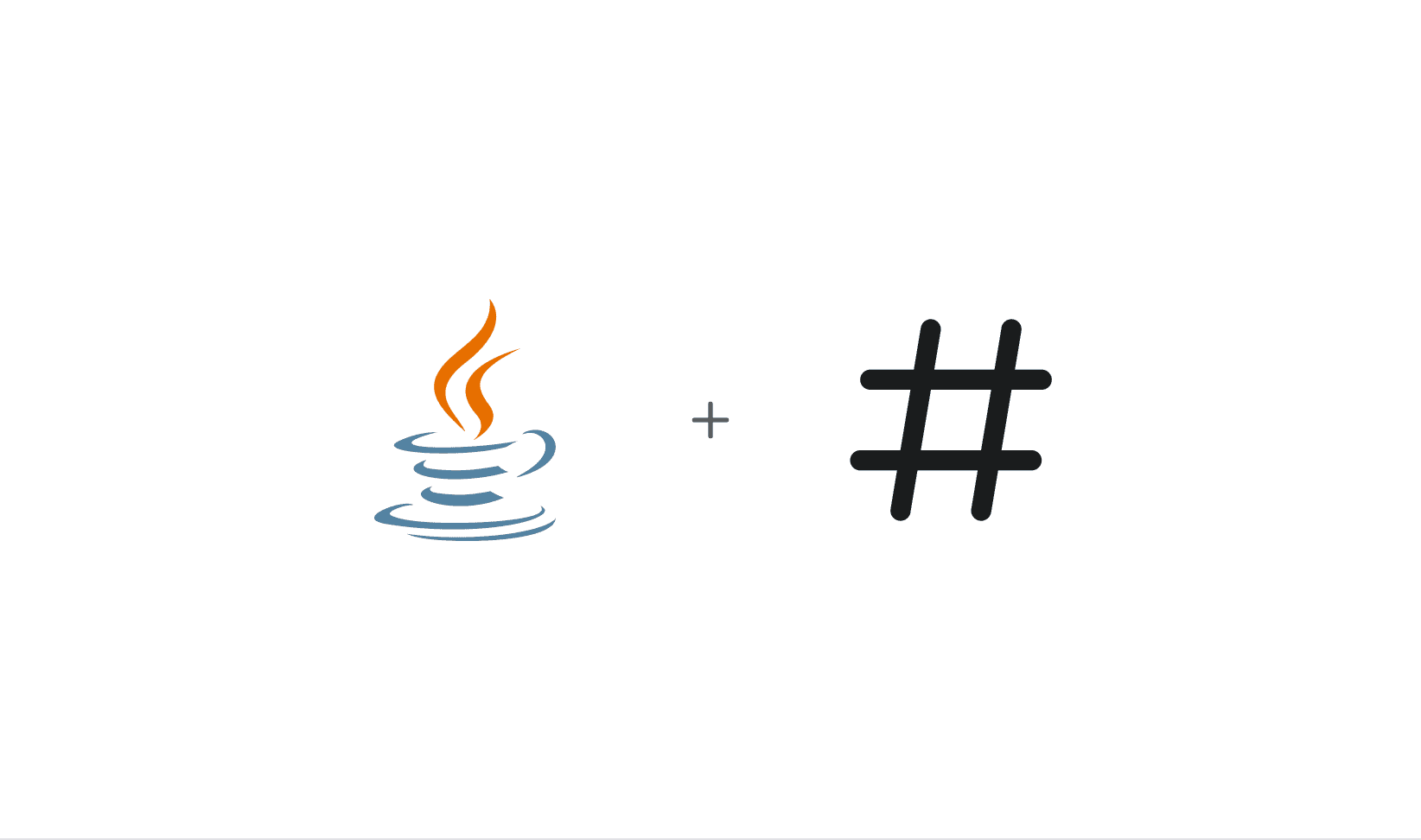
File convertors
DNS tools
Encoders & Decoders
Hash generators
Calculators
Generators
Regex testers
Numbers Regex Java Validator
URL Regex Java Validator
Password Regex Java Validator
SSN Regex Java Validator
Mac Address Regex Java Validator
UUID Regex Java Validator
Credit Card Regex Java Validator
GUID Regex Java Validator
IP Address Regex Java Validator
Date Regex Java Validator
Email Regex Javascript Validator
Phone Number Regex Javascript Validator
Numbers Regex Javascript Validator
URL Regex Javascript Validator
Password Regex Javascript Validator
SSN Regex Javascript Validator
Mac Address Regex Javascript Validator
UUID Regex Javascript Validator
Credit Card Regex Javascript Validator
GUID Regex Javascript Validator
IP Address Regex Javascript Validator
Date Regex Javascript Validator
Email Regex Python Validator
Phone Number Regex Python Validator
Numbers Regex Python Validator
URL Regex Python Validator
Password Regex Python Validator
SSN Regex Python Validator
Mac Address Regex Python Validator
UUID Regex Python Validator
Credit Card Regex Python Validator
GUID Regex Python Validator
IP Address Regex Python Validator
Date Regex Python Validator
RegEx Tester
Go RegEx Tester
Javascript RegEx Tester
Java RegEx Tester
Email Regex Go Validator
Phone Number Regex Go Validator
Numbers Regex Go Validator
URL Regex Go Validator
Password Regex Validator
SSN Regex Go Validator
Mac Address Regex Go Validator
UUID Regex Go Validator
Credit Card Regex Go Validator
GUID Regex Go Validator
IP Address Regex Go Validator
Date Regex Go Validator
Email Regex Java Validator
Phone Number Regex Java Validator
Numbers Regex Java Validator
Learn and test to validate numbers in Java using regex patterns. Useful for applications dealing with financial data, user input, or data processing.
Learn and test to validate numbers in Java using regex patterns. Useful for applications dealing with financial data, user input, or data processing.
Learn and test to validate numbers in Java using regex patterns. Useful for applications dealing with financial data, user input, or data processing.
Java
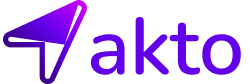
Show Your Support with a Star ⭐
It takes just a second, but it means the world to us.

Regular Expression - Documentation
Regular Expression - Documentation
Introduction
