Email Regex Javascript Validator
Javascript
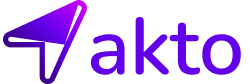
Show Your Support with a Star ⭐
It takes just a second, but it means the world to us.
Introduction to Email Validation with Javascript
JavaScript often requires email validation, especially in web applications, to ensure that user input is correctly formatted. Using regex for email validation in JavaScript is straightforward, efficient, and can be implemented client-side for immediate feedback. A common regex pattern for this purpose is
What is Email Regex JS?
An email address in JavaScript can be validated by breaking it down into three key components.
The Local Part
This part contains alphanumeric and specific special characters.
Pattern:
/^[a-zA-Z0-9._%+-]+/
The Domain
The domain follows the '@' symbol and usually includes alphanumeric characters and possibly hyphens.
Pattern:
/@[a-zA-Z0-9.-]+/
The Top-Level Domain (TLD)
The TLD is typically two or more alphabetic characters.
Pattern:
/\\\\.[a-zA-Z]{2,}$/
Complete Email Regex Pattern
The complete regex pattern in JavaScript is:
How to Validate Email in JavaScript?
To perform email validation in JavaScript:
Define the regex pattern.
Use the
test
method to validate the email string.
Uses of JS Email Regex Validation
Client-Side Validation: Ensuring that users enter valid email addresses in forms before submission.
Data Verification: Validating email addresses in client-side scripts for applications like sign-ups, contact forms, and newsletters.
What next with Email Regex JS?
In JavaScript applications, using regex for email validation ensures that user inputs are properly formatted. Use tools like Akto JavaScript validator to test your email regex.