
File convertors
DNS tools
Encoders & Decoders
Hash generators
Calculators
Generators
Regex testers
Password Regex Java Validator
SSN Regex Java Validator
Mac Address Regex Java Validator
UUID Regex Java Validator
Credit Card Regex Java Validator
GUID Regex Java Validator
IP Address Regex Java Validator
Date Regex Java Validator
Email Regex Javascript Validator
Phone Number Regex Javascript Validator
Numbers Regex Javascript Validator
URL Regex Javascript Validator
Password Regex Javascript Validator
SSN Regex Javascript Validator
Mac Address Regex Javascript Validator
UUID Regex Javascript Validator
Credit Card Regex Javascript Validator
GUID Regex Javascript Validator
IP Address Regex Javascript Validator
Date Regex Javascript Validator
Email Regex Python Validator
Phone Number Regex Python Validator
Numbers Regex Python Validator
URL Regex Python Validator
Password Regex Python Validator
SSN Regex Python Validator
Mac Address Regex Python Validator
UUID Regex Python Validator
Credit Card Regex Python Validator
GUID Regex Python Validator
IP Address Regex Python Validator
Date Regex Python Validator
RegEx Tester
Go RegEx Tester
Javascript RegEx Tester
Java RegEx Tester
Email Regex Go Validator
Phone Number Regex Go Validator
Numbers Regex Go Validator
URL Regex Go Validator
Password Regex Validator
SSN Regex Go Validator
Mac Address Regex Go Validator
UUID Regex Go Validator
Credit Card Regex Go Validator
GUID Regex Go Validator
IP Address Regex Go Validator
Date Regex Go Validator
Email Regex Java Validator
Phone Number Regex Java Validator
Numbers Regex Java Validator
URL Regex Java Validator
Password Regex Java Validator
Discover how to ensure application security with Java password validation. This page provides insights into creating regex patterns for password validation and implementing them in Java. Learn to enhance security and meet compliance standards.
Discover how to ensure application security with Java password validation. This page provides insights into creating regex patterns for password validation and implementing them in Java. Learn to enhance security and meet compliance standards.
Discover how to ensure application security with Java password validation. This page provides insights into creating regex patterns for password validation and implementing them in Java. Learn to enhance security and meet compliance standards.
Java
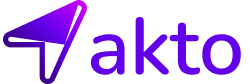
Show Your Support with a Star ⭐
It takes just a second, but it means the world to us.

Regular Expression - Documentation
Regular Expression - Documentation
Introduction
Validating passwords in Java is a crucial part of ensuring application security, especially in user management systems. Java's java.util.regex
package provides the means to create regex patterns for password validation, ensuring they meet specified security criteria.
Password Regex
A password regex in Java checks for a combination of uppercase, lowercase, digits, special characters, and length.
The Password Regex Pattern
Pattern:
^(?=.*[a-z])(?=.*[A-Z])(?=.*\\d)(?=.*[@$!%*?&])[A-Za-z\\d@$!%*?&]{8,}$
This pattern enforces a mix of character types and a minimum length.
How to Validate Passwords in Java?
In Java, password validation can be implemented as follows:
import java.util.regex.*;
public class PasswordValidator {
public static boolean isValidPassword(String password) {
String regex = "^(?=.*[a-z])(?=.*[A-Z])(?=.*\\d)(?=.*[@$!%*?&])[A-Za-z\\d@$!%*?&]{8,}$";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(password);
return matcher.matches();
}
public static void main(String[] args) {
String password = "Example123!";
System.out.println("Is password valid? " + isValidPassword(password));
}
}
Uses of Password Regex Validation
Enhancing Security: Ensuring that passwords used in the system are strong and resistant to common attack methods.
Regulatory Compliance: Adhering to industry standards and regulations that mandate complex passwords.
Conclusion
Java's capabilities for regex password validation play a key role in maintaining application security. For more nuanced password policies, Akto's regex validator offers an advanced solution for comprehensive password rule validation.
Frequently asked questions
Why is password validation important in Java?
Password validation in Java is crucial for ensuring application security, particularly in user management systems. It helps enforce strong password policies and reduces the risk of unauthorized access.
Why is password validation important in Java?
Password validation in Java is crucial for ensuring application security, particularly in user management systems. It helps enforce strong password policies and reduces the risk of unauthorized access.
What does the password regex pattern in Java check for?
The password regex pattern in Java checks for a combination of uppercase letters, lowercase letters, digits, special characters, and a minimum length. This ensures that passwords meet specified security criteria.
What does the password regex pattern in Java check for?
The password regex pattern in Java checks for a combination of uppercase letters, lowercase letters, digits, special characters, and a minimum length. This ensures that passwords meet specified security criteria.
How can I implement password validation in Java?
Password validation in Java can be implemented using the java.util.regex package. By creating a regex pattern and matching it against the password input, you can determine if the password meets the specified criteria.
How can I implement password validation in Java?
Password validation in Java can be implemented using the java.util.regex package. By creating a regex pattern and matching it against the password input, you can determine if the password meets the specified criteria.
What are the benefits of using password regex validation?
Using password regex validation enhances security by ensuring that passwords used in the system are strong and resistant to common attack methods. It also helps meet regulatory compliance requirements for complex passwords.
What are the benefits of using password regex validation?
Using password regex validation enhances security by ensuring that passwords used in the system are strong and resistant to common attack methods. It also helps meet regulatory compliance requirements for complex passwords.
Are there any advanced solutions for password rule validation in Java?
Yes, Akto's regex validator offers an advanced solution for comprehensive password rule validation. It provides more nuanced password policies and can be used to enforce specific password requirements beyond the standard regex pattern.
Are there any advanced solutions for password rule validation in Java?
Yes, Akto's regex validator offers an advanced solution for comprehensive password rule validation. It provides more nuanced password policies and can be used to enforce specific password requirements beyond the standard regex pattern.
Want to ask something?
Our community offers a network of support and resources. You can ask any question there and will get a reply in 24 hours.
Want to ask something?
Our community offers a network of support and resources. You can ask any question there and will get a reply in 24 hours.
Want to ask something?
Our community offers a network of support and resources. You can ask any question there and will get a reply in 24 hours.