In Java, number validation is a common task, particularly in applications dealing with financial data, user input, or data processing. Java's java.util.regex
package allows for efficient number validation using regex. A simple regex for integer validation might be ^\\d+$
.
What is Number Regex?
The regex pattern for number validation in Java varies based on the type of number (integer, decimal, etc.).
The Number Regex Pattern
Integers:
^\\d+$
Decimals:
^\\d+\\.\\d+$
How to Validate Numbers in Java?
Using the Pattern
and Matcher
classes in Java for number validation:
import java.util.regex.*;
public class PhoneNumberValidator {
public static boolean isValidPhoneNumber(String phoneNumber) {
String regex = "^\\+[1-9]\\d{1,14}$";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(phoneNumber);
return matcher.matches();
}
public static void main(String[] args) {
String phoneNumber = "+12345678901";
System.out.println("Is the phone number valid? " + isValidPhoneNumber(phoneNumber));
}
}
Uses of Number Regex Validation
Input Validation: Ensuring numerical inputs in forms and data fields are correctly formatted.
Data Integrity: Standardizing and validating numbers in datasets and databases.
What next?
Java provides robust tools for regex-based number validation, essential for ensuring data accuracy. For diverse and complex number formats, Akto's regex validator offers an extended solution for regex validation needs.
Frequently asked questions
Why is number validation important in Java applications?
Number validation ensures data integrity and accurate processing, especially in financial applications and user input scenarios.
Why is number validation important in Java applications?
Number validation ensures data integrity and accurate processing, especially in financial applications and user input scenarios.
How can I validate an integer in Java using regex?
You can validate an integer in Java using the regex pattern ^\\d+$, which matches one or more digits.
How can I validate an integer in Java using regex?
You can validate an integer in Java using the regex pattern ^\\d+$, which matches one or more digits.
What is the regex pattern for validating decimal numbers in Java?
The regex pattern for validating decimal numbers in Java is ^\\d+\\.\\d+$, which matches one or more digits before and after the decimal point.
What is the regex pattern for validating decimal numbers in Java?
