
File convertors
DNS tools
Encoders & Decoders
Hash generators
Calculators
Generators
Regex testers
URL Regex Java Validator
Password Regex Java Validator
SSN Regex Java Validator
Mac Address Regex Java Validator
UUID Regex Java Validator
Credit Card Regex Java Validator
GUID Regex Java Validator
IP Address Regex Java Validator
Date Regex Java Validator
Email Regex Javascript Validator
Phone Number Regex Javascript Validator
Numbers Regex Javascript Validator
URL Regex Javascript Validator
Password Regex Javascript Validator
SSN Regex Javascript Validator
Mac Address Regex Javascript Validator
UUID Regex Javascript Validator
Credit Card Regex Javascript Validator
GUID Regex Javascript Validator
IP Address Regex Javascript Validator
Date Regex Javascript Validator
Email Regex Python Validator
Phone Number Regex Python Validator
Numbers Regex Python Validator
URL Regex Python Validator
Password Regex Python Validator
SSN Regex Python Validator
Mac Address Regex Python Validator
UUID Regex Python Validator
Credit Card Regex Python Validator
GUID Regex Python Validator
IP Address Regex Python Validator
Date Regex Python Validator
RegEx Tester
Go RegEx Tester
Javascript RegEx Tester
Java RegEx Tester
Email Regex Go Validator
Phone Number Regex Go Validator
Numbers Regex Go Validator
URL Regex Go Validator
Password Regex Validator
SSN Regex Go Validator
Mac Address Regex Go Validator
UUID Regex Go Validator
Credit Card Regex Go Validator
GUID Regex Go Validator
IP Address Regex Go Validator
Date Regex Go Validator
Email Regex Java Validator
Phone Number Regex Java Validator
Numbers Regex Java Validator
URL Regex Java Validator
Learn how to validate URLs in Java using regular expressions. This guide includes a URL regex pattern and a simple Java method for URL validation. Useful for web applications and data validation.
Learn how to validate URLs in Java using regular expressions. This guide includes a URL regex pattern and a simple Java method for URL validation. Useful for web applications and data validation.
Learn how to validate URLs in Java using regular expressions. This guide includes a URL regex pattern and a simple Java method for URL validation. Useful for web applications and data validation.
Java
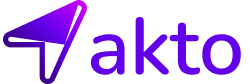
Show Your Support with a Star ⭐
It takes just a second, but it means the world to us.

Regular Expression - Documentation
Regular Expression - Documentation
Introduction
In Java, URL validation is commonly required in web applications and data processing. The java.util.regex
package in Java provides the functionality for regex-based URL validation.
URL Regex
Java regex for URL validation typically includes the protocol, domain, and optional path.
The URL Regex Pattern
Pattern:
https?://(?:www\\.)?[a-zA-Z0-9./]+
This regex pattern supports HTTP and HTTPS and optional 'www.'.
How to Validate URLs in Java?
To perform URL validation in Java:
import java.util.regex.*;
public class URLValidator {
public static boolean isValidURL(String url) {
String regex = "https?://(?:www\\.)?[a-zA-Z0-9./]+";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(url);
return matcher.matches();
}
public static void main(String[] args) {
String url = "https://www.example.com";
System.out.println("Is URL valid? " + isValidURL(url));
}
}
Uses of URL Regex Validation
Web Applications: Validating URLs in user input, such as form submissions or URL parameters.
Data Validation: Ensuring the correctness of URLs in datasets, crucial for data integrity.
What next?
Java's robust regex capabilities facilitate effective URL validation, ensuring correctness in web applications and data processing. For comprehensive validation, including advanced URL formats, Akto's regex validator is a valuable resource.
Frequently asked questions
Why is URL validation important in web applications?
URL validation is important in web applications to ensure that user-inputted URLs are correctly formatted and safe to use. It helps prevent potential security vulnerabilities and enhances the overall user experience.
Why is URL validation important in web applications?
URL validation is important in web applications to ensure that user-inputted URLs are correctly formatted and safe to use. It helps prevent potential security vulnerabilities and enhances the overall user experience.
What does the provided URL regex pattern support?
The provided URL regex pattern supports HTTP and HTTPS URLs, with an optional 'www.' subdomain. It validates the protocol, domain, and optional path of a URL.
What does the provided URL regex pattern support?
The provided URL regex pattern supports HTTP and HTTPS URLs, with an optional 'www.' subdomain. It validates the protocol, domain, and optional path of a URL.
Can I use the URL validation regex pattern for other programming languages?
The URL validation regex pattern provided in this document is specifically for Java. However, you can adapt the concept and use similar regex patterns in other programming languages that support regular expressions.
Can I use the URL validation regex pattern for other programming languages?
The URL validation regex pattern provided in this document is specifically for Java. However, you can adapt the concept and use similar regex patterns in other programming languages that support regular expressions.
Are there any additional resources for advanced URL validation?
For comprehensive validation, including advanced URL formats, you can explore Akto's regex validator. It provides a wide range of regex patterns for various purposes, including URL validation.
Are there any additional resources for advanced URL validation?
For comprehensive validation, including advanced URL formats, you can explore Akto's regex validator. It provides a wide range of regex patterns for various purposes, including URL validation.
What are the uses of URL regex validation in data processing?
URL regex validation is crucial in data processing to ensure the correctness of URLs in datasets. It helps maintain data integrity and reliability by identifying and filtering out invalid or malformed URLs.
What are the uses of URL regex validation in data processing?
URL regex validation is crucial in data processing to ensure the correctness of URLs in datasets. It helps maintain data integrity and reliability by identifying and filtering out invalid or malformed URLs.
Want to ask something?
Our community offers a network of support and resources. You can ask any question there and will get a reply in 24 hours.
Want to ask something?
Our community offers a network of support and resources. You can ask any question there and will get a reply in 24 hours.
Want to ask something?
Our community offers a network of support and resources. You can ask any question there and will get a reply in 24 hours.

