Java RegEx Tester
Java
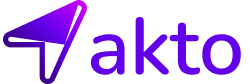
Show Your Support with a Star ⭐
It takes just a second, but it means the world to us.
Introduction Java regex*
Java regular expressions (regex) provide a potent mechanism for pattern matching and text manipulation. Utilizing the java.util.regex
package, Java enables developers to perform complex operations on strings, from validation to parsing and transformation.
Core Constructs of Java Regex Tester
Java regex operates with a variety of constructs, each serving a specific purpose:
Meta characters
.
: Matches any single character, except newline characters.^
: Asserts the start of a line.$
: Asserts the end of a line.|
: Acts as a logical OR operator.
Character Classes
[abc]
: Matches any one of the charactersa
,b
, orc
.[^abc]
: Negates the set; matches any character excepta
,b
, orc
.[a-zA-Z]
: Specifies a range, matching any letter froma
toz
orA
toZ
.
Predefined Character Classes
\\d
: Matches any digit, equivalent to[0-9]
.\\D
: Matches any non-digit.\\s
: Matches any whitespace character.\\S
: Matches any non-whitespace character.\\w
: Matches any word character (alphanumeric & underscore).\\W
: Matches any non-word character.
Quantifiers
``: Zero or more times.
+
: One or more times.?
: Zero or one time; also used to denote a non-greedy quantifier.{n}
: Exactly n times.{n,}
: n or more times.{n,m}
: Between n and m times, inclusive.
Special Constructs
(abc)
: A capturing group that matches the sequenceabc
.(?:abc)
: A non-capturing group.(?i)abc
: An inline flag for case-insensitive matching ofabc
.\\\\b
: A word boundary.\\\\B
: A non-word boundary.
Backreferences
\\\\1
,\\\\2
, ...: Matches the same text as previously matched by a capturing group.
Boundary Matchers
^
: Matches the beginning of a line.$
: Matches the end of a line.\\\\b
: Matches a word boundary.\\\\B
: Matches a non-word boundary.
Flags
(?i)
: Enables case-insensitive matching.(?s)
: Enables dot-all mode, where.
matches any character, including newline characters.
Anchors
\\\\A
: Matches the beginning of the input.\\\\Z
: Matches the end of the input, or before a newline at the end.\\\\z
: Matches the end of the input.
Logical Operators
(?=...)
: Positive lookahead assertion.(?!...)
: Negative lookahead assertion.(?<=...)
: Positive lookbehind assertion.(?<!...)
: Negative lookbehind assertion.
Greedy and Lazy Quantifiers
?
: Zero or more times, as few as possible.+?
: One or more times, as few as possible.??
: Zero or one time, as few as possible.{n}?
: Exactly n times, as few as possible.{n,}?
: n or more times, as few as possible.{n,m}?
: Between n and m times, as few as possible.
Unicode Support
\\\\p{...}
: Matches a Unicode character with the specified property.\\\\P{...}
: Matches any character that does not have the specified property.\\\\p{Is...}
: Matches a character in the specified Unicode block or category.
Java Regular Expressions Examples
Example 1: Java Email Validation
Example 2: Password Strength Check
Example 3: Extracting Words from a String
Practical Tips for Java Regular Expressions
Use the
Pattern
class fromjava.util.regex
to compile and store your regular expression patterns for efficient reuse.When validating email addresses, consider using a pre-built library like Apache Commons Validator or JavaMail instead of crafting your own regex. Email validation can be complex and error-prone.
For complex patterns, break them down into smaller, more manageable parts using capturing groups and non-capturing groups. This improves readability and maintainability.
Test your regular expressions thoroughly using different input scenarios and edge cases to ensure they produce expected results.
Leverage online regex testers, such as regex101 or Akto regex tester, to help you debug and fine-tune your regular expressions.
Be cautious with using regex for parsing HTML or XML. It's generally recommended to use dedicated libraries or parsers for handling structured markup languages.
Regular expressions can be resource-intensive, especially when applied to large input strings or in performance-critical scenarios. Consider optimizing your regex or exploring alternative approaches if performance becomes an issue.
Don't forget to escape special characters when using them in your regular expressions. For example, if you want to match a literal period (
.
), you need to escape it as\\\\.
.Regular expressions are case-sensitive by default. If you want to perform case-insensitive matching, you can use the
(?i)
flag at the beginning of your regex or specify thePattern.CASE_INSENSITIVE
flag when compiling the pattern.
Related Links- Javascript Regex Syntex