URL Regex Go Validator
Go
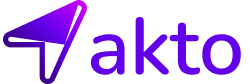
Show Your Support with a Star ⭐
It takes just a second, but it means the world to us.
Introduction
Regular expressions (regex) are immensely useful for validating different types of data, including URLs (Uniform Resource Locators). URLs are ubiquitous in modern software applications, serving as links to websites, resources, and network services. A basic regex pattern for URL validation might look like ^(http|https)://[a-zA-Z0-9.-]+\\.[a-zA-Z]{2,}$
, which checks for a valid web address structure.
URL Regex
URL validation regex needs to consider various components of a URL, such as the protocol, domain, and optional path and query parameters.
1. Protocol
Most URLs begin with a protocol, typically HTTP or HTTPS.
The regex pattern for the protocol part is:
^(http|https)://
This pattern matches URLs starting with either
http://
orhttps://
.
2. Domain
The domain is a critical part of a URL, identifying the website or resource.
The regex for the domain part could be:
[a-zA-Z0-9.-]+\\.[a-zA-Z]{2,}
This ensures the domain consists of valid characters and ends with a top-level domain.
3. Path and Query Parameters (Optional)
URLs might include additional paths and query parameters for specific resources or data.
The regex pattern could extend to include these:
(/[a-zA-Z0-9-._~:?#@!$&'()*+,;=]*)*
This part is optional and matches various characters used in paths and queries.
The Complete URL Regex Pattern
Combining these components, a comprehensive regex pattern for URL validation might be: ^(http|https)://[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}(/[a-zA-Z0-9-._~:?#@!$&'()*+,;=]*)*$
This pattern validates the protocol, domain, and optional paths and parameters.
How to Validate URLs Using Regex in Go?
Validating a URL in Go with regex involves:
Define the regex pattern for a valid URL.
Compile the regex using Go's
regexp
package.Validate the URL strings using the compiled regex.
Uses of URL Regex Validation
URL regex validation is essential in various aspects, such as:
Web Development: Ensuring that links and references to external resources are correctly formatted.
Data Cleaning: In processing datasets, it's important to validate and standardize URLs.
User Input Validation: In forms and user interfaces, preventing incorrect URL formats to ensure usability and functionality.
What next?
Regex provides a robust way to ensure URLs are correctly formatted and valid. Use Akto's URL Validator to test and confirm the efficacy of your URL validation logic, ensuring that your application can accurately handle various types of URLs.