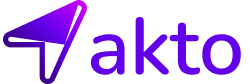
Show Your Support with a Star ⭐
It takes just a second, but it means the world to us.
Introduction to Date Regex
Date validation is essential in many applications to ensure the correct format and logical values. A regex pattern for a standard date format like YYYY-MM-DD
can be ^\\d{4}-\\d{2}-\\d{2}$
.
What is Date Regex?
The regex for date validation should match the specific format required by the application.
The Date Regex Pattern
For YYYY-MM-DD
format: ^\\d{4}-\\d{2}-\\d{2}$
. This pattern ensures that the date consists of four digits for the year, two for the month, and two for the day, separated by hyphens.
How to Validate Dates Using Regex in Go?
Validating dates in Go using regex:
Define the regex pattern for the specific date format.
Compile the regex using the
regexp
package in Go.Validate the date strings with the compiled regex.
package main
Uses of Date Regex Validation
Form Input Validation: Ensuring users enter dates in the correct format in web forms and applications.
Data Parsing and Processing: Standardizing date formats in data processing, especially when dealing with different regional formats.
Event and Schedule Management: Validating date inputs in applications related to event planning, scheduling, and reminders.
What next?
For effective date validation in Go, the described regex pattern provides a good method for ensuring that date inputs conform to the standard YYYY-MM-DD
format. In addition to regex validation, utilizing a tool like Akto's Date Validator can offer further assurance by checking for logical correctness of dates, such as correct month and day values, leap years, and more, thus enhancing the overall data integrity in your application.