Email Regex Go Validator
Go
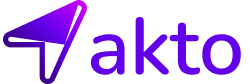
Show Your Support with a Star ⭐
It takes just a second, but it means the world to us.
Introduction Email Regex Go
Regular expressions are patterns used to match character combinations in strings. In the context of email validation, a regex is designed to match most patterns of valid email addresses as defined by internet standards. A basic email regex might look like this: ^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\\.[a-zA-Z]{2,}$
. This regex checks for a general structure: local-part@domain
.
What is Email regex?
An email address typically consists of three key parts: the local part, the domain, and the top-level domain (TLD).
1. The Local Part
The local part of the email address is what comes before the '@' symbol. It can include:
Alphanumeric characters: both uppercase and lowercase (
a-z
,A-Z
,0-9
).Special characters: such as period (
.
), underscore (_
), percent (%
), plus (+
), and hyphen (``).
The regex pattern for the local part is: [a-zA-Z0-9._%+-]+
2. The Domain
Following the local part is the domain, which comes after the '@' symbol and before the TLD. The domain can include:
Alphanumeric characters (
a-z
,A-Z
,0-9
).Hyphens (``) and periods (
.
) for domain separation.
The regex pattern for the domain is: [a-zA-Z0-9.-]+
3. The Top-Level Domain (TLD)
The TLD is the final part of the email address, following the last period. It is crucial for specifying the email's domain category. The TLD:
Consists of alphabetic characters only (
a-z
,A-Z
).Must be at least two characters long to accommodate standard TLDs like
.com
,.org
, etc.
The regex pattern for the TLD is: [a-zA-Z]{2,}
The Complete Email Regex Pattern
Combining all these parts, the complete basic regex pattern for an email address is:
^
and$
are anchors that ensure the entire string matches the pattern from start to end.The
+
quantifier after the character sets ensures that one or more of the specified characters are present in the respective part of the email address.
How to validate email regex in Go?
Validating an email using regex in Go involves a few steps:
Define the regex pattern for a valid email.
Use the
regexp
package in Go to compile the regex pattern.Use the compiled regex to validate email strings.
Extensive email regex
While the basic regex works for many cases, the RFC 5322 standard for email addresses is more complex. An extensive regex pattern that conforms more closely to this standard can be quite lengthy and intricate.
Key Considerations for RFC 5322 Compliance
Quoted Strings: The local part of an email address can include quoted strings, allowing for a wider range of characters, including spaces and special characters. For instance,
"john.doe"@example.com
is a valid format under RFC 5322.Comments and Folding Whitespace: RFC 5322 allows for comments in email addresses enclosed in parentheses, potentially interspersed with folding whitespace (spaces, tabs, and line breaks). These elements are typically ignored for the purpose of email routing.
Special Characters: The standard permits a variety of special characters in certain conditions, such as
!
,#
,$
,%
,&
,'
, ``,+
,/
,=
,?
,^
,_
, ```,{
,|
,}
,~
, and more.Domain Literal: Email addresses can have a domain literal rather than a domain name, like
user@[192.168.1.1]
. This requires the regex to account for IPv4 and potentially IPv6 addresses.
Given these considerations, an extensive regex pattern that attempts to adhere to RFC 5322 will be quite complex. It might look something like this (simplified for readability):
While such an extensive regex provides a higher degree of compliance with RFC 5322, its complexity might be overkill for many applications.
Uses of Email Regex Validation
Email regex validation is essential in various sectors of software development and data management, particularly for:
User Registration and Authentication: It ensures users provide valid email addresses during sign-ups, identity verification and communication.
Data Cleaning and Standardization: Regex validation helps maintain clean and standardized email databases for effective communication and marketing strategies.
Form Validation: It's used in web forms to prevent incorrect email formats, enhancing data quality and user experience.
What next?
To test and validate the effectiveness of their email regex patterns, Check out Akto’s email validator for different languages.