RegEx Tester
Python
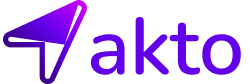
Show Your Support with a Star ⭐
It takes just a second, but it means the world to us.
Introduction RegEx Tester
Python regular expressions (regex) offer a powerful tool for pattern matching and text manipulation, integral to tasks like data validation, parsing, and transformation. Utilizing Python's built-in re
module, developers can execute complex string operations efficiently.
Core Constructs of Python Regex
Python regex employs various constructs, each tailored for specific matching criteria:
Metacharacters
.
: Matches any character except newline.^
: Matches the start of a string.$
: Matches the end of a string or just before the newline at the end.|
: Acts as a logical OR operator.
Character Classes
[abc]
: Matches any one ofa
,b
, orc
.[^abc]
: Negates the set; matches any character excepta
,b
, orc
.[a-zA-Z]
: Matches any alphabet character.
Predefined Character Classes
\\d
: Matches any digit, equivalent to[0-9]
.\\D
: Matches any non-digit.\\s
: Matches any whitespace character.\\S
: Matches any non-whitespace character.\\w
: Matches any word character (alphanumeric plus underscore).\\W
: Matches any non-word character.
Quantifiers
``: Matches 0 or more occurrences.
+
: Matches 1 or more occurrences.?
: Matches 0 or 1 occurrence, making it optional.{n}
: Exactlyn
occurrences.{n,}
: At leastn
occurrences.{n,m}
: Betweenn
andm
occurrences.
Special Constructs
(abc)
: Captures the groupabc
.(?:abc)
: Non-capturing version of regular parentheses.(?=abc)
: Positive lookahead assertion forabc
.(?!abc)
: Negative lookahead assertion forabc
.
Anchors and Boundaries
\\b
: Word boundary.\\B
: Non-word boundary.\\A
: Start of the string.\\Z
: End of the string.
Flags
re.IGNORECASE
orre.I
: Case-insensitive matching.re.MULTILINE
orre.M
: Multi-line mode.re.DOTALL
orre.S
: Makes.
match any character, including newlines.
Python Regular Expressions Examples
Example 1: Email Validation
Example 2: Password Strength Check
Example 3: Extracting Words from a String
Practical Tips for Python Regular Expressions
Use raw strings (
r'...'
) to define regex patterns in Python to avoid issues with backslashes.The
re.compile()
function can be used to compile regex patterns for efficiency, especially if the pattern will be used multiple times.Utilize named groups
(P<name>...)
for better readability and maintainability of complex patterns.Test your regex patterns with various input scenarios, including edge cases, to ensure they behave as expected.
For complex tasks, consider breaking down the pattern into simpler, understandable segments.
Leverage Python's regex methods like
search()
,match()
,findall()
, andfinditer()
as per the use case.Be aware of the performance impact when using regex on large texts or in performance-critical applications.
Remember that regex is not always the best tool for parsing structured or nested data like HTML or JSON; use dedicated parsers when appropriate.
Regular expressions in Python are Unicode-aware, which is important when working with international data.
Utilize online regex testers, such as regex101 or Akto regex tester, to debug and optimize your regex patterns.
By understanding and applying these constructs and tips, developers can effectively harness the power of Python regular expressions. For complex and varied patterns, Akto's regex validator is a valuable resource for testing and ensuring accuracy.
Check our other language Regex Tester - Java Script Regex Tester, Golang Regex Tester, Java Regex Tester