The regex pattern for validating decimal numbers in Java is ^\\d+\\.\\d+$, which matches one or more digits before and after the decimal point.
Can I modify the regex pattern for different types of numbers in Java?
Yes, you can modify the regex pattern to suit different types of numbers by adjusting the regex pattern in the isValidNumber method.
Can I modify the regex pattern for different types of numbers in Java?
Yes, you can modify the regex pattern to suit different types of numbers by adjusting the regex pattern in the isValidNumber method.
What are the uses of number regex validation in Java?
Number regex validation is commonly used for input validation in forms, ensuring correct formatting of numerical data, and maintaining data integrity in datasets and databases.
What are the uses of number regex validation in Java?
Number regex validation is commonly used for input validation in forms, ensuring correct formatting of numerical data, and maintaining data integrity in datasets and databases.
Want to ask something?
Our community offers a network of support and resources. You can ask any question there and will get a reply in 24 hours.
Want to ask something?
Our community offers a network of support and resources. You can ask any question there and will get a reply in 24 hours.
Want to ask something?
Our community offers a network of support and resources. You can ask any question there and will get a reply in 24 hours.
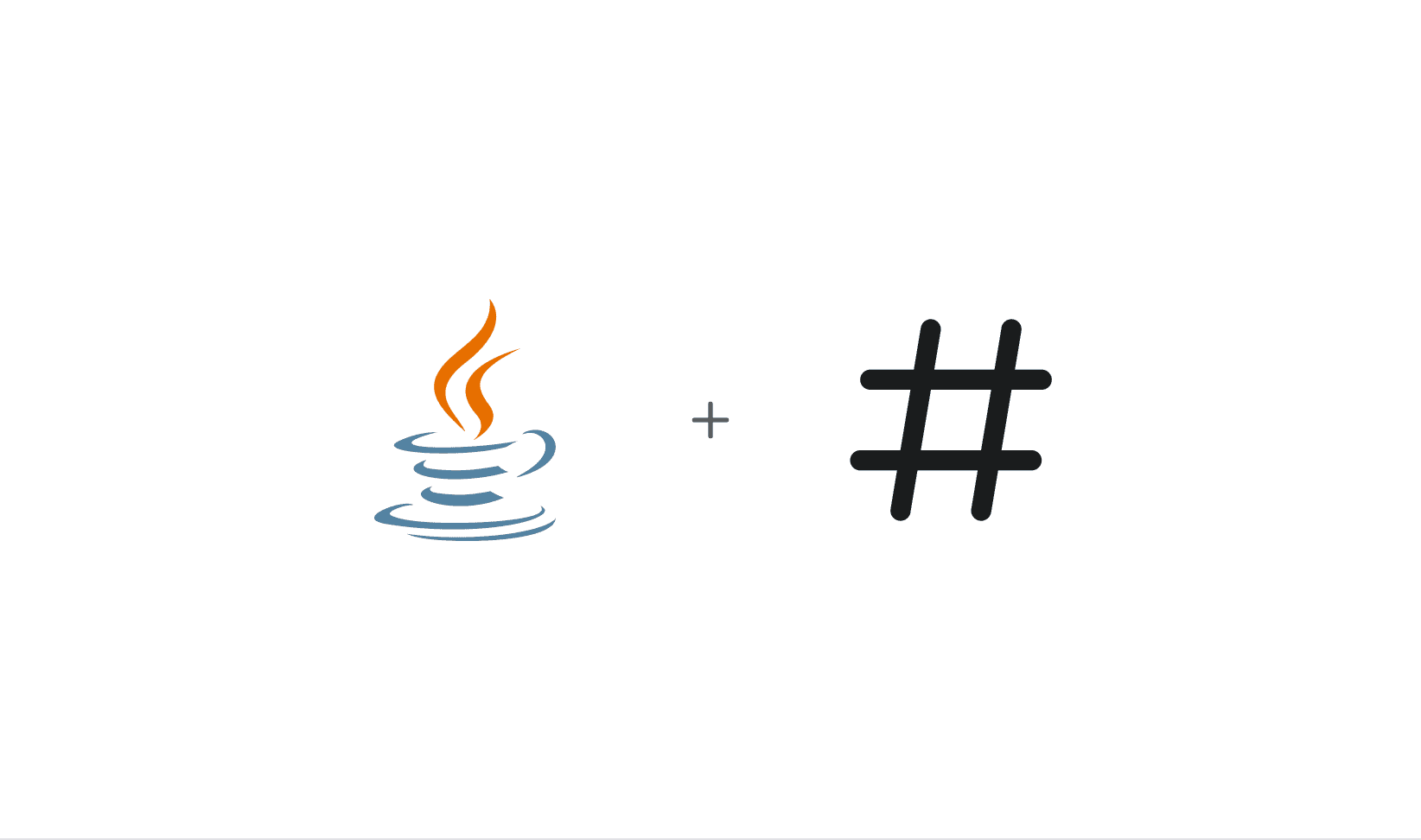
File convertors
DNS tools
Encoders & Decoders
Hash generators
Calculators
Generators
Regex testers
