Email Regex Java Validator
Java
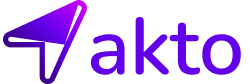
Show Your Support with a Star ⭐
It takes just a second, but it means the world to us.
Introduction to Email Regex Java
In Java, email validation is commonly implemented in various applications, from user data processing to form validation. Java's strong typing and robust standard libraries, including regex support, make it well-suited for accurately validating email addresses. A standard regex pattern for email validation in Java might be ^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\\\\.[a-zA-Z]{2,}$
.
What is Email Regex?
Email addresses generally consist of a local part, a domain, and a top-level domain (TLD).
The Local Part
The local part appears before the '@' and includes alphanumeric and special characters.
Pattern:
[a-zA-Z0-9._%+-]+
The Domain
The domain follows the '@' symbol and typically includes alphanumeric characters and hyphens.
Pattern:
[a-zA-Z0-9.-]+
The Top-Level Domain (TLD)
The TLD comes after the last period and is composed of alphabetic characters.
Pattern:
[a-zA-Z]{2,}
Complete Email Regex Pattern
Combining these elements, the complete regex for email validation in Java is:
How to Validate Email Regex in Java?
To validate an email address in Java:
Define the regex pattern.
Use
Pattern
andMatcher
classes fromjava.util.regex
package.Validate the email string.
Uses of Email Regex Java Validation
User Data Processing: Validating email addresses in user registration forms, login pages, and customer databases.
Data Cleaning and Standardization: Ensuring consistency and validity of email addresses in data sets, especially in large-scale applications.
Conclusion
For effective email validation in Java applications, regex provides a flexible and powerful mechanism. To test your emails in Java, use Akto’s Java email validator.
Check our other language Email regex validators - Email Python Regex, Email Golang Regex, Email Java script Regex