File convertors
DNS tools
Encoders & Decoders
Hash generators
Calculators
Generators
Regex testers
URL Regex Java Validator
Password Regex Java Validator
SSN Regex Java Validator
Mac Address Regex Java Validator
UUID Regex Java Validator
Credit Card Regex Java Validator
GUID Regex Java Validator
IP Address Regex Java Validator
Date Regex Java Validator
Email Regex Javascript Validator
Phone Number Regex Javascript Validator
Numbers Regex Javascript Validator
URL Regex Javascript Validator
Password Regex Javascript Validator
SSN Regex Javascript Validator
Mac Address Regex Javascript Validator
UUID Regex Javascript Validator
Credit Card Regex Javascript Validator
GUID Regex Javascript Validator
IP Address Regex Javascript Validator
Date Regex Javascript Validator
Email Regex Python Validator
Phone Number Regex Python Validator
Numbers Regex Python Validator
URL Regex Python Validator
Password Regex Python Validator
SSN Regex Python Validator
Mac Address Regex Python Validator
UUID Regex Python Validator
Credit Card Regex Python Validator
GUID Regex Python Validator
IP Address Regex Python Validator
Date Regex Python Validator
RegEx Tester
Go RegEx Tester
Javascript RegEx Tester
Java RegEx Tester
Email Regex Go Validator
Phone Number Regex Go Validator
Numbers Regex Go Validator
URL Regex Go Validator
Password Regex Validator
SSN Regex Go Validator
Mac Address Regex Go Validator
UUID Regex Go Validator
Credit Card Regex Go Validator
GUID Regex Go Validator
IP Address Regex Go Validator
Date Regex Go Validator
Email Regex Java Validator
Phone Number Regex Java Validator
Numbers Regex Java Validator

File convertors
DNS tools
Encoders & Decoders
Hash generators
Calculators
Generators
Regex testers
URL Regex Java Validator
Password Regex Java Validator
SSN Regex Java Validator
Mac Address Regex Java Validator
UUID Regex Java Validator
Credit Card Regex Java Validator
GUID Regex Java Validator
IP Address Regex Java Validator
Date Regex Java Validator
Email Regex Javascript Validator
Phone Number Regex Javascript Validator
Numbers Regex Javascript Validator
URL Regex Javascript Validator
Password Regex Javascript Validator
SSN Regex Javascript Validator
Mac Address Regex Javascript Validator
UUID Regex Javascript Validator
Credit Card Regex Javascript Validator
GUID Regex Javascript Validator
IP Address Regex Javascript Validator
Date Regex Javascript Validator
Email Regex Python Validator
Phone Number Regex Python Validator
Numbers Regex Python Validator
URL Regex Python Validator
Password Regex Python Validator
SSN Regex Python Validator
Mac Address Regex Python Validator
UUID Regex Python Validator
Credit Card Regex Python Validator
GUID Regex Python Validator
IP Address Regex Python Validator
Date Regex Python Validator
RegEx Tester
Go RegEx Tester
Javascript RegEx Tester
Java RegEx Tester
Email Regex Go Validator
Phone Number Regex Go Validator
Numbers Regex Go Validator
URL Regex Go Validator
Password Regex Validator
SSN Regex Go Validator
Mac Address Regex Go Validator
UUID Regex Go Validator
Credit Card Regex Go Validator
GUID Regex Go Validator
IP Address Regex Go Validator
Date Regex Go Validator
Email Regex Java Validator
Phone Number Regex Java Validator
Numbers Regex Java Validator