Numbers Regex Java Validator
URL Regex Java Validator
Password Regex Java Validator
SSN Regex Java Validator
Mac Address Regex Java Validator
UUID Regex Java Validator
Credit Card Regex Java Validator
GUID Regex Java Validator
IP Address Regex Java Validator
Date Regex Java Validator
Email Regex Javascript Validator
Phone Number Regex Javascript Validator
Numbers Regex Javascript Validator
URL Regex Javascript Validator
Password Regex Javascript Validator
SSN Regex Javascript Validator
Mac Address Regex Javascript Validator
UUID Regex Javascript Validator
Credit Card Regex Javascript Validator
GUID Regex Javascript Validator
IP Address Regex Javascript Validator
Date Regex Javascript Validator
Email Regex Python Validator
Phone Number Regex Python Validator
Numbers Regex Python Validator
URL Regex Python Validator
Password Regex Python Validator
SSN Regex Python Validator
Mac Address Regex Python Validator
UUID Regex Python Validator
Credit Card Regex Python Validator
GUID Regex Python Validator
IP Address Regex Python Validator
Date Regex Python Validator
RegEx Tester
Go RegEx Tester
Javascript RegEx Tester
Java RegEx Tester
Email Regex Go Validator
Phone Number Regex Go Validator
Numbers Regex Go Validator
URL Regex Go Validator
Password Regex Validator
SSN Regex Go Validator
Mac Address Regex Go Validator
UUID Regex Go Validator
Credit Card Regex Go Validator
GUID Regex Go Validator
IP Address Regex Go Validator
Date Regex Go Validator
Email Regex Java Validator
Phone Number Regex Java Validator
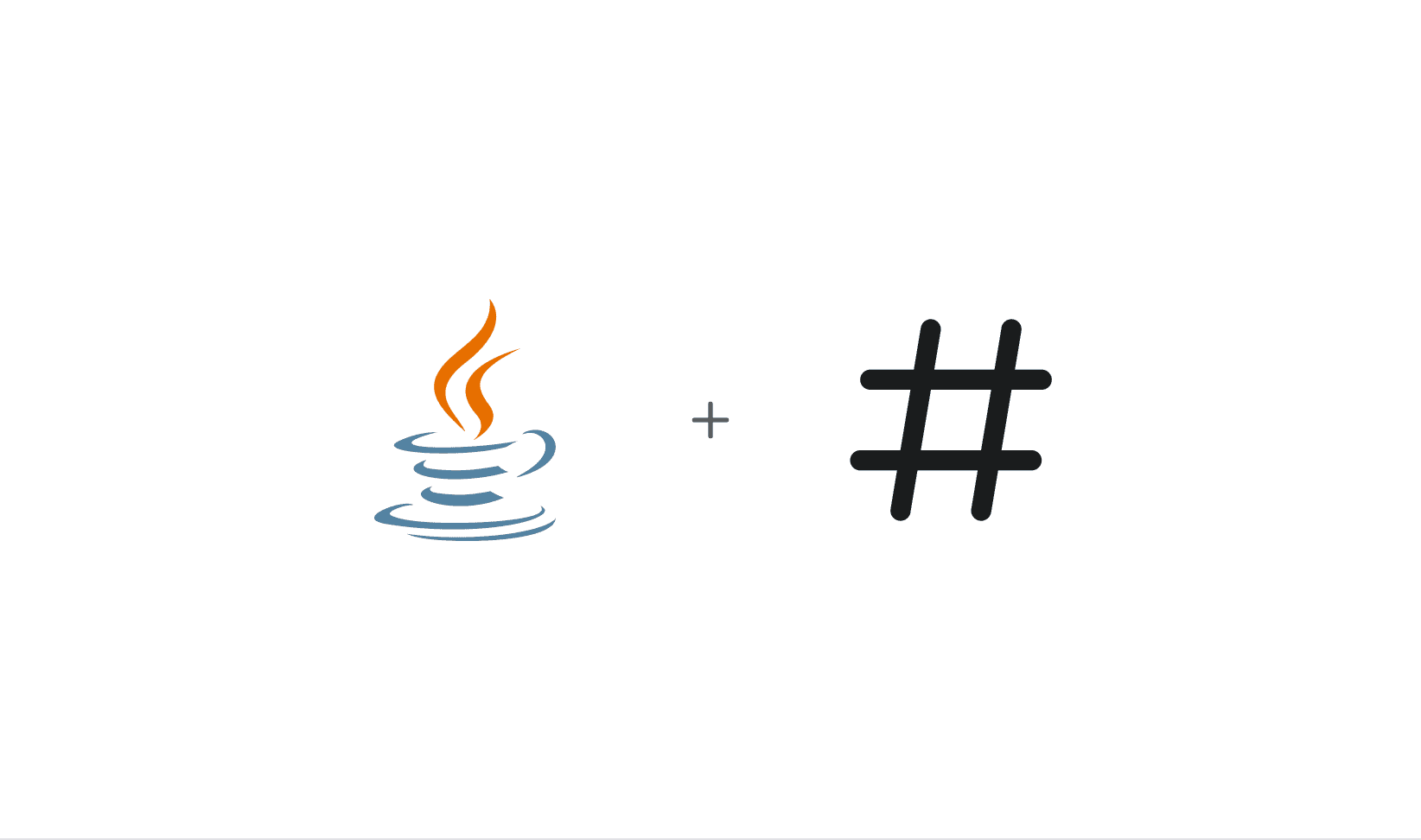
File convertors
DNS tools
