Password Regex Validator
Go
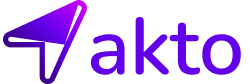
Show Your Support with a Star ⭐
It takes just a second, but it means the world to us.
Introduction to Password Regex.
Regular expressions (regex) are an essential tool for validating user input, especially for sensitive data like passwords. Password regex patterns can enforce rules such as minimum length, inclusion of special characters, and a mix of upper and lower case letters. A typical regex for password validation might be something like ^(?=.*[a-z])(?=.*[A-Z])(?=.*\\d)[a-zA-Z\\d]{8,}$
.
Password Regex Tool
A robust password regex pattern should enforce several rules to enhance security. These might include:
1. Minimum Length
Usually, passwords should have a minimum number of characters for security.
The regex snippet for this might be:
{8,}
This enforces a minimum length of 8 characters.
2. Mixed Character Types
Passwords should include a mix of uppercase and lowercase letters, numbers, and possibly special characters.
A regex pattern for this rule could be a combination of:
Lowercase letters:
(?=.*[a-z])
Uppercase letters:
(?=.*[A-Z])
Numbers:
(?=.*\\d)
Special characters (optional):
(?=.*[@$!%*?&])
The Complete Password Regex Pattern
Combining these requirements, a comprehensive regex pattern for password validation might be:
This pattern enforces at least one lowercase letter, one uppercase letter, and one number, with a minimum length of 8 characters.
How to Validate Passwords Using Regex in Go?
Validating a password using regex in Go involves:
Defining the regex pattern that meets your password policy.
Compiling the regex using the
regexp
package.Validating the password strings with the compiled regex.
Uses of Password Regex Validation
Password regex validation is crucial in areas such as:
User Account Security: Ensuring strong passwords for user accounts to protect against unauthorized access.
Data Protection: In applications handling sensitive data, strong passwords are a first line of defense.
Compliance and Standards: Meeting security standards and compliance requirements often necessitates stringent password policies.
What next?
Regular expressions provide a powerful means to enforce complex password policies effectively. Developers can leverage tools like Akto's Password Validator for testing and ensuring the robustness of their password regex patterns.