Password Regex Python Validator
Python
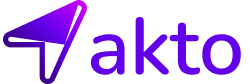
Show Your Support with a Star ⭐
It takes just a second, but it means the world to us.
Introduction Password Regex Python
In Python, password validation is often required to ensure security in applications. The re
module in Python can be used to create regex patterns that check for specific criteria in passwords, such as minimum length, inclusion of uppercase and lowercase characters, digits, and special characters. A common regex pattern for strong password validation might be ^(?=.*[a-z])(?=.*[A-Z])(?=.*\\d)(?=.*[@$!%*?&])[A-Za-z\\d@$!%*?&]{8,}$
.
Password Regex
A robust password regex pattern in Python typically includes checks for various character types and length.
The Password Regex Pattern
Pattern: ^(?=.*[a-z])(?=.*[A-Z])(?=.*\\d)(?=.*[@$!%*?&])[A-Za-z\\d@$!%*?&]{8,}$
This ensures passwords have mixed characters and a minimum length of 8.
How to Validate Passwords in Python?
To validate passwords using regex in Python:
Uses of Password Regex Validation
User Authentication: Enforcing strong passwords in user sign-up and login processes.
Security Compliance: Meeting security standards that require complex and strong passwords.
What next?
Python's regex functionality is effective for implementing strong password validation policies. For additional complexity and varied password rules, Akto's regex validator is a useful tool for testing and enhancing password validation strategies.