File convertors
DNS tools
Encoders & Decoders
Hash generators
Calculators
Generators
Regex testers
Password Regex Java Validator
SSN Regex Java Validator
Mac Address Regex Java Validator
UUID Regex Java Validator
Credit Card Regex Java Validator
GUID Regex Java Validator
IP Address Regex Java Validator
Date Regex Java Validator
Email Regex Javascript Validator
Phone Number Regex Javascript Validator
Numbers Regex Javascript Validator
URL Regex Javascript Validator
Password Regex Javascript Validator
SSN Regex Javascript Validator
Mac Address Regex Javascript Validator
UUID Regex Javascript Validator
Credit Card Regex Javascript Validator
GUID Regex Javascript Validator
IP Address Regex Javascript Validator
Date Regex Javascript Validator
Email Regex Python Validator
Phone Number Regex Python Validator
Numbers Regex Python Validator
URL Regex Python Validator
Password Regex Python Validator
SSN Regex Python Validator
Mac Address Regex Python Validator
UUID Regex Python Validator
Credit Card Regex Python Validator
GUID Regex Python Validator
IP Address Regex Python Validator
Date Regex Python Validator
RegEx Tester
Go RegEx Tester
Javascript RegEx Tester
Java RegEx Tester
Email Regex Go Validator
Phone Number Regex Go Validator
Numbers Regex Go Validator
URL Regex Go Validator
Password Regex Validator
SSN Regex Go Validator
Mac Address Regex Go Validator
UUID Regex Go Validator
Credit Card Regex Go Validator
GUID Regex Go Validator
IP Address Regex Go Validator
Date Regex Go Validator
Email Regex Java Validator
Phone Number Regex Java Validator
Numbers Regex Java Validator
URL Regex Java Validator

File convertors
DNS tools
Encoders & Decoders
Hash generators
Calculators
Generators
Regex testers
Password Regex Java Validator
SSN Regex Java Validator
Mac Address Regex Java Validator
UUID Regex Java Validator
Credit Card Regex Java Validator
GUID Regex Java Validator
IP Address Regex Java Validator
Date Regex Java Validator
Email Regex Javascript Validator
Phone Number Regex Javascript Validator
Numbers Regex Javascript Validator
URL Regex Javascript Validator
Password Regex Javascript Validator
SSN Regex Javascript Validator
Mac Address Regex Javascript Validator
UUID Regex Javascript Validator
Credit Card Regex Javascript Validator
GUID Regex Javascript Validator
IP Address Regex Javascript Validator
Date Regex Javascript Validator
Email Regex Python Validator
Phone Number Regex Python Validator
Numbers Regex Python Validator
URL Regex Python Validator
Password Regex Python Validator
SSN Regex Python Validator
Mac Address Regex Python Validator
UUID Regex Python Validator
Credit Card Regex Python Validator
GUID Regex Python Validator
IP Address Regex Python Validator
Date Regex Python Validator
RegEx Tester
Go RegEx Tester
Javascript RegEx Tester
Java RegEx Tester
Email Regex Go Validator
Phone Number Regex Go Validator
Numbers Regex Go Validator
URL Regex Go Validator
Password Regex Validator
SSN Regex Go Validator
Mac Address Regex Go Validator
UUID Regex Go Validator
Credit Card Regex Go Validator
GUID Regex Go Validator
IP Address Regex Go Validator
Date Regex Go Validator
Email Regex Java Validator
Phone Number Regex Java Validator
Numbers Regex Java Validator
URL Regex Java Validator