Encoders & Decoders
Hash generators
Calculators
Generators
Regex testers
Numbers Regex Java Validator
URL Regex Java Validator
Password Regex Java Validator
SSN Regex Java Validator
Mac Address Regex Java Validator
UUID Regex Java Validator
Credit Card Regex Java Validator
GUID Regex Java Validator
IP Address Regex Java Validator
Date Regex Java Validator
Email Regex Javascript Validator
Phone Number Regex Javascript Validator
Numbers Regex Javascript Validator
URL Regex Javascript Validator
Password Regex Javascript Validator
SSN Regex Javascript Validator
Mac Address Regex Javascript Validator
UUID Regex Javascript Validator
Credit Card Regex Javascript Validator
GUID Regex Javascript Validator
IP Address Regex Javascript Validator
Date Regex Javascript Validator
Email Regex Python Validator
Phone Number Regex Python Validator
Numbers Regex Python Validator
URL Regex Python Validator
Password Regex Python Validator
SSN Regex Python Validator
Mac Address Regex Python Validator
UUID Regex Python Validator
Credit Card Regex Python Validator
GUID Regex Python Validator
IP Address Regex Python Validator
Date Regex Python Validator
RegEx Tester
Go RegEx Tester
Javascript RegEx Tester
Java RegEx Tester
Email Regex Go Validator
Phone Number Regex Go Validator
Numbers Regex Go Validator
URL Regex Go Validator
Password Regex Validator
SSN Regex Go Validator
Mac Address Regex Go Validator
UUID Regex Go Validator
Credit Card Regex Go Validator
GUID Regex Go Validator
IP Address Regex Go Validator
Date Regex Go Validator
Email Regex Java Validator
Phone Number Regex Java Validator